
see http://mbed.org/users/gsfan/code/GSwifi/
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Websocket for GSwifi 00003 * 00004 * mbed WebSocket server / GSwifi client 00005 * html access http://sockets.mbed.org/ws/UserName/test/ 00006 * 00007 * GSwifi WebSocket server / other client 00008 * GSwifi_conf.h: #define GS_USE_WEBSOCKET 00009 * ws access ws://IP address/ws/hoge 00010 */ 00011 00012 #include "mbed.h" 00013 #include "GSwifi.h" 00014 00015 #define PORT 80 00016 00017 #define WS_HOST "sockets.mbed.org" 00018 #define WS_URI "/ws/UserName/test/rw" 00019 00020 #define SECURE GSwifi::GSSEC_WPA_PSK 00021 #define SSID "SSID" 00022 #define PASS "PASSPHRASE" 00023 00024 GSwifi gs(p13, p14, p20); // TX, RX, Reset (no flow control) 00025 //GSwifi gs(p13, p14, p12, P0_22, p20, NC, 115200); // TX, RX, CTS, RTS, Reset, Alarm 00026 00027 LocalFileSystem local("local"); 00028 Serial pc(USBTX, USBRX); 00029 DigitalOut led1(LED1), led2(LED2); 00030 00031 void ws_server (int cid, GSwifi::GS_httpd *gshttpd) { 00032 00033 pc.printf("WS_S %d: %s ? %s '%s' %d\r\n", cid, gshttpd->file, gshttpd->query, gshttpd->buf, gshttpd->len); 00034 00035 gs.wsSend(cid, gshttpd->buf, gshttpd->len); 00036 } 00037 00038 void ws_client (int cid, int len) { 00039 int n; 00040 char buf[len + 1]; 00041 00042 n = gs.recv(cid, buf, sizeof(buf)); 00043 buf[n] = 0; 00044 pc.printf("WS_C %d: %s %d\r\n", cid, buf, n); 00045 } 00046 00047 int main () { 00048 IpAddr ipaddr, netmask, gateway, nameserver; 00049 Host host; 00050 int cid; 00051 00052 led1 = 1; 00053 pc.baud(115200); 00054 00055 pc.printf("connecting...\r\n"); 00056 if (gs.connect(SECURE, SSID, PASS)) { 00057 return -1; 00058 } 00059 gs.getAddress(ipaddr, netmask, gateway, nameserver); 00060 pc.printf("ip %d.%d.%d.%d\r\n", ipaddr[0], ipaddr[1], ipaddr[2], ipaddr[3]); 00061 00062 led2 = 1; 00063 pc.printf("httpd\r\n"); 00064 gs.httpd(PORT); 00065 gs.attach_httpd("/ws/", &ws_server); 00066 gs.attach_httpd("/", "/local/"); 00067 00068 host.setName(WS_HOST); 00069 host.setPort(PORT); 00070 cid = gs.wsOpen(host, WS_URI, &ws_client); 00071 if (cid < 0) return -1; 00072 printf("ws open\r\n"); 00073 00074 for (;;) { 00075 gs.poll(); 00076 00077 if (pc.readable()) { 00078 char buf[] = "MBED= "; 00079 buf[5] = pc.getc(); 00080 if (gs.wsSend(cid, buf, 6, "MASK")) { 00081 printf("ws error\r\n"); 00082 } 00083 } 00084 00085 wait_ms(50); 00086 led1 = !led1; 00087 led2 = 0; 00088 } 00089 }
Generated on Mon Jul 25 2022 02:32:43 by
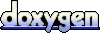