ROS Serial library for Mbed platforms for ROS Indigo Igloo. Check http://wiki.ros.org/rosserial_mbed/ for more information
Dependents: rosserial_mbed_hello_world_publisher rtos_base_control rosserial_mbed_F64MA ROS-RTOS ... more
ODEPhysics.h
00001 #ifndef _ROS_gazebo_msgs_ODEPhysics_h 00002 #define _ROS_gazebo_msgs_ODEPhysics_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace gazebo_msgs 00010 { 00011 00012 class ODEPhysics : public ros::Msg 00013 { 00014 public: 00015 bool auto_disable_bodies; 00016 uint32_t sor_pgs_precon_iters; 00017 uint32_t sor_pgs_iters; 00018 double sor_pgs_w; 00019 double sor_pgs_rms_error_tol; 00020 double contact_surface_layer; 00021 double contact_max_correcting_vel; 00022 double cfm; 00023 double erp; 00024 uint32_t max_contacts; 00025 00026 ODEPhysics(): 00027 auto_disable_bodies(0), 00028 sor_pgs_precon_iters(0), 00029 sor_pgs_iters(0), 00030 sor_pgs_w(0), 00031 sor_pgs_rms_error_tol(0), 00032 contact_surface_layer(0), 00033 contact_max_correcting_vel(0), 00034 cfm(0), 00035 erp(0), 00036 max_contacts(0) 00037 { 00038 } 00039 00040 virtual int serialize(unsigned char *outbuffer) const 00041 { 00042 int offset = 0; 00043 union { 00044 bool real; 00045 uint8_t base; 00046 } u_auto_disable_bodies; 00047 u_auto_disable_bodies.real = this->auto_disable_bodies; 00048 *(outbuffer + offset + 0) = (u_auto_disable_bodies.base >> (8 * 0)) & 0xFF; 00049 offset += sizeof(this->auto_disable_bodies); 00050 *(outbuffer + offset + 0) = (this->sor_pgs_precon_iters >> (8 * 0)) & 0xFF; 00051 *(outbuffer + offset + 1) = (this->sor_pgs_precon_iters >> (8 * 1)) & 0xFF; 00052 *(outbuffer + offset + 2) = (this->sor_pgs_precon_iters >> (8 * 2)) & 0xFF; 00053 *(outbuffer + offset + 3) = (this->sor_pgs_precon_iters >> (8 * 3)) & 0xFF; 00054 offset += sizeof(this->sor_pgs_precon_iters); 00055 *(outbuffer + offset + 0) = (this->sor_pgs_iters >> (8 * 0)) & 0xFF; 00056 *(outbuffer + offset + 1) = (this->sor_pgs_iters >> (8 * 1)) & 0xFF; 00057 *(outbuffer + offset + 2) = (this->sor_pgs_iters >> (8 * 2)) & 0xFF; 00058 *(outbuffer + offset + 3) = (this->sor_pgs_iters >> (8 * 3)) & 0xFF; 00059 offset += sizeof(this->sor_pgs_iters); 00060 union { 00061 double real; 00062 uint64_t base; 00063 } u_sor_pgs_w; 00064 u_sor_pgs_w.real = this->sor_pgs_w; 00065 *(outbuffer + offset + 0) = (u_sor_pgs_w.base >> (8 * 0)) & 0xFF; 00066 *(outbuffer + offset + 1) = (u_sor_pgs_w.base >> (8 * 1)) & 0xFF; 00067 *(outbuffer + offset + 2) = (u_sor_pgs_w.base >> (8 * 2)) & 0xFF; 00068 *(outbuffer + offset + 3) = (u_sor_pgs_w.base >> (8 * 3)) & 0xFF; 00069 *(outbuffer + offset + 4) = (u_sor_pgs_w.base >> (8 * 4)) & 0xFF; 00070 *(outbuffer + offset + 5) = (u_sor_pgs_w.base >> (8 * 5)) & 0xFF; 00071 *(outbuffer + offset + 6) = (u_sor_pgs_w.base >> (8 * 6)) & 0xFF; 00072 *(outbuffer + offset + 7) = (u_sor_pgs_w.base >> (8 * 7)) & 0xFF; 00073 offset += sizeof(this->sor_pgs_w); 00074 union { 00075 double real; 00076 uint64_t base; 00077 } u_sor_pgs_rms_error_tol; 00078 u_sor_pgs_rms_error_tol.real = this->sor_pgs_rms_error_tol; 00079 *(outbuffer + offset + 0) = (u_sor_pgs_rms_error_tol.base >> (8 * 0)) & 0xFF; 00080 *(outbuffer + offset + 1) = (u_sor_pgs_rms_error_tol.base >> (8 * 1)) & 0xFF; 00081 *(outbuffer + offset + 2) = (u_sor_pgs_rms_error_tol.base >> (8 * 2)) & 0xFF; 00082 *(outbuffer + offset + 3) = (u_sor_pgs_rms_error_tol.base >> (8 * 3)) & 0xFF; 00083 *(outbuffer + offset + 4) = (u_sor_pgs_rms_error_tol.base >> (8 * 4)) & 0xFF; 00084 *(outbuffer + offset + 5) = (u_sor_pgs_rms_error_tol.base >> (8 * 5)) & 0xFF; 00085 *(outbuffer + offset + 6) = (u_sor_pgs_rms_error_tol.base >> (8 * 6)) & 0xFF; 00086 *(outbuffer + offset + 7) = (u_sor_pgs_rms_error_tol.base >> (8 * 7)) & 0xFF; 00087 offset += sizeof(this->sor_pgs_rms_error_tol); 00088 union { 00089 double real; 00090 uint64_t base; 00091 } u_contact_surface_layer; 00092 u_contact_surface_layer.real = this->contact_surface_layer; 00093 *(outbuffer + offset + 0) = (u_contact_surface_layer.base >> (8 * 0)) & 0xFF; 00094 *(outbuffer + offset + 1) = (u_contact_surface_layer.base >> (8 * 1)) & 0xFF; 00095 *(outbuffer + offset + 2) = (u_contact_surface_layer.base >> (8 * 2)) & 0xFF; 00096 *(outbuffer + offset + 3) = (u_contact_surface_layer.base >> (8 * 3)) & 0xFF; 00097 *(outbuffer + offset + 4) = (u_contact_surface_layer.base >> (8 * 4)) & 0xFF; 00098 *(outbuffer + offset + 5) = (u_contact_surface_layer.base >> (8 * 5)) & 0xFF; 00099 *(outbuffer + offset + 6) = (u_contact_surface_layer.base >> (8 * 6)) & 0xFF; 00100 *(outbuffer + offset + 7) = (u_contact_surface_layer.base >> (8 * 7)) & 0xFF; 00101 offset += sizeof(this->contact_surface_layer); 00102 union { 00103 double real; 00104 uint64_t base; 00105 } u_contact_max_correcting_vel; 00106 u_contact_max_correcting_vel.real = this->contact_max_correcting_vel; 00107 *(outbuffer + offset + 0) = (u_contact_max_correcting_vel.base >> (8 * 0)) & 0xFF; 00108 *(outbuffer + offset + 1) = (u_contact_max_correcting_vel.base >> (8 * 1)) & 0xFF; 00109 *(outbuffer + offset + 2) = (u_contact_max_correcting_vel.base >> (8 * 2)) & 0xFF; 00110 *(outbuffer + offset + 3) = (u_contact_max_correcting_vel.base >> (8 * 3)) & 0xFF; 00111 *(outbuffer + offset + 4) = (u_contact_max_correcting_vel.base >> (8 * 4)) & 0xFF; 00112 *(outbuffer + offset + 5) = (u_contact_max_correcting_vel.base >> (8 * 5)) & 0xFF; 00113 *(outbuffer + offset + 6) = (u_contact_max_correcting_vel.base >> (8 * 6)) & 0xFF; 00114 *(outbuffer + offset + 7) = (u_contact_max_correcting_vel.base >> (8 * 7)) & 0xFF; 00115 offset += sizeof(this->contact_max_correcting_vel); 00116 union { 00117 double real; 00118 uint64_t base; 00119 } u_cfm; 00120 u_cfm.real = this->cfm; 00121 *(outbuffer + offset + 0) = (u_cfm.base >> (8 * 0)) & 0xFF; 00122 *(outbuffer + offset + 1) = (u_cfm.base >> (8 * 1)) & 0xFF; 00123 *(outbuffer + offset + 2) = (u_cfm.base >> (8 * 2)) & 0xFF; 00124 *(outbuffer + offset + 3) = (u_cfm.base >> (8 * 3)) & 0xFF; 00125 *(outbuffer + offset + 4) = (u_cfm.base >> (8 * 4)) & 0xFF; 00126 *(outbuffer + offset + 5) = (u_cfm.base >> (8 * 5)) & 0xFF; 00127 *(outbuffer + offset + 6) = (u_cfm.base >> (8 * 6)) & 0xFF; 00128 *(outbuffer + offset + 7) = (u_cfm.base >> (8 * 7)) & 0xFF; 00129 offset += sizeof(this->cfm); 00130 union { 00131 double real; 00132 uint64_t base; 00133 } u_erp; 00134 u_erp.real = this->erp; 00135 *(outbuffer + offset + 0) = (u_erp.base >> (8 * 0)) & 0xFF; 00136 *(outbuffer + offset + 1) = (u_erp.base >> (8 * 1)) & 0xFF; 00137 *(outbuffer + offset + 2) = (u_erp.base >> (8 * 2)) & 0xFF; 00138 *(outbuffer + offset + 3) = (u_erp.base >> (8 * 3)) & 0xFF; 00139 *(outbuffer + offset + 4) = (u_erp.base >> (8 * 4)) & 0xFF; 00140 *(outbuffer + offset + 5) = (u_erp.base >> (8 * 5)) & 0xFF; 00141 *(outbuffer + offset + 6) = (u_erp.base >> (8 * 6)) & 0xFF; 00142 *(outbuffer + offset + 7) = (u_erp.base >> (8 * 7)) & 0xFF; 00143 offset += sizeof(this->erp); 00144 *(outbuffer + offset + 0) = (this->max_contacts >> (8 * 0)) & 0xFF; 00145 *(outbuffer + offset + 1) = (this->max_contacts >> (8 * 1)) & 0xFF; 00146 *(outbuffer + offset + 2) = (this->max_contacts >> (8 * 2)) & 0xFF; 00147 *(outbuffer + offset + 3) = (this->max_contacts >> (8 * 3)) & 0xFF; 00148 offset += sizeof(this->max_contacts); 00149 return offset; 00150 } 00151 00152 virtual int deserialize(unsigned char *inbuffer) 00153 { 00154 int offset = 0; 00155 union { 00156 bool real; 00157 uint8_t base; 00158 } u_auto_disable_bodies; 00159 u_auto_disable_bodies.base = 0; 00160 u_auto_disable_bodies.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00161 this->auto_disable_bodies = u_auto_disable_bodies.real; 00162 offset += sizeof(this->auto_disable_bodies); 00163 this->sor_pgs_precon_iters = ((uint32_t) (*(inbuffer + offset))); 00164 this->sor_pgs_precon_iters |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00165 this->sor_pgs_precon_iters |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00166 this->sor_pgs_precon_iters |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00167 offset += sizeof(this->sor_pgs_precon_iters); 00168 this->sor_pgs_iters = ((uint32_t) (*(inbuffer + offset))); 00169 this->sor_pgs_iters |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00170 this->sor_pgs_iters |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00171 this->sor_pgs_iters |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00172 offset += sizeof(this->sor_pgs_iters); 00173 union { 00174 double real; 00175 uint64_t base; 00176 } u_sor_pgs_w; 00177 u_sor_pgs_w.base = 0; 00178 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00179 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00180 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00181 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00182 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00183 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00184 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00185 u_sor_pgs_w.