Utility library for HSP SPo2 HR demo including user interface, board support adn accelerometer.
Embed:
(wiki syntax)
Show/hide line numbers
MAX20303.h
00001 /******************************************************************************* 00002 * Copyright (C) 2018 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef __MAX20303_H_ 00034 #define __MAX20303_H_ 00035 00036 #include "mbed.h" 00037 00038 #define MAX20303_SLAVE_ADDR (0x50 >> 1) 00039 #define MAX20303_SLAVE_WR_ADDR ((MAX20303_SLAVE_ADDR << 1)) 00040 #define MAX20303_SLAVE_RD_ADDR ((MAX20303_SLAVE_ADDR << 1) | 1) 00041 00042 00043 #define MAX20303_NO_ERROR 0 00044 #define MAX20303_ERROR -1 00045 00046 #define MAX20303_I2C_ADDR_FUEL_GAUGE 0x6c 00047 #define MAX20303_I2C_ADDR_FUEL_GAUGE 0x6C 00048 00049 #define MAX20303_LDO_MIN_MV 800 00050 #define MAX20303_LDO_MAX_MV 3600 00051 #define MAX20303_LDO_STEP_MV 100 00052 00053 #define MAX20303_OFF_COMMAND 0xB2 00054 00055 class MAX20303 00056 { 00057 00058 public: 00059 /** 00060 * @brief Register Addresses 00061 * @details Enumerated MAX20303 register addresses 00062 */ 00063 enum registers_t { 00064 REG_HARDWARE_ID = 0x00, ///< HardwareID Register 00065 REG_FIRMWARE_REV = 0x01, ///< FirmwareID Register 00066 // = 0x02, ///< 00067 REG_INT0 = 0x03, ///< Int0 Register 00068 REG_INT1 = 0x04, ///< Int1 Register 00069 REG_INT2 = 0x05, ///< Int2 Register 00070 REG_STATUS0 = 0x06, ///< Status Register 0 00071 REG_STATUS1 = 0x07, ///< Status Register 1 00072 REG_STATUS2 = 0x08, ///< Status Register 2 00073 REG_STATUS3 = 0x09, ///< Status Register 2 00074 // = 0x0A, ///< 00075 REG_SYSTEM_ERROR = 0x0B, ///< SystemError Register 00076 REG_INT_MASK0 = 0x0C, ///< IntMask0 Register 00077 REG_INT_MASK1 = 0x0D, ///< IntMask1 Register 00078 REG_INT_MASK2 = 0x0E, ///< IntMask1 Register 00079 REG_AP_DATOUT0 = 0x0F, ///< APDataOut0 Register 00080 REG_AP_DATOUT1 = 0x10, ///< APDataOut1 Register 00081 REG_AP_DATOUT2 = 0x11, ///< APDataOut2 Register 00082 REG_AP_DATOUT3 = 0x12, ///< APDataOut3 Register 00083 REG_AP_DATOUT4 = 0x13, ///< APDataOut4 Register 00084 REG_AP_DATOUT5 = 0x14, ///< APDataOut5 Register 00085 REG_AP_DATOUT6 = 0x15, ///< APDataOut6 Register 00086 REG_AP_CMDOUT = 0x17, ///< APCmdOut Register 00087 REG_AP_RESPONSE = 0x18, ///< APResponse Register 00088 REG_AP_DATAIN0 = 0x19, 00089 REG_AP_DATAIN1 = 0x1A, 00090 REG_AP_DATAIN2 = 0x1B, 00091 REG_AP_DATAIN3 = 0x1C, 00092 REG_AP_DATAIN4 = 0x1D, 00093 REG_AP_DATAIN5 = 0x1E, 00094 // = 0x1F, ///< 00095 REG_LDO_DIRECT = 0x20, 00096 REG_MPC_DIRECTWRITE = 0x21, 00097 REG_MPC_DIRECTRED = 0x22, 00098 00099 REG_LED_STEP_DIRECT = 0x2C, 00100 REG_LED0_DIRECT = 0x2D, 00101 REG_LED1_DIRECT = 0x2E, 00102 REG_LED2_DIRECT = 0x2F, 00103 00104 00105 REG_LDO1_CONFIG_WRITE = 0x40, 00106 REG_LDO1_CONFIG_READ = 0x41, 00107 REG_LDO2_CONFIG_WRITE = 0x42, 00108 REG_LDO2_CONFIG_READ = 0x43 00109 00110 /* 00111 REG_CHG_TMR = 0x0C, ///< Charger Timers 00112 REG_BUCK1_CFG = 0x0D, ///< Buck 1 Configuration 00113 REG_BUCK1_VSET = 0x0E, ///< Buck 1 Voltage Setting 00114 REG_BUCK2_CFG = 0x0F, ///< Buck 2 Configuration 00115 REG_BUCK2_VSET = 0x10, ///< Buck 2 Voltage Setting 00116 REG_RSVD_11 = 0x11, ///< Reserved 0x11 00117 