Buffer from Sam Grove changed to have static instead of dynamic memory allocation. Fixed size to 256B.
Dependents: BufferedSerialStatic
Fork of Buffer by
MyBuffer.h
00001 00002 /** 00003 * @file Buffer.h 00004 * @brief Software Buffer - Templated Ring Buffer for most data types 00005 * @author sam grove 00006 * @version 1.0 00007 * @see 00008 * 00009 * Copyright (c) 2013 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); 00012 * you may not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, 00019 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 */ 00023 00024 /** 00025 * Modification of original code to implement buffer with static memory 00026 * allocation with size 256. 00027 */ 00028 00029 #ifndef MYBUFFER_H 00030 #define MYBUFFER_H 00031 00032 #include <stdint.h> 00033 #include <string.h> 00034 00035 template <typename T> 00036 class MyBuffer 00037 { 00038 private: 00039 const static uint16_t BUFFER_SIZE = 256; 00040 T _buf[BUFFER_SIZE]; 00041 volatile uint32_t _wloc; 00042 volatile uint32_t _rloc; 00043 uint32_t _size; 00044 00045 public: 00046 /** Create a Buffer and allocate memory for it 00047 * @param size The size of the buffer 00048 */ 00049 MyBuffer(); 00050 00051 /** Get the size of the ring buffer 00052 * @return the size of the ring buffer 00053 */ 00054 uint32_t getSize(); 00055 00056 /** Destry a Buffer and release it's allocated memory 00057 */ 00058 ~MyBuffer(); 00059 00060 /** Add a data element into the buffer 00061 * @param data Something to add to the buffer 00062 */ 00063 void put(T data); 00064 00065 /** Remove a data element from the buffer 00066 * @return Pull the oldest element from the buffer 00067 */ 00068 T get(void); 00069 00070 /** Get the address to the head of the buffer 00071 * @return The address of element 0 in the buffer 00072 */ 00073 T *head(void); 00074 00075 /** Reset the buffer to 0. Useful if using head() to parse packeted data 00076 */ 00077 void clear(void); 00078 00079 /** Determine if anything is readable in the buffer 00080 * @return 1 if something can be read, 0 otherwise 00081 */ 00082 uint32_t available(void); 00083 00084 /** Overloaded operator for writing to the buffer 00085 * @param data Something to put in the buffer 00086 * @return 00087 */ 00088 MyBuffer &operator= (T data) 00089 { 00090 put(data); 00091 return *this; 00092 } 00093 00094 /** Overloaded operator for reading from the buffer 00095 * @return Pull the oldest element from the buffer 00096 */ 00097 operator int(void) 00098 { 00099 return get(); 00100 } 00101 00102 uint32_t peek(char c); 00103 00104 }; 00105 00106 template <class T> 00107 inline void MyBuffer<T>::put(T data) 00108 { 00109 _buf[_wloc++] = data; 00110 _wloc %= (_size-1); 00111 00112 return; 00113 } 00114 00115 template <class T> 00116 inline T MyBuffer<T>::get(void) 00117 { 00118 T data_pos = _buf[_rloc++]; 00119 _rloc %= (_size-1); 00120 00121 return data_pos; 00122 } 00123 00124 template <class T> 00125 inline T *MyBuffer<T>::head(void) 00126 { 00127 T *data_pos = &_buf[0]; 00128 00129 return data_pos; 00130 } 00131 00132 template <class T> 00133 inline uint32_t MyBuffer<T>::available(void) 00134 { 00135 return (_wloc == _rloc) ? 0 : 1; 00136 } 00137 00138 #endif 00139
Generated on Wed Jul 13 2022 09:34:03 by
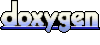