ROS Serial library for Mbed platforms for ROS Indigo Igloo. Check http://wiki.ros.org/rosserial_mbed/ for more information
Dependents: rosserial_mbed_hello_world_publisher rtos_base_control rosserial_mbed_F64MA ROS-RTOS ... more
GetJointProperties.h
00001 #ifndef _ROS_SERVICE_GetJointProperties_h 00002 #define _ROS_SERVICE_GetJointProperties_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 00008 namespace gazebo_msgs 00009 { 00010 00011 static const char GETJOINTPROPERTIES[] = "gazebo_msgs/GetJointProperties"; 00012 00013 class GetJointPropertiesRequest : public ros::Msg 00014 { 00015 public: 00016 const char* joint_name; 00017 00018 GetJointPropertiesRequest(): 00019 joint_name("") 00020 { 00021 } 00022 00023 virtual int serialize(unsigned char *outbuffer) const 00024 { 00025 int offset = 0; 00026 uint32_t length_joint_name = strlen(this->joint_name); 00027 memcpy(outbuffer + offset, &length_joint_name, sizeof(uint32_t)); 00028 offset += 4; 00029 memcpy(outbuffer + offset, this->joint_name, length_joint_name); 00030 offset += length_joint_name; 00031 return offset; 00032 } 00033 00034 virtual int deserialize(unsigned char *inbuffer) 00035 { 00036 int offset = 0; 00037 uint32_t length_joint_name; 00038 memcpy(&length_joint_name, (inbuffer + offset), sizeof(uint32_t)); 00039 offset += 4; 00040 for(unsigned int k= offset; k< offset+length_joint_name; ++k){ 00041 inbuffer[k-1]=inbuffer[k]; 00042 } 00043 inbuffer[offset+length_joint_name-1]=0; 00044 this->joint_name = (char *)(inbuffer + offset-1); 00045 offset += length_joint_name; 00046 return offset; 00047 } 00048 00049 const char * getType(){ return GETJOINTPROPERTIES; }; 00050 const char * getMD5(){ return "0be1351618e1dc030eb7959d9a4902de"; }; 00051 00052 }; 00053 00054 class GetJointPropertiesResponse : public ros::Msg 00055 { 00056 public: 00057 uint8_t type; 00058 uint8_t damping_length; 00059 double st_damping; 00060 double * damping; 00061 uint8_t position_length; 00062 double st_position; 00063 double * position; 00064 uint8_t rate_length; 00065 double st_rate; 00066 double * rate; 00067 bool success; 00068 const char* status_message; 00069 enum { REVOLUTE = 0 }; 00070 enum { CONTINUOUS = 1 }; 00071 enum { PRISMATIC = 2 }; 00072 enum { FIXED = 3 }; 00073 enum { BALL = 4 }; 00074 enum { UNIVERSAL = 5 }; 00075 00076 GetJointPropertiesResponse(): 00077 type(0), 00078 damping_length(0), damping(NULL), 00079 position_length(0), position(NULL), 00080 rate_length(0), rate(NULL), 00081 success(0), 00082 status_message("") 00083 { 00084 } 00085 00086 virtual int serialize(unsigned char *outbuffer) const 00087 { 00088 int offset = 0; 00089 *(outbuffer + offset + 0) = (this->type >> (8 * 0)) & 0xFF; 00090 offset += sizeof(this->type); 00091 *(outbuffer + offset++) = damping_length; 00092 *(outbuffer + offset++) = 0; 00093 *(outbuffer + offset++) = 0; 00094 *(outbuffer + offset++) = 0; 00095 for( uint8_t i = 0; i < damping_length; i++){ 00096 union { 00097 double real; 00098 uint64_t base; 00099 } u_dampingi; 00100 u_dampingi.real = this->damping[i]; 00101 *(outbuffer + offset + 0) = (u_dampingi.base >> (8 * 0)) & 0xFF; 00102 *(outbuffer + offset + 1) = (u_dampingi.base >> (8 * 1)) & 0xFF; 00103 *(outbuffer + offset + 2) = (u_dampingi.base >> (8 * 2)) & 0xFF; 00104 *(outbuffer + offset + 3) = (u_dampingi.base >> (8 * 3)) & 0xFF; 00105 *(outbuffer + offset + 4) = (u_dampingi.base >> (8 * 4)) & 0xFF; 00106 *(outbuffer + offset + 5) = (u_dampingi.base >> (8 * 5)) & 0xFF; 