OLD
Embed:
(wiki syntax)
Show/hide line numbers
GSwifi.h
Go to the documentation of this file.
00001 /** 00002 * Gainspan wi-fi module library for mbed 00003 * Copyright (c) 2012 gsfan 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 /** @file 00008 * @brief Gainspan wi-fi module library for mbed 00009 * GS1011MIC, GS1011MIP, GainSpan WiFi Breakout, etc. 00010 * module configuration: ATB=115200 00011 */ 00012 00013 #ifndef _GSWIFI_H_ 00014 #define _GSWIFI_H_ 00015 00016 #include "mbed.h" 00017 #include "GSwifi_net.h" 00018 #include "RingBuffer.h" 00019 #include "host.h" 00020 #include "ipaddr.h" 00021 00022 #define GS_BAUD 115200 00023 #define GS_UART_DIRECT 00024 00025 #define GS_BULK 00026 00027 #define GS_TIMEOUT 10000 // ms 00028 #define GS_TIMEOUT2 30000 // ms 00029 #define GS_CMD_SIZE 100 00030 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00031 //#define GS_DATA_SIZE 1500 00032 #define GS_DATA_SIZE 1000 00033 #elif defined(TARGET_LPC11U24) 00034 #define GS_DATA_SIZE 500 00035 #endif 00036 00037 /** 00038 * Wi-Fi security 00039 */ 00040 enum GSSECURITY { 00041 GSSEC_AUTO = 0, 00042 GSSEC_NONE = 0, 00043 GSSEC_OPEN = 1, 00044 GSSEC_WEP = 2, 00045 GSSEC_WPA_PSK = 4, 00046 GSSEC_WPA2_PSK = 8, 00047 GSSEC_WPA_ENT = 16, 00048 GSSEC_WPA2_ENT = 32, 00049 GSSEC_WPS_BUTTON = 64, 00050 }; 00051 00052 /** 00053 * TCP/IP protocol 00054 */ 00055 enum GSPROTOCOL { 00056 GSPROT_UDP = 0, 00057 GSPROT_TCP = 1, 00058 GSPROT_HTTPGET, 00059 GSPROT_HTTPPOST, 00060 GSPROT_HTTPD, 00061 }; 00062 00063 /** 00064 * Client/Server 00065 */ 00066 enum GSTYPE { 00067 GSTYPE_CLIENT = 0, 00068 GSTYPE_SERVER = 1, 00069 }; 00070 00071 enum GSRESPONCE { 00072 GSRES_NORMAL, 00073 GSRES_CONNECT, 00074 GSRES_WPS, 00075 GSRES_MACADDRESS, 00076 GSRES_DHCP, 00077 GSRES_DNSLOOKUP, 00078 GSRES_HTTP, 00079 GSRES_RSSI, 00080 GSRES_TIME, 00081 }; 00082 00083 enum GSMODE { 00084 GSMODE_COMMAND, 00085 GSMODE_DATA_RX, 00086 GSMODE_DATA_RXUDP, 00087 GSMODE_DATA_RX_BULK, 00088 GSMODE_DATA_RXUDP_BULK, 00089 GSMODE_DATA_RXHTTP, 00090 }; 00091 00092 enum GSSTATUS { 00093 GSSTAT_READY, 00094 GSSTAT_STANDBY, 00095 GSSTAT_WAKEUP, 00096 GSSTAT_DEEPSLEEP, 00097 }; 00098 00099 /** 00100 * data receive callback function 00101 */ 00102 typedef void (*onGsReceiveFunc)(int cid, int len); 00103 00104 struct GS_Socket { 00105 GSTYPE type; 00106 GSPROTOCOL protocol; 00107 bool connect; 00108 Host host; 00109 RingBuffer *data; 00110 int lcid; 00111 int received; 00112 onGsReceiveFunc onGsReceive; 00113 }; 00114 00115 /** 00116 * GSwifi class 00117 */ 00118 class GSwifi { 00119 public: 00120 /** 00121 * default constructor 00122 */ 00123 GSwifi (PinName p_tx, PinName p_rx, int baud = GS_BAUD); 00124 /** 00125 * Default constructor (with hardware fllow controll) 00126 */ 00127 GSwifi (PinName p_tx, PinName p_rx, PinName p_cts, PinName p_rts, int baud = GS_BAUD); 00128 /** 00129 * send command 00130 */ 00131 int command (const char *cmd, GSRESPONCE res, int timeout = GS_TIMEOUT); 00132 /** 00133 * recv responce 00134 */ 00135 int cmdResponse (GSRESPONCE res, int ms); 00136 /** 00137 * associate infrastructure 00138 * @param sec GSSEC_OPEN, GSSEC_WEP, GSSEC_WPA_PSK, GSSEC_WPA2_PSK, GSSEC_WPS_BUTTON 00139 * @param ssid SSID 00140 * @param pass pass phrase 00141 * @param dhcp 0:static ip, 1:dhcp 00142 * @retval 0 success 00143 * @retval -1 failure 00144 */ 00145 int connect (GSSECURITY sec, const char *ssid, const char *pass, int dhcp = 1); 00146 /** 00147 * adhock 00148 * @param sec GSSEC_OPEN or GSSEC_WEP 00149 * @param ssid SSID 00150 * @param pass 10 or 26 hexadecimal digits 00151 * @param ipaddr my ip address 00152 * @param netmask subnet mask 00153 * @retval 0 success 00154 * @retval -1 failure 00155 */ 00156 int adhock (GSSECURITY sec, const char *ssid, const char *pass, IpAddr ipaddr, IpAddr netmask); 00157 /** 00158 * limited AP 00159 * @param sec GSSEC_OPEN or GSSEC_WEP 00160 * @param ssid SSID 00161 * @param pass 10 or 26 hexadecimal digits 00162 * @param ipaddr my ip address (dhcp start address) 00163 * @param netmask subnet mask 00164 * @retval 0 success 00165 * @retval -1 failure 00166 * firmware: s2w-secureweb, s2w-web, s2w-wpsweb 00167 */ 00168 int limitedap (GSSECURITY sec, const char *ssid, const char *pass, IpAddr ipaddr, IpAddr netmask, bool prov = false); 00169 /** 00170 * unassociate 00171 */ 00172 int disconnect (); 00173 00174 /** 00175 * use DHCP 00176 */ 00177 int setAddress (); 00178 /** 00179 * use static ip address 00180 */ 00181 int setAddress (IpAddr ipaddr, IpAddr netmask, IpAddr gateway, IpAddr nameserver); 00182 /** 00183 * get ip address 00184 */ 00185 int getAddress (IpAddr &ipaddr, IpAddr &netmask, IpAddr &gateway, IpAddr &nameserver); 00186 /** 00187 * resolv hostname 00188 * @param name hostname 00189 * @param addr resolved ip address 00190 */ 00191 int getHostByName (const char* name, IpAddr &addr); 00192 /** 00193 * resolv hostname 00194 * @param host.name hostname 00195 * @param host.ipaddr resolved ip address 00196 */ 00197 int getHostByName (Host &host); 00198 /** 00199 * RF power 00200 * @param power 0(high)-7(low) 00201 */ 00202 int setRFPower (int power); 00203 /** 00204 * power save mode 00205 * @param active rx radio 0:switched off, 1:always on 00206 * @param save power save 0:disable, 1:enable 00207 */ 00208 int powerSave (int active, int save); 00209 /** 00210 * standby mode 00211 * @param msec wakeup after 00212 * wakeup after msec or alarm1/2 00213 * core off, save to RTC ram 00214 */ 00215 int standby (int msec); 00216 /** 00217 * restore standby 00218 */ 00219 int wakeup (); 00220 /** 00221 * deep sleep mode 00222 */ 00223 int deepSleep (); 00224 /** 00225 * wifi connected 00226 */ 00227 bool isConnected (); 00228 /** 00229 * status 00230 * @return GSSTATUS 00231 */ 00232 GSSTATUS getStatus (); 00233 /** 00234 * RSSI 00235 * @return RSSI (dBm) 00236 */ 00237 int getRssi (); 00238 /** 00239 * set NTP server 00240 * @param host SNTP server 00241 * @param sec time sync interval, 0:one time 00242 */ 00243 int ntpdate (Host host, int sec = 0); 00244 /** 00245 * set system time 00246 * @param time date time (UTC) 00247 */ 00248 int setTime (time_t time); 00249 /** 00250 * get RTC time 00251 * @return date time (UTC) 00252 */ 00253 time_t getTime (); 00254 /** 00255 * GPIO output 00256 * @param port 10,11,30,31 00257 * @param out 0:set(high), 1:reset(low) 00258 */ 00259 int gpioOut (int port, int out); 00260 00261 /** 00262 * main polling 00263 */ 00264 void poll(); 00265 00266 /** 00267 * tcp/udp client 00268 * @return CID, -1:failure 00269 */ 00270 int open (Host &host, GSPROTOCOL pro, onGsReceiveFunc ponGsReceive = NULL); 00271 /** 00272 * tcp/udp server 00273 * @return CID, -1:failure 00274 */ 00275 int listen (int port, GSPROTOCOL pro, onGsReceiveFunc ponGsReceive = NULL); 00276 /* 00277 template<typename T> 00278 int listen2 (T* tptr, int port, GSPROTOCOL pro, void (T::*mptr)(int,int)) { 00279 if((mptr != NULL) && (tptr != NULL)) { 00280 return listen(port, pro, reinterpret_cast<onGsReceiveFunc>(mptr)); 00281 } 00282 } 00283 */ 00284 /** 00285 * close client/server 00286 */ 00287 int close (int cid); 00288 /** 00289 * send data tcp(s/c), udp(c) 00290 */ 00291 int send (int cid, const char *buf, int len); 00292 /** 00293 * send data udp(s) 00294 */ 00295 int send (int cid, const char *buf, int len, Host &host); 00296 /** 00297 * recv data tcp(s/c), udp(c) 00298 * @return length 00299 */ 00300 int recv (int cid, char *buf, int len); 00301 /** 00302 * recv data udp(s) 00303 * @return length 00304 */ 00305 int recv (int cid, char *buf, int len, Host &host); 00306 /** 00307 * tcp/udp connected 00308 */ 00309 bool isConnected (int cid); 00310 00311 /** 00312 * http request (GET method) 00313 */ 00314 int httpGet (Host &host, const char *uri, const char *user, const char *pwd, int ssl = 0, onGsReceiveFunc ponGsReceive = NULL); 00315 int httpGet (Host &host, const char *uri, int ssl = 0, onGsReceiveFunc ponGsReceive = NULL); 00316 /** 00317 * http request (POST method) 00318 */ 00319 int httpPost (Host &host, const char *uri, const char *body, const char *user, const char *pwd, int ssl = 0, onGsReceiveFunc ponGsReceive = NULL); 00320 int httpPost (Host &host, const char *uri, const char *body, int ssl = 0, onGsReceiveFunc ponGsReceive = NULL); 00321 00322 /** 00323 * certificate 00324 */ 00325 int certAdd (const char *name, const char *cert, int len); 00326 00327 #ifdef GS_USE_SMTP 00328 /** 00329 * send mail (smtp) 00330 * @param host SMTP server 00331 * @param to To address 00332 * @param from From address 00333 * @param subject Subject 00334 * @param mesg Message 00335 * @param user username (SMTP Auth) 00336 * @param pwd password (SMTP Auth) 00337 * @retval 0 success 00338 * @retval -1 failure 00339 */ 00340 int mail (Host &host, const char *to, const char *from, const char *subject, const char *mesg, const char *user = NULL, const char *pwd = NULL); 00341 #endif 00342 00343 #ifdef GS_USE_HTTPD 00344 /** 00345 * start http server 00346 * @param port 00347 */ 00348 int httpd (int port = 80); 00349 /** 00350 * attach uri to dirctory handler 00351 */ 00352 void send_httpd_error (int cid, int err); 00353 /** 00354 * attach uri to dirctory handler 00355 */ 00356 int attach_httpd (const char *uri, const char *dir); 00357 /** 00358 * attach uri to cgi handler 00359 */ 00360 int attach_httpd (const char *uri, onHttpdCgiFunc ponHttpCgi); 00361 #endif 00362 00363 /** 00364 * base64 encode 00365 */ 00366 int base64encode (char *input, int length, char *output, int len); 00367 /** 00368 * url encode 00369 */ 00370 int urlencode (char *str, char *buf, int len); 00371 /** 00372 * url decode 00373 */ 00374 int urldecode (char *str, char *buf, int len); 00375 00376 #ifdef DEBUF 00377 void test (); 00378 int getc(); 00379 void putc(char c); 00380 int readable(); 00381 #endif 00382 00383 protected: 00384 void poll_cmd (); 00385 int x2i (char c); 00386 char i2x (int i); 00387 void isr_recv (); 00388 void newSock (int cid, GSTYPE type, GSPROTOCOL pro, onGsReceiveFunc ponGsReceive); 00389 int from_hex (int ch); 00390 int to_hex (int code); 00391 00392 #ifdef GS_USE_SMTP 00393 int wait_smtp (int cid, int code); 00394 #endif 00395 00396 #ifdef GS_USE_HTTPD 00397 int httpd_request (int cid, GS_httpd *gshttpd, char *dir); 00398 char *mimetype (char *file); 00399 #endif 00400 00401 private: 00402 Serial _gs; 00403 bool _rts; 00404 volatile bool _connect; 00405 volatile GSSTATUS _status; 00406 volatile int _gs_ok, _gs_failure, _gs_enter; 00407 volatile int _response; 00408 GSMODE _gs_mode; 00409 int _escape; 00410 int _cid, _rssi; 00411 IpAddr _ipaddr, _netmask, _gateway, _nameserver, _resolv; 00412 Host _from, _to; 00413 char _mac[6]; 00414 RingBuffer _buf_cmd; 00415 struct GS_Socket _gs_sock[16]; 00416 time_t _time; 00417 00418 #ifdef GS_USE_HTTPD 00419 struct GS_httpd _httpd[16]; 00420 struct GS_httpd_handler _handler[10]; 00421 int _handler_count; 00422 00423 void poll_httpd (int cid, int len); 00424 #endif 00425 }; 00426 00427 #endif
Generated on Fri Jul 15 2022 01:47:52 by
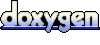