ROS Serial library for Mbed platforms for ROS Indigo Igloo. Check http://wiki.ros.org/rosserial_mbed/ for more information
Dependents: rosserial_mbed_hello_world_publisher rtos_base_control rosserial_mbed_F64MA ROS-RTOS ... more
NodeletUnload.h
00001 #ifndef _ROS_SERVICE_NodeletUnload_h 00002 #define _ROS_SERVICE_NodeletUnload_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 00008 namespace nodelet 00009 { 00010 00011 static const char NODELETUNLOAD[] = "nodelet/NodeletUnload"; 00012 00013 class NodeletUnloadRequest : public ros::Msg 00014 { 00015 public: 00016 const char* name; 00017 00018 NodeletUnloadRequest(): 00019 name("") 00020 { 00021 } 00022 00023 virtual int serialize(unsigned char *outbuffer) const 00024 { 00025 int offset = 0; 00026 uint32_t length_name = strlen(this->name); 00027 memcpy(outbuffer + offset, &length_name, sizeof(uint32_t)); 00028 offset += 4; 00029 memcpy(outbuffer + offset, this->name, length_name); 00030 offset += length_name; 00031 return offset; 00032 } 00033 00034 virtual int deserialize(unsigned char *inbuffer) 00035 { 00036 int offset = 0; 00037 uint32_t length_name; 00038 memcpy(&length_name, (inbuffer + offset), sizeof(uint32_t)); 00039 offset += 4; 00040 for(unsigned int k= offset; k< offset+length_name; ++k){ 00041 inbuffer[k-1]=inbuffer[k]; 00042 } 00043 inbuffer[offset+length_name-1]=0; 00044 this->name = (char *)(inbuffer + offset-1); 00045 offset += length_name; 00046 return offset; 00047 } 00048 00049 const char * getType(){ return NODELETUNLOAD; }; 00050 const char * getMD5(){ return "c1f3d28f1b044c871e6eff2e9fc3c667"; }; 00051 00052 }; 00053 00054 class NodeletUnloadResponse : public ros::Msg 00055 { 00056 public: 00057 bool success; 00058 00059 NodeletUnloadResponse(): 00060 success(0) 00061 { 00062 } 00063 00064 virtual int serialize(unsigned char *outbuffer) const 00065 { 00066 int offset = 0; 00067 union { 00068 bool real; 00069 uint8_t base; 00070 } u_success; 00071 u_success.real = this->success; 00072 *(outbuffer + offset + 0) = (u_success.base >> (8 * 0)) & 0xFF; 00073 offset += sizeof(this->success); 00074 return offset; 00075 } 00076 00077 virtual int deserialize(unsigned char *inbuffer) 00078 { 00079 int offset = 0; 00080 union { 00081 bool real; 00082 uint8_t base; 00083 } u_success; 00084 u_success.base = 0; 00085 u_success.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00086 this->success = u_success.real; 00087 offset += sizeof(this->success); 00088 return offset; 00089 } 00090 00091 const char * getType(){ return NODELETUNLOAD; }; 00092 const char * getMD5(){ return "358e233cde0c8a8bcfea4ce193f8fc15"; }; 00093 00094 }; 00095 00096 class NodeletUnload { 00097 public: 00098 typedef NodeletUnloadRequest Request; 00099 typedef NodeletUnloadResponse Response; 00100 }; 00101 00102 } 00103 #endif
Generated on Tue Jul 12 2022 18:39:40 by
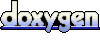