
master
Fork of master_working_1_2 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 00004 const int addr = 0x20; //slave address 00005 I2C master (D14,D15); //configure pins p27,p28 as I2C master 00006 Serial pc (USBTX,USBRX); 00007 DigitalOut interrupt(D9); 00008 InterruptIn data_ready(D10); 00009 00010 int reset; 00011 00012 typedef struct 00013 { 00014 char data[13]; // To avoid dynamic memory allocation 00015 int length; 00016 }i2c_data; 00017 00018 Mail<i2c_data,16> i2c_data_receive; 00019 Mail<i2c_data,16> i2c_data_send; 00020 00021 Thread * ptr_t_i2c; 00022 void FUNC_I2C_MASTER_FSLAVE(char * data,int length) 00023 { 00024 //printf("press backspace for master to start \n\r"); 00025 //while(1){ 00026 //interrupt = 0; 00027 // if(pc.getc()=='z'){ 00028 wait(0.5); 00029 interrupt = 1; 00030 printf("\nMaster executed\n"); 00031 00032 00033 bool ack0 =true; 00034 bool loopvariable0 =true; 00035 //char *data = new char; 00036 //*data = 'b'; 00037 00038 00039 00040 master.frequency(100000); //set clock frequency 00041 //master.start(); 00042 /*while(write2slave) 00043 { *writedata = pc.getc(); 00044 wait(0.5); 00045 00046 printf("master clk freq setup and addressing slave\n\r"); 00047 master.start(); //initiating the data transfer 00048 master_status_write = (bool) master.write(addr|0x00,writedata,1); 00049 if(master_status_write==0) 00050 { 00051 printf("master has written %c to slave\n\r",*writedata); 00052 write2slave=false; 00053 } 00054 00055 00056 }*/ 00057 00058 while(ack0) 00059 { 00060 //printf("2 master clk freq setup and addressing slave\n\r"); 00061 00062 master.start(); //initiating the data transfer 00063 ack0 = master.read(addr,data,length); 00064 } 00065 if(!ack0) 00066 { 00067 printf("\n master has read %s from slave\n\r",data); 00068 loopvariable0=false; 00069 } 00070 //master.stop(); 00071 00072 //printf("done\n\r"); 00073 //delete data; 00074 interrupt=0; 00075 } 00076 00077 void T_I2C_MASTER_FSLAVE(void const *args) 00078 { 00079 char data_receive[13]; 00080 while(1) 00081 { 00082 Thread::signal_wait(0x1); 00083 FUNC_I2C_MASTER_FSLAVE(data_receive,13); 00084 i2c_data * i2c_data_r = i2c_data_receive.alloc(); 00085 strcpy(i2c_data_r->data , data_receive); 00086 i2c_data_r->length = 13; 00087 i2c_data_receive.put(i2c_data_r); 00088 printf("\n Data received from slave is %s\n\r",data_receive); 00089 i2c_data_receive.free(i2c_data_r); 00090 } 00091 } 00092 00093 void FUNC_INT() 00094 { 00095 00096 ptr_t_i2c->signal_set(0x1); 00097 00098 } 00099 00100 char writedata[13]; 00101 void FUNC_MASTER_WRITE() 00102 { 00103 bool write2slave=true; 00104 00105 interrupt = 0; 00106 00107 char data[13]="shakti priya"; 00108 i2c_data * i2c_data_s = i2c_data_send.alloc(); 00109 strcpy(i2c_data_s->data ,data); 00110 i2c_data_s->length = 13; 00111 i2c_data_send.put(i2c_data_s); 00112 bool master_status_write = true; 00113 interrupt = 1; 00114 osEvent evt = i2c_data_send.get(); 00115 if (evt.status == osEventMail) 00116 { 00117 i2c_data *i2c_data_s = (i2c_data*)evt.value.p; 00118 strcpy(writedata ,i2c_data_s -> data); 00119 master.frequency(100000); //set clock frequency 00120 while(write2slave) 00121 { 00122 printf("master clk freq setup and addressing slave\n\r"); 00123 master.start(); //initiating the data transfer 00124 master_status_write = (bool) master.write(addr|0x00,writedata,13); 00125 if(master_status_write==0) 00126 { 00127 printf("master has written %s to slave\n\r",writedata); 00128 write2slave=false; 00129 } 00130 i2c_data_send.free(i2c_data_s); 00131 } 00132 } 00133 } 00134 00135 00136 00137 int main() 00138 { 00139 ptr_t_i2c = new Thread(T_I2C_MASTER_FSLAVE); 00140 data_ready.rise(&FUNC_INT); 00141 printf("\nstarted master\n"); 00142 while(1) 00143 { 00144 //Thread::wait(10000); 00145 // FUNC_MASTER_WRITE(); 00146 ; 00147 } 00148 }
Generated on Wed Jul 13 2022 02:09:14 by
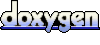