00107 *(outbuffer + offset + 6) = (u_dampingi.base >> (8 * 6)) & 0xFF; 00108 *(outbuffer + offset + 7) = (u_dampingi.base >> (8 * 7)) & 0xFF; 00109 offset += sizeof(this->damping[i]); 00110 } 00111 *(outbuffer + offset++) = position_length; 00112 *(outbuffer + offset++) = 0; 00113 *(outbuffer + offset++) = 0; 00114 *(outbuffer + offset++) = 0; 00115 for( uint8_t i = 0; i < position_length; i++){ 00116 union { 00117 double real; 00118 uint64_t base; 00119 } u_positioni; 00120 u_positioni.real = this->position[i]; 00121 *(outbuffer + offset + 0) = (u_positioni.base >> (8 * 0)) & 0xFF; 00122 *(outbuffer + offset + 1) = (u_positioni.base >> (8 * 1)) & 0xFF; 00123 *(outbuffer + offset + 2) = (u_positioni.base >> (8 * 2)) & 0xFF; 00124 *(outbuffer + offset + 3) = (u_positioni.base >> (8 * 3)) & 0xFF; 00125 *(outbuffer + offset + 4) = (u_positioni.base >> (8 * 4)) & 0xFF; 00126 *(outbuffer + offset + 5) = (u_positioni.base >> (8 * 5)) & 0xFF; 00127 *(outbuffer + offset + 6) = (u_positioni.base >> (8 * 6)) & 0xFF; 00128 *(outbuffer + offset + 7) = (u_positioni.base >> (8 * 7)) & 0xFF; 00129 offset += sizeof(this->position[i]); 00130 } 00131 *(outbuffer + offset++) = rate_length; 00132 *(outbuffer + offset++) = 0; 00133 *(outbuffer + offset++) = 0; 00134 *(outbuffer + offset++) = 0; 00135 for( uint8_t i = 0; i < rate_length; i++){ 00136 union { 00137 double real; 00138 uint64_t base; 00139 } u_ratei; 00140 u_ratei.real = this->rate[i]; 00141 *(outbuffer + offset + 0) = (u_ratei.base >> (8 * 0)) & 0xFF; 00142 *(outbuffer + offset + 1) = (u_ratei.base >> (8 * 1)) & 0xFF; 00143 *(outbuffer + offset + 2) = (u_ratei.base >> (8 * 2)) & 0xFF; 00144 *(outbuffer + offset + 3) = (u_ratei.base >> (8 * 3)) & 0xFF; 00145 *(outbuffer + offset + 4) = (u_ratei.base >> (8 * 4)) & 0xFF; 00146 *(outbuffer + offset + 5) = (u_ratei.base >> (8 * 5)) & 0xFF; 00147 *(outbuffer + offset + 6) = (u_ratei.base >> (8 * 6)) & 0xFF; 00148 *(outbuffer + offset + 7) = (u_ratei.base >> (8 * 7)) & 0xFF; 00149 offset += sizeof(this->rate[i]); 00150 } 00151 union { 00152 bool real; 00153 uint8_t base; 00154 } u_success; 00155 u_success.real = this->success; 00156 *(outbuffer + offset + 0) = (u_success.base >> (8 * 0)) & 0xFF; 00157 offset += sizeof(this->success); 00158 uint32_t length_status_message = strlen(this->status_message); 00159 memcpy(outbuffer + offset, &length_status_message, sizeof(uint32_t)); 00160 offset += 4; 00161 memcpy(outbuffer + offset, this->status_message, length_status_message); 00162 offset += length_status_message; 00163 return offset; 00164 } 00165 00166 virtual int deserialize(unsigned char *inbuffer) 00167 { 00168 int offset = 0; 00169 this->type = ((uint8_t) (*(inbuffer + offset))); 00170 offset += sizeof(this->type); 00171 uint8_t damping_lengthT = *(inbuffer + offset++); 00172 if(damping_lengthT > damping_length) 00173 this->damping = (double*)realloc(this->damping, damping_lengthT * sizeof(double)); 00174 offset += 3; 00175 damping_length = damping_lengthT; 00176 for( uint8_t i = 0; i < damping_length; i++){ 00177 union { 00178 double real; 00179 uint64_t base; 00180 } u_st_damping; 00181 u_st_damping.base = 0; 00182 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00183 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00184 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00185 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00186 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00187 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00188 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00189 u_st_damping.