GainSpan Wi-Fi library see: http://mbed.org/users/gsfan/notebook/gainspan_wifi/
Dependents: GSwifi_httpd GSwifi_websocket GSwifi_tcpclient GSwifi_tcpserver ... more
Fork of GSwifi by
GSwifi_smtp.cpp
00001 /* Copyright (C) 2013 gsfan, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 /** @file 00019 * @brief Gainspan wi-fi module library for mbed 00020 * GS1011MIC, GS1011MIP, GainSpan WiFi Breakout, etc. 00021 */ 00022 00023 #include "GSwifi_conf.h" 00024 #ifdef GS_ENABLE_SMTP 00025 00026 #include "dbg.h" 00027 #include "mbed.h" 00028 #include "GSwifi.h" 00029 00030 int GSwifi::mail (Host &host, const char *to, const char *from, const char *subject, const char *mesg, const char *user, const char *pwd) { 00031 int ret = -1; 00032 int cid; 00033 00034 if (! _connect || _status != GSSTAT_READY) return -1; 00035 00036 if (host.getIp().isNull()) { 00037 if (getHostByName(host)) { 00038 if (getHostByName(host)) return -1; 00039 } 00040 } 00041 if (host.getPort() == 0) { 00042 host.setPort(25); 00043 } 00044 00045 cid = open(host, GSPROT_TCP); 00046 if (cid < 0) return -1; 00047 DBG("cid %d\r\n", cid); 00048 00049 if (wait_smtp(cid ,220)) goto exit; 00050 00051 // send request 00052 send(cid, "EHLO gainspan\r\n", 15); 00053 wait_ms(100); 00054 if (wait_smtp(cid ,250)) goto exit; 00055 wait_smtp(cid ,0); 00056 00057 if (user && pwd) { 00058 // smtp auth 00059 char tmp[80], buf[100]; 00060 int len; 00061 snprintf(tmp, sizeof(tmp), "%s%c%s%c%s", user, 0, user, 0, pwd); 00062 len = strlen(user) * 2 + strlen(pwd) + 2; 00063 base64encode(tmp, len, buf, sizeof(buf)); 00064 send(cid, "AUTH PLAIN ", 11); 00065 send(cid, buf, strlen(buf)); 00066 send(cid, "\r\n", 2); 00067 if (wait_smtp(cid ,235)) goto quit; 00068 } 00069 00070 send(cid, "MAIL FROM: ", 11); 00071 send(cid, from, strlen(from)); 00072 send(cid, "\r\n", 2); 00073 if (wait_smtp(cid ,250)) goto quit; 00074 00075 send(cid, "RCPT TO: ", 9); 00076 send(cid, to, strlen(to)); 00077 send(cid, "\r\n", 2); 00078 if (wait_smtp(cid ,250)) goto quit; 00079 00080 send(cid, "DATA\r\n", 6); 00081 if (wait_smtp(cid ,354)) goto quit; 00082 00083 // mail data 00084 send(cid, "From: ", 6); 00085 send(cid, from, strlen(from)); 00086 send(cid, "\r\n", 2); 00087 send(cid, "To: ", 9); 00088 send(cid, to, strlen(to)); 00089 send(cid, "\r\n", 2); 00090 send(cid, "Subject: ", 9); 00091 send(cid, subject, strlen(subject)); 00092 send(cid, "\r\n\r\n", 4); 00093 00094 send(cid, mesg, strlen(mesg)); 00095 send(cid, "\r\n.\r\n", 5); 00096 if (wait_smtp(cid ,250)) goto quit; 00097 ret = 0; 00098 00099 LOG("Mail, from: %s, to: %s %d\r\n", from, to, strlen(mesg)); 00100 00101 quit: 00102 send(cid, "QUIT\r\n", 6); 00103 if (wait_smtp(cid ,221)) goto exit; 00104 00105 exit: 00106 close(cid); 00107 return ret; 00108 } 00109 00110 int GSwifi::wait_smtp (int cid, int code) { 00111 Timer timeout; 00112 int i, n, len = 0; 00113 char buf[200], data[100]; 00114 00115 if (code == 0) { 00116 // dummy read 00117 timeout.start(); 00118 while (timeout.read_ms() < GS_TIMEOUT) { 00119 wait_ms(10); 00120 if (_gs_sock[cid].data->isEmpty()) break; 00121 poll(); 00122 n = recv(cid, buf, sizeof(buf)); 00123 if (n <= 0) break; 00124 } 00125 timeout.stop(); 00126 return 0; 00127 } 00128 00129 // wait responce 00130 len = 0; 00131 timeout.start(); 00132 while (timeout.read_ms() < SMTP_TIMEOUT) { 00133 wait_ms(10); 00134 poll(); 00135 n = recv(cid, buf, sizeof(buf)); 00136 for (i = 0; i < n; i ++) { 00137 if (buf[i] == '\r') continue; 00138 if (buf[i] == '\n') { 00139 if (len == 0) continue; 00140 goto next; 00141 } else 00142 if (len < sizeof(data) - 1) { 00143 data[len] = buf[i]; 00144 len ++; 00145 } 00146 } 00147 } 00148 next: 00149 data[len] = 0; 00150 DBG("smtp: %s\r\n", data); 00151 timeout.stop(); 00152 00153 // check return code 00154 i = atoi(data); 00155 DBG("smtp status %d\r\n", i); 00156 if (i == code) return 0; 00157 00158 return -1; 00159 } 00160 00161 #endif
Generated on Tue Jul 12 2022 22:02:57 by
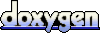