Library for my home monitoring classes and serial communication protocol. It monitors temperature and movement on the mbed application board.
Communication.h
00001 #ifndef HOME_MON_COMM_H 00002 #define HOME_MON_COMM_H 00003 00004 #include "USBHomeMon.h" 00005 #include <stdio.h> 00006 #include <vector> 00007 00008 /** Function to send an alert to the host 00009 * 00010 * @param alert - enum of different allowed alert types 00011 * @param return - pass or fail based on ack from host 00012 */ 00013 bool send_alert(alert_type alert); 00014 /** Function to wait for an ack from the host. 00015 * 00016 * @param return - true if host acked, false otherwise 00017 */ 00018 bool host_wait(); 00019 /** Function to check if host is alive 00020 * 00021 * Checks to see if there is any data on the serial port. If so, 00022 * checks command to see if it is a "connect". 00023 * 00024 * @param return - true if host sent connect message, false otherwise 00025 */ 00026 bool check_connection(); 00027 /** Function to get character over serial interface 00028 * 00029 * @param return - character received over serial interface 00030 */ 00031 char rec_command() ; 00032 /** Function to send multiple data samples to the host 00033 * 00034 * @param samples - vector of strings representing an array of values to be sent to host 00035 * @param return - true if host properly received data, false otherwise 00036 */ 00037 bool send_samples(std::vector<std::string> samples); 00038 /** Function to receive one sample from the host 00039 * 00040 * @param return - one sample value as a double 00041 */ 00042 double get_sample(void); 00043 /** Function to parse messages from the host. It takes the 00044 * motion and temperature class references to be able to set and get values. 00045 * 00046 * @param temp - reference to temperature object 00047 * @param motion - reference to motion object storing motion db, thresholds, etc. 00048 */ 00049 void host_command_resp(msg_type msg, Temperature &temp, Motion &motion); 00050 /** Function to parse the actual character received over serial interface 00051 * do error checking and if okay passes it on to host_command_resp() method 00052 * 00053 * @param msg - Character message received from the host 00054 */ 00055 msg_type parse_msg(char msg) ; 00056 00057 #endif
Generated on Fri Jul 22 2022 02:01:25 by
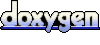