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00190 this->st_damping = u_st_damping.real; 00191 offset += sizeof(this->st_damping); 00192 memcpy( &(this->damping[i]), &(this->st_damping), sizeof(double)); 00193 } 00194 uint8_t position_lengthT = *(inbuffer + offset++); 00195 if(position_lengthT > position_length) 00196 this->position = (double*)realloc(this->position, position_lengthT * sizeof(double)); 00197 offset += 3; 00198 position_length = position_lengthT; 00199 for( uint8_t i = 0; i < position_length; i++){ 00200 union { 00201 double real; 00202 uint64_t base; 00203 } u_st_position; 00204 u_st_position.base = 0; 00205 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00206 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00207 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00208 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00209 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00210 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00211 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00212 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00213 this->st_position = u_st_position.real; 00214 offset += sizeof(this->st_position); 00215 memcpy( &(this->position[i]), &(this->st_position), sizeof(double)); 00216 } 00217 uint8_t rate_lengthT = *(inbuffer + offset++); 00218 if(rate_lengthT > rate_length) 00219 this->rate = (double*)realloc(this->rate, rate_lengthT * sizeof(double)); 00220 offset += 3; 00221 rate_length = rate_lengthT; 00222 for( uint8_t i = 0; i < rate_length; i++){ 00223 union { 00224 double real; 00225 uint64_t base; 00226 } u_st_rate; 00227 u_st_rate.base = 0; 00228 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00229 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00230 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00231 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00232 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00233 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00234 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00235 u_st_rate.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00236 this->st_rate = u_st_rate.real; 00237 offset += sizeof(this->st_rate); 00238 memcpy( &(this->rate[i]), &(this->st_rate), sizeof(double)); 00239 } 00240 union { 00241 bool real; 00242 uint8_t base; 00243 } u_success; 00244 u_success.base = 0; 00245 u_success.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00246 this->success = u_success.real; 00247 offset += sizeof(this->success); 00248 uint32_t length_status_message; 00249 memcpy(&length_status_message, (inbuffer + offset), sizeof(uint32_t)); 00250 offset += 4; 00251 for(unsigned int k= offset; k< offset+length_status_message; ++k){ 00252 inbuffer[k-1]=inbuffer[k]; 00253 } 00254 inbuffer[offset+length_status_message-1]=0; 00255 this->status_message = (char *)(inbuffer + offset-1); 00256 offset += length_status_message; 00257 return offset; 00258 } 00259 00260 const char * getType(){ return GETJOINTPROPERTIES; }; 00261 const char * getMD5(){ return "cd7b30a39faa372283dc94c5f6457f82"; }; 00262 00263 }; 00264 00265 class GetJointProperties { 00266 public: 00267 typedef GetJointPropertiesRequest Request; 00268 typedef GetJointPropertiesResponse Response; 00269 }; 00270 00271 } 00272 #endif
Generated on Tue Jul 12 2022 18:39:39 by
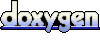