Library for my home monitoring classes and serial communication protocol. It monitors temperature and movement on the mbed application board.
Communication.cpp
00001 #include "USBHomeMon.h" 00002 #include <stdio.h> 00003 #include <stdlib.h> 00004 #include <vector> 00005 #include "Temperature.h" 00006 #include "HomeMonUtils.h" 00007 #include "USBSerial.h" 00008 #include "Motion.h" 00009 #include <sstream> 00010 #include <string> 00011 00012 extern USBSerial serial; 00013 00014 /* ! \fn Gets character from host */ 00015 int mon_get() { 00016 return serial._getc(); 00017 } 00018 00019 /// Sends character to the host 00020 int mon_send(char c) { 00021 return serial._putc(c); 00022 } 00023 00024 /// Waits for the host to ack 00025 bool host_wait() { 00026 char resp; 00027 // FIXME - check buffer size before waiting so 00028 // we don't block? 00029 if ((resp = mon_get()) == 'k') { 00030 return true; 00031 } 00032 else { 00033 return false; 00034 } 00035 } 00036 00037 /// Listens for connection message from host 00038 bool check_connection() { 00039 if (serial.available()) { 00040 if (mon_get() == 'c') { 00041 send_ack(); 00042 return true; 00043 } 00044 else { 00045 return false; 00046 } 00047 } 00048 } 00049 00050 /// Sends actual alert to the host 00051 bool send_alert(alert_type alert) { 00052 int response; 00053 mon_send('a'); // Send alert 00054 // Wait for response 00055 response = host_wait(); 00056 if (!response) { 00057 return false; 00058 } 00059 switch (alert) { 00060 case MOTION: 00061 mon_send('m'); 00062 break; 00063 case TEMP_LOW: 00064 mon_send('l'); 00065 break; 00066 case TEMP_HI: 00067 mon_send('h'); 00068 break; 00069 } 00070 response = host_wait(); 00071 if (!response) { 00072 return false; 00073 } 00074 00075 return true; 00076 } 00077 00078 char rec_command() { 00079 00080 char host_msg; 00081 00082 host_msg = mon_get(); 00083 00084 return host_msg; 00085 } 00086 00087 bool send_sample(double sample) { 00088 // Somewhat kludgy way of creating a fixed width field so 00089 // I don't have to worry about parsing. All samples are always 00090 // 7 characters wide and will be padded with spaces to ensure it. 00091 00092 std::string mystring; 00093 if (convert_sample(sample, mystring)) { 00094 for (size_t i=0; i < mystring.size(); ++i) { 00095 mon_send(mystring[i]); 00096 } 00097 return true; 00098 } 00099 else { 00100 printf("Error with %f\n", sample); 00101 send_err(); 00102 return false; 00103 } 00104 } 00105 00106 bool send_samples(std::vector<std::string> samples) { 00107 for (size_t i=0; i < samples.size(); ++i) { 00108 std::string tempstr = samples[i]; 00109 for (size_t j=0; j < tempstr.size(); ++j) { 00110 mon_send(tempstr[j]); 00111 } 00112 } 00113 00114 // FIXME - need to make sure samples were sent okay and return 00115 // proper value. 00116 return true; 00117 } 00118 00119 double get_sample(void) { 00120 char char_sample[SAMP_SIZE+1]; 00121 char_sample[SAMP_SIZE] = '\0'; 00122 for (int i=0; i<SAMP_SIZE; ++i) { 00123 char_sample[i] = mon_get(); 00124 } 00125 // FIXME - need error checking here on sample. Try 00126 // printing it to a string and make sure it will fit in 00127 // 7 characters or don't allow it to be set. 00128 // DEBUG printf("Sample = %s\n", char_sample); 00129 return atof(char_sample); 00130 } 00131 00132 msg_type parse_msg(char msg) { 00133 switch(msg) { 00134 case 'z': 00135 return SET_TEMP_MIN; 00136 break; 00137 case 'y': 00138 return SET_TEMP_MAX; 00139 break; 00140 case 'x': 00141 return SET_TEMP_NUM_SAMPLES; 00142 break; 00143 case 'w': 00144 return