REG_LDO1_CFG = 0x12, ///< LDO 1 Configuration 00118 REG_LDO1_VSET = 0x13, ///< LDO 1 Voltage Setting 00119 REG_LDO2_CFG = 0x14, ///< LDO 2 Configuration 00120 REG_LDO2_VSET = 0x15, ///< LDO 2 Voltage Setting 00121 REG_LDO3_CFG = 0x16, ///< LDO 3 Configuration 00122 REG_LDO3_VSET = 0x17, ///< LDO 3 Voltage Setting 00123 REG_THRM_CFG = 0x18, ///< Thermistor Configuration 00124 REG_MON_CFG = 0x19, ///< Monitor Multiplexer Configuration 00125 REG_BOOT_CFG = 0x1A, ///< Boot Configuration 00126 REG_PIN_STATUS = 0x1B, ///< Pin Status 00127 REG_BUCK_EXTRA = 0x1C, ///< Additional Buck Settings 00128 REG_PWR_CFG = 0x1D, ///< Power Configuration 00129 REG_NULL = 0x1E, ///< Reserved 0x1E 00130 REG_PWR_OFF = 0x1F, ///< Power Off Register 00131 */ 00132 }; 00133 00134 /** 00135 * @brief Constructor using reference to I2C object 00136 * @param i2c - Reference to I2C object 00137 * @param slaveAddress - 7-bit I2C address 00138 */ 00139 MAX20303(I2C *i2c); 00140 00141 /** @brief Destructor */ 00142 ~MAX20303(void); 00143 00144 int led0on(char enable); 00145 int led1on(char enable); 00146 int led2on(char enable); 00147 int BoostEnable(void); 00148 int BuckBoostEnable(void); 00149 00150 /// @brief Enable the 1.8V output rail **/ 00151 int LDO1Config(void); 00152 00153 /// @brief Enable the 3V output rail **/ 00154 int LDO2Config(void); 00155 00156 00157 int mv2bits(int mV); 00158 00159 /** @brief Power Off the board 00160 */ 00161 int PowerOffthePMIC(); 00162 00163 /** @brief Power Off the board with 30ms delay 00164 */ 00165 int PowerOffDelaythePMIC(); 00166 00167 /** @brief Soft reset the PMIC 00168 */ 00169 int SoftResetthePMIC(); 00170 00171 /** @brief Hard reset the PMIC 00172 */ 00173 int HardResetthePMIC(); 00174 00175 /** @brief check if can communicate with max20303 00176 */ 00177 char CheckPMICHWID(); 00178 00179 /** @brief CheckPMICStatusRegisters 00180 */ 00181 int CheckPMICStatusRegisters(unsigned char buf_results[5]); 00182 00183 int Max20303_BatteryGauge(unsigned char *batterylevel); 00184 00185 private: 00186 00187 int writeReg(registers_t reg, uint8_t value); 00188 int readReg(registers_t reg, uint8_t &value); 00189 00190 int writeRegMulti(registers_t reg, uint8_t *value, uint8_t len); 00191 int readRegMulti(registers_t reg, uint8_t *value, uint8_t len); 00192 00193 /// I2C object 00194 I2C *m_i2c; 00195 00196 /// Device slave addresses 00197 uint8_t m_writeAddress, m_readAddress; 00198 00199 // Application Processor Interface Related Variables 00200 uint8_t i2cbuffer_[16]; 00201 uint8_t appdatainoutbuffer_[8]; 00202 uint8_t appcmdoutvalue_; 00203 00204 00205 /** @brief API Related Functions ***/ 00206 00207 /*** 00208 * @brief starts writing from ApResponse register 0x0F 00209 * check the datasheet to determine the value of dataoutlen 00210 */ 00211 int AppWrite(uint8_t dataoutlen); 00212 00213 /** @brief starts reading from ApResponse register 0x18 00214 * check the datasheet to determine the value of datainlen 00215 * the result values are written into i2cbuffer 00216 * 00217 */ 00218 int AppRead(uint8_t datainlen); 00219 }; 00220 00221 #endif /* __MAX20303_H_ */
Generated on Fri Jul 15 2022 01:41:58 by
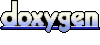