asdf
Dependencies: L3GD20 LSM303DLHC mbed
BufferAverage.h
00001 #ifndef BUFFER_AVERAGE_H 00002 #define BUFFER_AVERAGE_H 00003 00004 #include "Communication.h" 00005 00006 // A buffer size of 128 updated every 4ms or so gives us and average for the last half second. 00007 #define BUFFER_SIZE 128 00008 class BufferAverage 00009 { 00010 public: 00011 explicit BufferAverage() 00012 { 00013 reset(); 00014 } 00015 00016 void reset() 00017 { 00018 ptr = 0; 00019 total = 0; 00020 for(int i = 0; i < BUFFER_SIZE; i++) 00021 buff[i] = 0.0f; 00022 } 00023 00024 void add(float reading) 00025 { 00026 WIRELESS.printf("%i \n\r", (int)(ptr % BUFFER_SIZE)); 00027 total -= buff[ptr % BUFFER_SIZE]; 00028 total += reading; 00029 buff[ptr++] = reading; 00030 } 00031 00032 float average() 00033 { 00034 return total / (BUFFER_SIZE > ptr ? BUFFER_SIZE : ptr); //min(BUFFER_SIZE, ptr); 00035 } 00036 00037 private: 00038 unsigned long ptr; 00039 float total; 00040 float buff [BUFFER_SIZE]; 00041 }; 00042 00043 BufferAverage leftBufferAvg; 00044 BufferAverage rightBufferAvg; 00045 BufferAverage frontBufferAvg; 00046 #endif
Generated on Tue Jul 12 2022 19:34:20 by
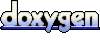