GSwifiInterface library (interface for GainSpan Wi-Fi GS1011 modules) Please see https://mbed.org/users/gsfan/notebook/GSwifiInterface/
Dependents: GSwifiInterface_HelloWorld GSwifiInterface_HelloServo GSwifiInterface_UDPEchoServer GSwifiInterface_UDPEchoClient ... more
Fork of WiflyInterface by
GSwifi_smtp.cpp
00001 /* Copyright (C) 2013 gsfan, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "GSwifi.h" 00020 00021 #ifdef CFG_ENABLE_SMTP 00022 00023 int GSwifi::mail (const char *host, int port, const char *to, const char *from, const char *subject, const char *mesg, const char *user, const char *pwd) { 00024 int ret = -1; 00025 int cid; 00026 char cmd[CFG_DATA_SIZE]; 00027 char ip[17]; 00028 00029 if (!isAssociated() || _state.status != STAT_READY) return -1; 00030 00031 if (getHostByName(host, ip)) return -1; 00032 if (! port) { 00033 port = 25; 00034 } 00035 00036 cid = open(PROTO_TCP, ip, port); 00037 if (cid < 0) return -1; 00038 DBG("cid %d\r\n", cid); 00039 00040 if (smtpWait(cid ,220)) goto exit; 00041 00042 // send request 00043 snprintf(cmd, sizeof(cmd), "EHLO %s\r\n", _state.name); 00044 send(cid, cmd, strlen(cmd)); 00045 wait_ms(100); 00046 if (smtpWait(cid ,250)) goto quit; 00047 smtpWait(cid ,0); 00048 00049 if (user && pwd) { 00050 // smtp auth 00051 int len; 00052 snprintf(cmd, sizeof(cmd), "%s%c%s%c%s", user, 0, user, 0, pwd); 00053 len = strlen(user) * 2 + strlen(pwd) + 2; 00054 char tmp[len + (len / 2)]; 00055 base64encode(cmd, len, tmp, sizeof(tmp)); 00056 snprintf(cmd, sizeof(cmd), "AUTH PLAIN %s\r\n", tmp); 00057 send(cid, cmd, strlen(cmd)); 00058 if (smtpWait(cid ,235)) goto quit; 00059 } 00060 00061 snprintf(cmd, sizeof(cmd), "MAIL FROM: %s\r\n", from); 00062 send(cid, cmd, strlen(cmd)); 00063 if (smtpWait(cid ,250)) goto quit; 00064 00065 snprintf(cmd, sizeof(cmd), "RCPT TO: %s\r\n", to); 00066 send(cid, cmd, strlen(cmd)); 00067 if (smtpWait(cid ,250)) goto quit; 00068 00069 strcpy(cmd, "DATA\r\n"); 00070 send(cid, cmd, strlen(cmd)); 00071 if (smtpWait(cid ,354)) goto quit; 00072 00073 // mail data 00074 snprintf(cmd, sizeof(cmd), "From: %s\r\n", from); 00075 send(cid, cmd, strlen(cmd)); 00076 snprintf(cmd, sizeof(cmd), "To: %s\r\n", to); 00077 send(cid, cmd, strlen(cmd)); 00078 snprintf(cmd, sizeof(cmd), "Subject: %s\r\n\r\n", subject); 00079 send(cid, cmd, strlen(cmd)); 00080 00081 send(cid, mesg, strlen(mesg)); 00082 strcpy(cmd, "\r\n.\r\n"); 00083 send(cid, cmd, strlen(cmd)); 00084 if (smtpWait(cid ,250)) goto quit; 00085 ret = 0; 00086 00087 INFO("Mail, from: %s, to: %s %d\r\n", from, to, strlen(mesg)); 00088 00089 quit: 00090 strcpy(cmd, "QUIT\r\n"); 00091 send(cid, cmd, strlen(cmd)); 00092 smtpWait(cid ,221); 00093 exit: 00094 close(cid); 00095 return ret; 00096 } 00097 00098 int GSwifi::smtpWait (int cid, int code) { 00099 Timer timeout; 00100 int i, n, len = 0; 00101 char buf[CFG_CMD_SIZE], data[CFG_CMD_SIZE]; 00102 00103 if (code == 0) { 00104 // dummy read 00105 timeout.start(); 00106 while (timeout.read_ms() < CFG_TIMEOUT) { 00107 wait_ms(10); 00108 if (!readable(cid)) break; 00109 n = recv(cid, buf, sizeof(buf)); 00110 if (n <= 0) break; 00111 } 00112 timeout.stop(); 00113 return 0; 00114 } 00115 00116 // wait responce 00117 len = 0; 00118 timeout.start(); 00119 while (timeout.read_ms() < CFG_TIMEOUT) { 00120 wait_ms(10); 00121 n = recv(cid, buf, sizeof(buf)); 00122 for (i = 0; i < n; i ++) { 00123 if (buf[i] == '\r') continue; 00124 if (buf[i] == '\n') { 00125 if (len == 0) continue; 00126 goto next; 00127 } else 00128 if (len < sizeof(data) - 1) { 00129 data[len] = buf[i]; 00130 len ++; 00131 } 00132 } 00133 } 00134 next: 00135 data[len] = 0; 00136 DBG("smtp: %s\r\n", data); 00137 timeout.stop(); 00138 00139 // check return code 00140 i = atoi(data); 00141 DBG("smtp status %d\r\n", i); 00142 return i == code ? 0 : -1; 00143 } 00144 00145 #endif
Generated on Thu Jul 14 2022 07:53:37 by
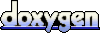