Library for my home monitoring classes and serial communication protocol. It monitors temperature and movement on the mbed application board.
Motion.cpp
00001 // 00002 // This file contains function related to the motion 00003 // control of the home monitoring system 00004 // 00005 00006 #include <vector> 00007 #include <string> 00008 #include <sstream> 00009 00010 // TODO - add timestamp to motion samples 00011 #include "Motion.h" 00012 00013 Motion::Motion() { 00014 // These are motion deltas based on playing with 00015 // mbed application board motion sensors. 00016 // 00017 // Originally 0.097 was sufficient, but I get false 00018 // motion of 0.14 sometimes. This makes it somewhat less 00019 // responsive to small motion, but better than too many 00020 // false alarms. I should look into motion sensors to 00021 // see what is up or if something has been fixed?? 00022 min_motion.x = 0.15; 00023 min_motion.y = 0.15; 00024 min_motion.z = 0.15; 00025 max_samples_limit = 100; // Max motion buffer is 100 deep 00026 max_samples = 20; 00027 sample_ptr = 0; 00028 wrapped_once = false; 00029 // WARNING - width here is important - must be 7 characters! 00030 motion_samples.resize(max_samples,"0.00000"); 00031 } 00032 00033 bool Motion::change_max_samples(int num_samples) { 00034 // WARNING - setting this dumps all saved data! 00035 if (num_samples > 0 && num_samples <= max_samples_limit) { 00036 max_samples = num_samples; 00037 // WARNING - width here is important - must be 7 characters! 00038 motion_samples.resize(max_samples, "0.00000"); 00039 return true; 00040 } 00041 else { 00042 return false; 00043 } 00044 } 00045 00046 void Motion::add_sample(double sample) { 00047 std::string str_sample; 00048 convert_sample(sample, str_sample); 00049 if (sample_ptr == max_samples) { 00050 wrapped_once = true; 00051 sample_ptr = 0; 00052 motion_samples[sample_ptr] = str_sample; 00053 } 00054 else { 00055 motion_samples[sample_ptr++] = str_sample; 00056 } 00057 } 00058 00059 bool Motion::set_motion_thresh(motion_vec motion_thresh) { 00060 // FIXME - maybe add limit checking here. At least 00061 // limit to 7 character values? 00062 min_motion.x = motion_thresh.x; 00063 min_motion.y = motion_thresh.y; 00064 min_motion.z = motion_thresh.z; 00065 return true; 00066 } 00067 00068 motion_vec Motion::get_motion_thresh(void) { 00069 return min_motion; 00070 } 00071 00072 int Motion::get_max_samples() { 00073 return max_samples; 00074 } 00075 00076 const std::vector<std::string> &Motion::get_samples(void) { 00077 // FIXME - need to copy out samples in order of oldest 00078 // to newest or maybe not if change to contain timestamp 00079 // and let the host worry about adding it. If use multi-map 00080 // it would be sorted automatically. Maybe a better idea. 00081 return motion_samples; 00082 } 00083
Generated on Fri Jul 22 2022 02:01:25 by
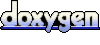