
UDP echo server for GSwifi see: http://mbed.org/users/gsfan/notebook/gainspan_wifi/
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "GSwifi.h" 00003 00004 #define PORT 10080 00005 00006 #define SECURE GSwifi::GSSEC_WPA_PSK 00007 #define SSID "SSID" 00008 #define PASS "PASSPHRASE" 00009 00010 GSwifi gs(p13, p14, p20); // TX, RX, Reset (no flow control) 00011 //GSwifi gs(p13, p14, p12, P0_22, p20, NC, 115200); // TX, RX, CTS, RTS, Reset, Alarm 00012 00013 Serial pc(USBTX, USBRX); 00014 DigitalOut led1(LED1), led2(LED2); 00015 00016 void onGsReceive (int cid, int len) { 00017 int i; 00018 char buf[100]; 00019 Host host; 00020 00021 led2 = 1; 00022 00023 i = gs.recv(cid, buf, sizeof(buf), host); 00024 gs.send(cid, buf, i, host); 00025 pc.printf("recv %d\r\n", i); 00026 } 00027 00028 int main () { 00029 IpAddr ipaddr, netmask, gateway, nameserver; 00030 00031 led1 = 1; 00032 pc.baud(115200); 00033 00034 pc.printf("connect\r\n"); 00035 if (gs.connect(SECURE, SSID, PASS)) { 00036 return -1; 00037 } 00038 gs.getAddress(ipaddr, netmask, gateway, nameserver); 00039 pc.printf("ip %d.%d.%d.%d\r\n", ipaddr[0], ipaddr[1], ipaddr[2], ipaddr[3]); 00040 00041 pc.printf("listen\r\n"); 00042 gs.listen(PORT, GSwifi::GSPROT_UDP, &onGsReceive); 00043 00044 for (;;) { 00045 gs.poll(); 00046 00047 led1 = !led1; 00048 wait_ms(50); 00049 } 00050 }
Generated on Fri Jul 15 2022 02:16:33 by
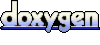