Library for my home monitoring classes and serial communication protocol. It monitors temperature and movement on the mbed application board.
Motion.h
00001 #ifndef MOTION_H 00002 #define MOTION_H 00003 00004 #include <vector> 00005 #include <string> 00006 #include "HomeMonUtils.h" 00007 00008 /** Simple structure to hold a motion vector - x, y, and z motion 00009 */ 00010 struct motion_vec { 00011 double x; 00012 double y; 00013 double z; 00014 }; 00015 00016 /** Motion class stores historical motion alert data and thresholding for alert generation 00017 */ 00018 class Motion { 00019 private: 00020 motion_vec min_motion; 00021 int max_samples_limit; 00022 int max_samples; 00023 int sample_ptr; 00024 bool wrapped_once; 00025 std::vector<std::string> motion_samples; 00026 00027 public: 00028 /** 00029 * Instantiate a motion class. Initialization hard-coded to 00030 * known good values. Use member functions to modify. 00031 */ 00032 Motion(); 00033 /** 00034 * Allows host to retrieve motion threshold as a vector. 00035 * 00036 * @param return - Returns a motion vector structure with x, y, and z threholds for motion. 00037 */ 00038 motion_vec get_motion_thresh(void); 00039 /** 00040 * Allows host to set the motion threshold 00041 * 00042 * @param motion_thresh - Motion vector threshold setting 00043 * @param return - pass/faill for set 00044 */ 00045 bool set_motion_thresh(motion_vec motion_thresh); 00046 /** 00047 * Adds a sample to the motion database. Units are time in seconds since device booted. 00048 * 00049 * @param motion_sample - represents seconds since device booted that a motion alert occurred. 00050 */ 00051 void add_sample(double motion_sample); 00052 /** 00053 * Returns all motion samples in the motion database. 00054 * 00055 * @param return - vector containing strings for all motion alerts (seconds since device booted) 00056 */ 00057 const std::vector<std::string> &get_samples(); 00058 /** 00059 * Allows the host to change the motion database size. 00060 * WARNING: This may cause sample to be lost due to vector resize operation 00061 * 00062 * @param num_samples - new size of motion alert DB (max allowed currently 100) 00063 * @param return - pass/fail of operation 00064 */ 00065 bool change_max_samples(int num_samples); 00066 /** 00067 * Returns current database size 00068 * 00069 * @param return - size in samples of motion alert DB 00070 */ 00071 int get_max_samples(); 00072 00073 }; 00074 00075 #endif
Generated on Fri Jul 22 2022 02:01:25 by
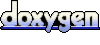