
BBC Basic in Z80 emulation on the mbed, USB serial terminal output only. LOAD and SAVE work on the local file system but there is no error signalling.
module.h
00001 // ------------------------------------------------------------- 00002 // BBC Basic hardware emulation part 00003 // ------------------------------------------------------------- 00004 00005 #include "bbc_0100.h" 00006 00007 #include "mbed.h" 00008 #define byte unsigned char 00009 #define word unsigned short 00010 00011 // some defaults 00012 #define ESCFLG 0xff80 // escape flag 00013 #define FAULT 0xff82 // fault address 00014 #define DEFERR 0xff84 // default error handler 00015 #define CMDPTR 0xff86 // command line tail 00016 #define SCRATCHPAD 0xff88 // Basic scratchpad area 00017 #define RESERVED 0xff9a 00018 #define OSRDRM 0xffb9 // read byte in paged ROM 00019 #define MAINVDU 0xffbc // main VDU character output entry point 00020 #define OSEVEN 0xffbf // generate an event 00021 #define GSINIT 0xffc2 // general string input initialise routine 00022 #define GSREAD 0xffc5 // read character from string input 00023 #define UKCMD 0xffc9 // unknown command 00024 #define OSFSC 0xffcb // filing system control 00025 #define OSFIND 0xffce // open or close a file 00026 #define OSGBPB 0xffd1 // multiple byte access 00027 #define OSBPUT 0xffd4 // put a byte to a file 00028 #define OSBGET 0xffd7 // get a byte from a file 00029 #define OSARGS 0xffda // read or set file arguments 00030 #define OSFILE 0xffdd // load or save a file 00031 #define OSRDCH 0xffe0 // input a character 00032 #define OSASCI 0xffe3 // print a character with CR converted to LF, CR 00033 #define OSNEWL 0xffe7 // print a LF, CR sequence 00034 #define OSWRCH 0xffee // print a character 00035 #define OSWORD 0xfff1 // do an OSWORD call 00036 #define OSBYTE 0xfff4 // do an OSBYTE call 00037 #define OSCLI 0xfff7 // interpret a command 00038 #define BRKV 0xfffa // FAULT vector 00039 00040 // default CP/M ram pages 00041 #define RAMSIZE (word)0x4000 // 16K ram 00042 #define RAMSTART 0x34ee 00043 #define RAMEND RAMSTART+RAMSIZE 00044 #define PAGE 0x3800 00045 00046 // the emulated ram space 00047 volatile unsigned char ram[RAMSIZE]; 00048 00049 // ramtop space with vectors 00050 volatile unsigned char ramtop[0x80]; 00051 00052 00053 // read from memory space 00054 unsigned char rdmem(word addr) { 00055 extern volatile unsigned short pc; 00056 00057 // all rom space 00058 if (addr<RAMSTART) { 00059 return rom[addr]; 00060 } 00061 00062 // ramtop with vectors 00063 if ((addr >=0xff80) && (addr <=0xffff)) { 00064 return ramtop[addr-0xff80]; 00065 } 00066 00067 // relocate $3400 00068 if ((addr >= RAMSTART) && (addr < RAMEND)) { 00069 return ram[addr-0x34ee]; 00070 } 00071 return 0xff; // non existant memory reads as 0xff 00072 } 00073 00074 // write to memory space 00075 void wrmem (word addr, byte val) { 00076 extern volatile unsigned short pc; 00077 00078 // memtop vector space 00079 if ((addr >=0xff80) && (addr <=0xffff)) { 00080 ramtop[addr-0xff80]=val; 00081 return; 00082 } 00083 // printf("MEMW %04x %02x\n\r",addr,val); 00084 if ((addr >= RAMSTART) && (addr < RAMEND)) { 00085 ram[addr-RAMSTART] = val; 00086 } 00087 } 00088 00089 // write a byte to IO space 00090 void out(unsigned char addr, unsigned char val) { 00091 printf("IO out called %02d, %02d\n\r",addr,val); 00092 } 00093 00094 // read a byte from IO space 00095 unsigned char in(unsigned char addr) { 00096 printf("IO in called %02d\n\r",addr); 00097 return 0xff; 00098 } 00099
Generated on Wed Jul 13 2022 16:07:28 by
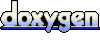