
see: http://mbed.org/users/gsfan/notebook/GSwifiInterface/
Dependencies: GSwifiInterface mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "GSwifiInterface.h" 00003 00004 #define SEC GSwifi::SEC_WPA_PSK 00005 #define SSID "SSID" 00006 #define PASS "PASSPHRASE" 00007 00008 #define ECHO_SERVER_ADDRESS "192.168.1.101" 00009 #define ECHO_SERVER_PORT 10000 00010 00011 int main () { 00012 GSwifiInterface gs(p13, p14, p12, P0_22, p20, NC); 00013 printf("UDP Echo Client...\n"); 00014 gs.init(); //Use DHCP 00015 if (gs.connect(SEC, SSID, PASS)) return -1; // join the network 00016 printf("IP Address is %s\n", gs.getIPAddress()); 00017 00018 UDPSocket sock; 00019 sock.init(); 00020 00021 Endpoint echo_server; 00022 echo_server.set_address(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT); 00023 00024 char out_buffer[] = "Hello World\n"; 00025 sock.sendTo(echo_server, out_buffer, sizeof(out_buffer)); 00026 00027 char in_buffer[256]; 00028 int n = sock.receiveFrom(echo_server, in_buffer, sizeof(in_buffer)); 00029 00030 in_buffer[n] = '\0'; 00031 printf("%s\n", in_buffer); 00032 00033 sock.close(); 00034 00035 gs.disconnect(); 00036 while(1) {} 00037 }
Generated on Thu Jul 14 2022 12:13:04 by
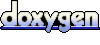