ROS Serial library for Mbed platforms for ROS Indigo Igloo. Check http://wiki.ros.org/rosserial_mbed/ for more information
Dependents: rosserial_mbed_hello_world_publisher rtos_base_control rosserial_mbed_F64MA ROS-RTOS ... more
TwoIntsGoal.h
00001 #ifndef _ROS_actionlib_TwoIntsGoal_h 00002 #define _ROS_actionlib_TwoIntsGoal_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace actionlib 00010 { 00011 00012 class TwoIntsGoal : public ros::Msg 00013 { 00014 public: 00015 int64_t a; 00016 int64_t b; 00017 00018 TwoIntsGoal(): 00019 a(0), 00020 b(0) 00021 { 00022 } 00023 00024 virtual int serialize(unsigned char *outbuffer) const 00025 { 00026 int offset = 0; 00027 union { 00028 int64_t real; 00029 uint64_t base; 00030 } u_a; 00031 u_a.real = this->a; 00032 *(outbuffer + offset + 0) = (u_a.base >> (8 * 0)) & 0xFF; 00033 *(outbuffer + offset + 1) = (u_a.base >> (8 * 1)) & 0xFF; 00034 *(outbuffer + offset + 2) = (u_a.base >> (8 * 2)) & 0xFF; 00035 *(outbuffer + offset + 3) = (u_a.base >> (8 * 3)) & 0xFF; 00036 *(outbuffer + offset + 4) = (u_a.base >> (8 * 4)) & 0xFF; 00037 *(outbuffer + offset + 5) = (u_a.base >> (8 * 5)) & 0xFF; 00038 *(outbuffer + offset + 6) = (u_a.base >> (8 * 6)) & 0xFF; 00039 *(outbuffer + offset + 7) = (u_a.base >> (8 * 7)) & 0xFF; 00040 offset += sizeof(this->a); 00041 union { 00042 int64_t real; 00043 uint64_t base; 00044 } u_b; 00045 u_b.real = this->b; 00046 *(outbuffer + offset + 0) = (u_b.base >> (8 * 0)) & 0xFF; 00047 *(outbuffer + offset + 1) = (u_b.base >> (8 * 1)) & 0xFF; 00048 *(outbuffer + offset + 2) = (u_b.base >> (8 * 2)) & 0xFF; 00049 *(outbuffer + offset + 3) = (u_b.base >> (8 * 3)) & 0xFF; 00050 *(outbuffer + offset + 4) = (u_b.base >> (8 * 4)) & 0xFF; 00051 *(outbuffer + offset + 5) = (u_b.base >> (8 * 5)) & 0xFF; 00052 *(outbuffer + offset + 6) = (u_b.base >> (8 * 6)) & 0xFF; 00053 *(outbuffer + offset + 7) = (u_b.base >> (8 * 7)) & 0xFF; 00054 offset += sizeof(this->b); 00055 return offset; 00056 } 00057 00058 virtual int deserialize(unsigned char *inbuffer) 00059 { 00060 int offset = 0; 00061 union { 00062 int64_t real; 00063 uint64_t base; 00064 } u_a; 00065 u_a.base = 0; 00066 u_a.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00067 u_a.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00068 u_a.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00069 u_a.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00070 u_a.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00071 u_a.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00072 u_a.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00073 u_a.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00074 this->a = u_a.real; 00075 offset += sizeof(this->a); 00076 union { 00077 int64_t real; 00078 uint64_t base; 00079 } u_b; 00080 u_b.base = 0; 00081 u_b.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00082 u_b.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00083 u_b.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00084 u_b.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00085 u_b.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00086 u_b.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00087 u_b.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00088 u_b.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00089 this->b = u_b.real; 00090 offset += sizeof(this->b); 00091 return offset; 00092 } 00093 00094 const char * getType(){ return "actionlib/TwoIntsGoal"; }; 00095 const char * getMD5(){ return "36d09b846be0b371c5f190354dd3153e"; }; 00096 00097 }; 00098 00099 } 00100 #endif
Generated on Tue Jul 12 2022 18:39:41 by
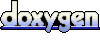