Library for Aitendo 2.6 inch TFT shield for Arduino-UNO and Nucleo
Embed:
(wiki syntax)
Show/hide line numbers
AitendoTFT.h
00001 #ifndef _aitendo_tft_h 00002 #define _aitendo_tft_h 00003 00004 #include "mbed.h" 00005 00006 class AitendoTFT 00007 { 00008 public: 00009 AitendoTFT(); 00010 enum {TFT_WIDTH = 240, TFT_HEIGHT = 320, 00011 BLACK = 0x0000, BLUE = 0x001F, RED = 0xf800, GREEN = 0x07e0, WHITE = 0xffff 00012 }; 00013 void fillArea(int x0, int y0, int x1, int y1, int color); 00014 void fill(int color) { 00015 fillArea(0, 0, TFT_WIDTH, TFT_HEIGHT, color); 00016 }; 00017 void pset(int x, int y, int color); 00018 void setPos(int x, int y) { 00019 _pos_set(x, y); 00020 }; 00021 void put(int color) { 00022 _write(1, color); 00023 } 00024 private: 00025 DigitalOut _rst, _cs, _rs, _wr, _rd; 00026 BusInOut _d; 00027 00028 void _init(void); 00029 void _reset(void); 00030 void _write(int rs, int d); 00031 void _data_write(int data) { 00032 _write(1, data); 00033 }; 00034 void _cmd_write(int cmd) { 00035 _write(0, cmd); 00036 }; 00037 void _reg_write(int addr, int data); 00038 void _pos_set(int x, int y); 00039 }; 00040 00041 #endif /* _aitendo_tft_h */
Generated on Thu Jul 14 2022 07:17:36 by
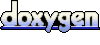