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00186 this->sor_pgs_w = u_sor_pgs_w.real; 00187 offset += sizeof(this->sor_pgs_w); 00188 union { 00189 double real; 00190 uint64_t base; 00191 } u_sor_pgs_rms_error_tol; 00192 u_sor_pgs_rms_error_tol.base = 0; 00193 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00194 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00195 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00196 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00197 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00198 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00199 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00200 u_sor_pgs_rms_error_tol.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00201 this->sor_pgs_rms_error_tol = u_sor_pgs_rms_error_tol.real; 00202 offset += sizeof(this->sor_pgs_rms_error_tol); 00203 union { 00204 double real; 00205 uint64_t base; 00206 } u_contact_surface_layer; 00207 u_contact_surface_layer.base = 0; 00208 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00209 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00210 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00211 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00212 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00213 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00214 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00215 u_contact_surface_layer.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00216 this->contact_surface_layer = u_contact_surface_layer.real; 00217 offset += sizeof(this->contact_surface_layer); 00218 union { 00219 double real; 00220 uint64_t base; 00221 } u_contact_max_correcting_vel; 00222 u_contact_max_correcting_vel.base = 0; 00223 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00224 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00225 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00226 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00227 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00228 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00229 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00230 u_contact_max_correcting_vel.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00231 this->contact_max_correcting_vel = u_contact_max_correcting_vel.real; 00232 offset += sizeof(this->contact_max_correcting_vel); 00233 union { 00234 double real; 00235 uint64_t base; 00236 } u_cfm; 00237 u_cfm.base = 0; 00238 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00239 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00240 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00241 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00242 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00243 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00244 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00245 u_cfm.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00246 this->cfm = u_cfm.real; 00247 offset += sizeof(this->cfm); 00248 union { 00249 double real; 00250 uint64_t base; 00251 } u_erp; 00252 u_erp.base = 0; 00253 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00254 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00255 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00256 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00257 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00258 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00259 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00260 u_erp.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00261 this->erp = u_erp.real; 00262 offset += sizeof(this->erp); 00263 this->max_contacts = ((uint32_t) (*(inbuffer + offset))); 00264 this->max_contacts |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00265 this->max_contacts |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00266 this->max_contacts |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00267 offset += sizeof(this->max_contacts); 00268 return offset; 00269 } 00270 00271 const char * getType(){ return "gazebo_msgs/ODEPhysics"; }; 00272 const char * getMD5(){ return "667d56ddbd547918c32d1934503dc335"; }; 00273 00274 }; 00275 00276 } 00277 #endif
Generated on Tue Jul 12 2022 18:39:40 by
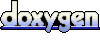