
módulo WIFI para comunicación de KL25Z midiendo corriente a través de una sonda amperimétrica con Android App neurGAI
Dependencies: WiflyInterface mbed
Fork of wiflyServer by
main.cpp
00001 // el código de la aplicación Android está accesibl en https://github.com/gorkabueno/NeurGai 00002 00003 //#define DEBUG 00004 #include "mbed.h" 00005 #include "WiflyInterface.h" 00006 00007 #if (defined(DEBUG)) 00008 #define DBG(x, ...) std::printf("[neurGAI : DBG]"x"\r\n", ##__VA_ARGS__); 00009 #define WARN(x, ...) std::printf("[neurGAI : WARN]"x"\r\n", ##__VA_ARGS__); 00010 #define ERR(x, ...) std::printf("[neurGAI : ERR]"x"\r\n", ##__VA_ARGS__); 00011 #else 00012 #define DBG(x, ...) 00013 #define WARN(x, ...) 00014 #define ERR(x, ...) 00015 #endif 00016 00017 #define ECHO_SERVER_PORT 7 00018 00019 WiflyInterface wifly(D14, D15, D5, LED1, "NeurGai", "neurGai2016", WPA); 00020 00021 Ticker medidor; 00022 00023 AnalogIn sonda(A2); // la sonda amperimétrica debe conectarse entre los pines A2 y PTE30 00024 AnalogOut salida_offset(PTE30); 00025 00026 int frecuencia_muestreo = 5000; // con 8000 Hz hace bien el muestreo, pero el volcado del debug no es completo 00027 int numero_muestra = 0; 00028 float valor_muestra; 00029 float v_offset = 0; 00030 float v_offset_medido = 0; 00031 float potencia = 0; 00032 float potencia_medida = 0; 00033 float potencia_230V =0; 00034 float corriente_medida = 0; 00035 bool medido = false; 00036 bool medido_y_enviado = false; 00037 00038 float pendiente = 201.206437; 00039 float offset = 0; 00040 00041 int num_medida = 0; 00042 00043 TCPSocketServer server; 00044 TCPSocketConnection client; 00045 00046 char buffer[256]; // string con los datos 00047 00048 00049 void medir() 00050 { 00051 numero_muestra++; 00052 valor_muestra = sonda.read(); 00053 v_offset = v_offset + valor_muestra; 00054 potencia = potencia + pow((valor_muestra - v_offset_medido), 2); 00055 if (numero_muestra == frecuencia_muestreo) // hemos llegado a un segundo 00056 { 00057 medidor.detach(); 00058 potencia_medida = potencia / frecuencia_muestreo; 00059 corriente_medida = sqrt(potencia_medida); 00060 v_offset_medido = v_offset / frecuencia_muestreo; 00061 v_offset = 0; 00062 potencia = 0; 00063 numero_muestra = 0; 00064 medido = true; 00065 potencia_230V = (corriente_medida * pendiente + offset) * 230; 00066 sprintf(buffer, "#%i&%f*\n", num_medida, potencia_230V); 00067 DBG("%s %i", buffer, strlen(buffer)); 00068 int numDatosEnviados = client.send_all(buffer, strlen(buffer)); 00069 medido = false; 00070 num_medida++; 00071 medido_y_enviado = true; 00072 DBG("datos enviados : %i", numDatosEnviados); 00073 } 00074 } 00075 00076 int main (void) 00077 { 00078 DBG("Empezando..."); 00079 wifly.init(); // use DHCP 00080 while (!wifly.connect()); // join the network 00081 DBG("\nLa direccion IP es %s", wifly.getIPAddress()); 00082 00083 server.bind(ECHO_SERVER_PORT); 00084 server.listen(); 00085 00086 DBG("\nEsperando conexion..."); 00087 server.accept(client); 00088 DBG("\nServidor aceptado..."); 00089 wait(1); //si no se le mete un pequeño retardo, se queda colgado. También vale con el DBG anterior 00090 00091 //configura el offset de voltaje a sumar a la sonda 00092 salida_offset = 0.5; 00093 00094 while (true) { 00095 DBG("Esperando dato..."); 00096 int n = client.receive(buffer, sizeof(buffer)); 00097 if (n <= 0) continue; 00098 DBG("Recibido dato: %s Longitud %i", buffer, n); 00099 buffer[n] = 0; 00100 if (strcmp(buffer, "Pot?\n") == 0) { 00101 // activa el muestreo 00102 medido_y_enviado = false; 00103 medidor.attach(&medir, 1.0/frecuencia_muestreo); 00104 } 00105 00106 // spin in a main loop. medidor will interrupt it to call medir 00107 while (!medido_y_enviado) 00108 wait(.1); 00109 } 00110 }
Generated on Wed Jul 20 2022 14:56:26 by
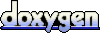