ROS Serial library for Mbed platforms for ROS Indigo Igloo. Check http://wiki.ros.org/rosserial_mbed/ for more information
Dependents: rosserial_mbed_hello_world_publisher rtos_base_control rosserial_mbed_F64MA ROS-RTOS ... more
Pose2D.h
00001 #ifndef _ROS_geometry_msgs_Pose2D_h 00002 #define _ROS_geometry_msgs_Pose2D_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace geometry_msgs 00010 { 00011 00012 class Pose2D : public ros::Msg 00013 { 00014 public: 00015 double x; 00016 double y; 00017 double theta; 00018 00019 Pose2D(): 00020 x(0), 00021 y(0), 00022 theta(0) 00023 { 00024 } 00025 00026 virtual int serialize(unsigned char *outbuffer) const 00027 { 00028 int offset = 0; 00029 union { 00030 double real; 00031 uint64_t base; 00032 } u_x; 00033 u_x.real = this->x; 00034 *(outbuffer + offset + 0) = (u_x.base >> (8 * 0)) & 0xFF; 00035 *(outbuffer + offset + 1) = (u_x.base >> (8 * 1)) & 0xFF; 00036 *(outbuffer + offset + 2) = (u_x.base >> (8 * 2)) & 0xFF; 00037 *(outbuffer + offset + 3) = (u_x.base >> (8 * 3)) & 0xFF; 00038 *(outbuffer + offset + 4) = (u_x.base >> (8 * 4)) & 0xFF; 00039 *(outbuffer + offset + 5) = (u_x.base >> (8 * 5)) & 0xFF; 00040 *(outbuffer + offset + 6) = (u_x.base >> (8 * 6)) & 0xFF; 00041 *(outbuffer + offset + 7) = (u_x.base >> (8 * 7)) & 0xFF; 00042 offset += sizeof(this->x); 00043 union { 00044 double real; 00045 uint64_t base; 00046 } u_y; 00047 u_y.real = this->y; 00048 *(outbuffer + offset + 0) = (u_y.base >> (8 * 0)) & 0xFF; 00049 *(outbuffer + offset + 1) = (u_y.base >> (8 * 1)) & 0xFF; 00050 *(outbuffer + offset + 2) = (u_y.base >> (8 * 2)) & 0xFF; 00051 *(outbuffer + offset + 3) = (u_y.base >> (8 * 3)) & 0xFF; 00052 *(outbuffer + offset + 4) = (u_y.base >> (8 * 4)) & 0xFF; 00053 *(outbuffer + offset + 5) = (u_y.base >> (8 * 5)) & 0xFF; 00054 *(outbuffer + offset + 6) = (u_y.base >> (8 * 6)) & 0xFF; 00055 *(outbuffer + offset + 7) = (u_y.base >> (8 * 7)) & 0xFF; 00056 offset += sizeof(this->y); 00057 union { 00058 double real; 00059 uint64_t base; 00060 } u_theta; 00061 u_theta.real = this->theta; 00062 *(outbuffer + offset + 0) = (u_theta.base >> (8 * 0)) & 0xFF; 00063 *(outbuffer + offset + 1) = (u_theta.base >> (8 * 1)) & 0xFF; 00064 *(outbuffer + offset + 2) = (u_theta.base >> (8 * 2)) & 0xFF; 00065 *(outbuffer + offset + 3) = (u_theta.base >> (8 * 3)) & 0xFF; 00066 *(outbuffer + offset + 4) = (u_theta.base >> (8 * 4)) & 0xFF; 00067 *(outbuffer + offset + 5) = (u_theta.base >> (8 * 5)) & 0xFF; 00068 *(outbuffer + offset + 6) = (u_theta.base >> (8 * 6)) & 0xFF; 00069 *(outbuffer + offset + 7) = (u_theta.base >> (8 * 7)) & 0xFF; 00070 offset += sizeof(this->theta); 00071 return offset; 00072 } 00073 00074 virtual int deserialize(unsigned char *inbuffer) 00075 { 00076 int offset = 0; 00077 union { 00078 double real; 00079 uint64_t base; 00080 } u_x; 00081 u_x.base = 0; 00082 u_x.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00083 u_x.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00084 u_x.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00085 u_x.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00086 u_x.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00087 u_x.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00088 u_x.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00089 u_x.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00090 this->x = u_x.real; 00091 offset += sizeof(this->x); 00092 union { 00093 double real; 00094 uint64_t base; 00095 } u_y; 00096 u_y.base = 0; 00097 u_y.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00098 u_y.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00099 u_y.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00100 u_y.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00101 u_y.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00102 u_y.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00103 u_y.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00104 u_y.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00105 this->y = u_y.real; 00106 offset += sizeof(this->y); 00107 union { 00108 double real; 00109 uint64_t base; 00110 } u_theta; 00111 u_theta.base = 0; 00112 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00113 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00114 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00115 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00116 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00117 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00118 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00119 u_theta.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00120 this->theta = u_theta.real; 00121 offset += sizeof(this->theta); 00122 return offset; 00123 } 00124 00125 const char * getType(){ return "geometry_msgs/Pose2D"; }; 00126 const char * getMD5(){ return "938fa65709584ad8e77d238529be13b8"; }; 00127 00128 }; 00129 00130 } 00131 #endif
Generated on Tue Jul 12 2022 18:39:40 by
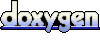