Class library for using the true Random Number Generator on STM32F4xxx devices.
Dependents: MCU-Benchmark-Sute Doom_Flame-F429ZI_v02 Wether_Meter
STM32F4_RNG.h
00001 /* STM32F4_RNG Library v1.0 00002 * Copyright (c) 2016 Grant Phillips 00003 * grant.phillips@nmmu.ac.za 00004 * 00005 * This library was adapted from tm_stm32f4_rng written by Tilen Majerle. 00006 * (http://stm32f4-discovery.com/2014/07/library-22-true-random-number-generator-stm32f4xx/) 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 */ 00026 00027 #ifndef STM32F4_RNG_H 00028 #define STM32F4_RNG_H 00029 00030 #include "mbed.h" 00031 00032 /** Class library for using the true Random Number Generator on STM32F4xxx devices. 00033 * 00034 * Example: 00035 * @code 00036 * #include "mbed.h" 00037 * #include "STM32F4_RNG.h" 00038 * #include "SWO.h" 00039 * 00040 * STM32F4_RNG rnd; 00041 * 00042 * int main() { 00043 * // create a 32-bit unsigned variable 00044 * unsigned long num; //or uint32_t num 00045 * 00046 * while(1) { 00047 * num = rnd.Get(); 00048 * printf("%u\n", num); 00049 * wait(1.0); 00050 * } 00051 * } 00052 * @endcode 00053 */ 00054 00055 class STM32F4_RNG { 00056 public: 00057 /** Create a STM32F4_RNG object. 00058 * 00059 */ 00060 STM32F4_RNG(); 00061 00062 /** Gets a 32-bit random number. 00063 * @param 00064 * None 00065 * @return 00066 * 32-bit random number. 00067 */ 00068 unsigned long Get(); 00069 }; 00070 00071 #endif
Generated on Fri Jul 15 2022 20:32:14 by
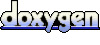