Counts pulses on pin p29. Pulse frequency can be up to 48 MHz.
Embed:
(wiki syntax)
Show/hide line numbers
PulseCounter.h
00001 /* Copyright (c) 2012 Christian Buntebarth, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef MBED_PULSECOUNTER_H 00020 #define MBED_PULSECOUNTER_H 00021 00022 #include "mbed.h" 00023 00024 const int _pulseCountArrayLength = 20; 00025 00026 /** Counts pulses on pin29 coming in with a frequency up to 48 MHz. The counter is read (and reset to 0) by rising edge (trigger event) on pin30. 00027 * These events can be triggered at a rate up to 9kHz. The counter results are stored in a "circular array" of 20 elements. 00028 * ("circular array" = array pointer increments each time a new value is stored into the array; if pointer reaches last element, it is redirected to first element) 00029 * */ 00030 class PulseCounter 00031 { 00032 public: 00033 00034 /** Initializer for PulseCounter instance 00035 */ 00036 void init(); 00037 00038 /** Start counter 00039 */ 00040 void start(); 00041 00042 /** Stop counter 00043 */ 00044 void stop(); 00045 00046 /** Read pulse count results into external array. Do not call this method before you called method stop() - otherwise it will return zeros. 00047 * @param counterArray = Pointer to array in which the pulse count results should be read into. 00048 * @param counterArrayLength = Size of counterArray. Should be 20 or less. If more than 20, remaining elements will be filled with zeros. 00049 * @code #include "mbed.h" 00050 #include "PwmOscillator.h" 00051 #include "PulseCounter.h" 00052 00053 Serial usbPC(USBTX, USBRX); // sends log messages via USB to PC terminal 00054 PwmOscillator pulseGenerator; // generates pulses to be count (48 MHz) on pin p22. Connect this pin to p29 (counter input). 00055 PulseCounter pulseCounter; // counts the pulses on pin p29 between trigger events (= rising edges) on pin p30. 00056 00057 int main() 00058 { 00059 usbPC.printf("---> start <---\n"); 00060 pulseGenerator.initWithFrequency(48000000); 00061 pulseCounter.init(); 00062 pulseGenerator.start(); 00063 00064 pulseCounter.start(); 00065 wait(1); // waiting 1 second for trigger events (pin30: rising edge). Count pulses between trigger events. 00066 pulseCounter.stop(); 00067 00068 pulseGenerator.stop(); 00069 00070 // read & print pulseCounter results 00071 int pulseCounterResults[20]; 00072 pulseCounter.counterArray(&pulseCounterResults[0],sizeof pulseCounterResults / sizeof (int)); 00073 00074 for(int i = 0; i < (sizeof pulseCounterResults / sizeof (int)); i++) { 00075 usbPC.printf("counter of trigger event %i = %i\n",i,pulseCounterResults[i]); 00076 } 00077 // 00078 00079 usbPC.printf(" finished.\n"); 00080 } 00081 * @endcode */ 00082 void counterArray(uint32_t* counterArray, int counterArrayLength); 00083 00084 private: 00085 00086 static void _triggerInterrupt(); 00087 static PulseCounter *instance; 00088 bool _hasFinishedCounting; 00089 00090 volatile int _triggerCounter; 00091 volatile uint32_t *_arrayPointer; 00092 volatile uint32_t _pulseCountArray[_pulseCountArrayLength + 1]; 00093 }; 00094 00095 #endif
Generated on Fri Jul 15 2022 01:47:36 by
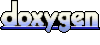