
Cosm (Pachube) feed see: http://mbed.org/users/gsfan/notebook/gainspan_wifi/
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "GSwifi.h" 00003 00004 #define SECURE GSwifi::GSSEC_WPA_PSK 00005 #define SSID "SSID" 00006 #define PASS "passkey" 00007 00008 #define FEED_HOST "api.cosm.com" 00009 #define FEED_ID "000000" 00010 #define API_KEY "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" 00011 00012 GSwifi gs(p13, p14, p20); // TX, RX, Reset (no flow control) 00013 //GSwifi gs(p13, p14, p12, P0_22, p20, NC, 115200); // TX, RX, CTS, RTS, Reset, Alarm 00014 Serial pc(USBTX, USBRX); 00015 DigitalOut led1(LED1), led2(LED2); 00016 00017 void onGsReceive (int cid, int len) { 00018 int i; 00019 char buf[GS_DATA_SIZE + 1]; 00020 00021 led2 = 1; 00022 i = gs.recv(cid, buf, len); 00023 buf[i] = 0; 00024 pc.printf(buf); 00025 } 00026 00027 int cosm (char *data) { 00028 int i; 00029 Host host; 00030 int cid; 00031 char buf[100]; 00032 00033 host.setName(FEED_HOST); 00034 host.setPort(80); 00035 cid = gs.open(host, GSwifi::GSPROT_TCP, &onGsReceive); 00036 if (cid < 0) return -1; 00037 00038 sprintf(buf, "PUT /v2/feeds/" FEED_ID ".csv HTTP/1.1\r\n"); 00039 gs.send(cid, buf, strlen(buf)); 00040 sprintf(buf, "Host: " FEED_HOST "\r\n"); 00041 gs.send(cid, buf, strlen(buf)); 00042 sprintf(buf, "Connection: close\r\n"); 00043 gs.send(cid, buf, strlen(buf)); 00044 sprintf(buf, "X-ApiKey: " API_KEY "\r\n"); 00045 gs.send(cid, buf, strlen(buf)); 00046 sprintf(buf, "Content-Type: text/csv\r\n"); 00047 gs.send(cid, buf, strlen(buf)); 00048 sprintf(buf, "Content-Length: %d\r\n", strlen(data)); 00049 gs.send(cid, buf, strlen(buf)); 00050 gs.send(cid, "\r\n", 2); 00051 gs.send(cid, data, strlen(data)); 00052 00053 for (i = 0; i < 10; i ++) { 00054 if (! gs.isConnected(cid)) break; 00055 wait(100); 00056 } 00057 gs.close(cid); 00058 return 0; 00059 } 00060 00061 int main () { 00062 IpAddr ipaddr, netmask, gateway, nameserver; 00063 char data[] = "1,123\r\n2,345\r\n"; 00064 00065 led1 = 1; 00066 pc.baud(115200); 00067 00068 pc.printf("connect\r\n"); 00069 if (gs.connect(SECURE, SSID, PASS, 0)) { 00070 return -1; 00071 } 00072 gs.getAddress(ipaddr, netmask, gateway, nameserver); 00073 pc.printf("ip %d.%d.%d.%d\r\n", ipaddr[0], ipaddr[1], ipaddr[2], ipaddr[3]); 00074 00075 pc.printf("Cosm\r\n"); 00076 cosm(data); 00077 00078 pc.printf("exit\r\n"); 00079 }
Generated on Thu Jul 21 2022 20:16:17 by
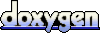