SET_MOTION_NUM_SAMPLES; 00145 break; 00146 case 'v': 00147 return SET_PERIOD; 00148 break; 00149 case 'u': 00150 return SET_MOTION_THRESH; 00151 break; 00152 case 't': 00153 return GET_TEMP_SAMPLES; 00154 break; 00155 case 's': 00156 return GET_MOTION_SAMPLES; 00157 break; 00158 case 'r': 00159 return GET_MIN_TEMP; 00160 break; 00161 case 'q': 00162 return GET_MAX_TEMP; 00163 break; 00164 case 'p': 00165 return GET_PERIOD; 00166 break; 00167 case 'o': 00168 return GET_TEMP_NUM_SAMPLES; 00169 break; 00170 case 'n': 00171 return GET_MOTION_NUM_SAMPLES; 00172 break; 00173 case 'j': 00174 return GET_MOTION_THRESH; 00175 break; 00176 default: 00177 printf("Received unknown command: %c\n", msg); 00178 return MON_ERROR; 00179 } 00180 } 00181 00182 void host_command_resp(msg_type msg, Temperature &temp, Motion &motion) { 00183 double sample, sample2, sample3; 00184 motion_vec motion_thresh; 00185 switch (msg) { 00186 case SET_TEMP_MIN: 00187 send_ack(); 00188 sample = get_sample(); 00189 // DEBUG printf(" sample = %f\n", sample); 00190 if(temp.set_min(sample)) { 00191 send_ack(); 00192 } 00193 else { 00194 printf("Error receiving sample\n"); 00195 send_err(); 00196 } 00197 break; 00198 case SET_TEMP_MAX: 00199 send_ack(); 00200 sample = get_sample(); 00201 if(temp.set_max(sample)) { 00202 send_ack(); 00203 } 00204 else { 00205 send_err(); 00206 } 00207 break; 00208 case SET_TEMP_NUM_SAMPLES: 00209 send_ack(); 00210 sample = get_sample(); 00211 if (temp.change_max_samples((int)sample)) { 00212 send_ack(); 00213 } 00214 else { 00215 send_err(); 00216 } 00217 break; 00218 case SET_MOTION_NUM_SAMPLES: 00219 send_ack(); 00220 sample = get_sample(); 00221 if (motion.change_max_samples((int)sample)) { 00222 send_ack(); 00223 } 00224 else { 00225 send_err(); 00226 } 00227 break; 00228 case SET_PERIOD: 00229 send_ack(); 00230 sample = get_sample(); 00231 if (temp.set_period(sample)) { 00232 send_ack(); 00233 } 00234 else { 00235 send_err(); 00236 } 00237 break; 00238 case SET_MOTION_THRESH: 00239 send_ack(); 00240 sample = get_sample(); 00241 sample2 = get_sample(); 00242 sample3 = get_sample(); 00243 motion_thresh.x = sample; 00244 motion_thresh.y = sample2; 00245 motion_thresh.z = sample3; 00246 if (motion.set_motion_thresh(motion_thresh)) { 00247 send_ack(); 00248 } 00249 else { 00250 send_err(); 00251 } 00252 break; 00253 case GET_TEMP_SAMPLES: 00254 send_ack(); 00255 send_sample(temp.get_max_samples()); 00256 send_samples(temp.get_samples()); 00257 break; 00258 case GET_MOTION_SAMPLES: 00259 send_ack(); 00260 send_sample(motion.get_max_samples()); 00261 send_samples(motion.get_samples()); 00262 break; 00263 case GET_MIN_TEMP: 00264 send_ack(); 00265 send_sample(temp.get_min()); 00266 break; 00267 case GET_MAX_TEMP: 00268 send_ack(); 00269 send_sample(temp.get_max()); 00270 break; 00271 case GET_PERIOD: 00272 send_ack(); 00273 send_sample(temp.get_period()); 00274 break; 00275 case GET_TEMP_NUM_SAMPLES: 00276 send_ack(); 00277 send_sample((double)temp.get_max_samples()); 00278 break; 00279 case GET_MOTION_NUM_SAMPLES: 00280 send_ack(); 00281 send_sample((double)motion.get_max_samples()); 00282 break; 00283 case GET_MOTION_THRESH: 00284 send_ack(); 00285 motion_thresh = motion.get_motion_thresh(); 00286 send_sample(motion_thresh.x); 00287 send_sample(motion_thresh.y); 00288 send_sample(motion_thresh.z); 00289 break; 00290 default: 00291 printf("Received unkown message type\n"); 00292 send_err(); 00293 break; 00294 } 00295 } 00296
Generated on Fri Jul 22 2022 02:01:25 by
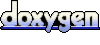