
Example od using MPU6050 library
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MPU6050.h" 00003 00004 DigitalOut myled(LED1); 00005 Serial pc(USBTX, USBRX); 00006 MPU6050 mpu; 00007 00008 int16_t ax, ay, az; 00009 int16_t gx, gy, gz; 00010 00011 int main() 00012 { 00013 pc.printf("MPU6050 test\n\n"); 00014 pc.printf("MPU6050 initialize \n"); 00015 00016 mpu.initialize(); 00017 pc.printf("MPU6050 testConnection \n"); 00018 00019 bool mpu6050TestResult = mpu.testConnection(); 00020 if(mpu6050TestResult) { 00021 pc.printf("MPU6050 test passed \n"); 00022 } else { 00023 pc.printf("MPU6050 test failed \n"); 00024 } 00025 00026 while(1) { 00027 wait(1); 00028 mpu.getMotion6(&ax, &ay, &az, &gx, &gy, &gz); 00029 //writing current accelerometer and gyro position 00030 pc.printf("%d;%d;%d;%d;%d;%d\n",ax,ay,az,gx,gy,gz); 00031 } 00032 }
Generated on Thu Jul 14 2022 08:31:11 by
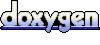