
testing TextLCD and SD card
Embed:
(wiki syntax)
Show/hide line numbers
SDFileSystem.h
00001 /* mbed Microcontroller Library - SDFileSystem 00002 * Copyright (c) 2008-2009, sford 00003 */ 00004 00005 #ifndef SDFILESYSTEM_H 00006 #define SDFILESYSTEM_H 00007 00008 #include "mbed.h" 00009 #include "FATFileSystem.h" 00010 00011 /* Class: SDFileSystem 00012 * Access the filesystem on an SD Card using SPI 00013 * 00014 * Example: 00015 * > SDFileSystem sd(p5, p6, p7, p12, "sd"); 00016 * > 00017 * > int main() { 00018 * > FILE *fp = fopen("/sd/myfile.txt", "w"); 00019 * > fprintf(fp, "Hello World!\n"); 00020 * > fclose(fp); 00021 * > } 00022 */ 00023 class SDFileSystem : public FATFileSystem { 00024 public: 00025 00026 /* Constructor: SDFileSystem 00027 * Create the File System for accessing an SD Card using SPI 00028 * 00029 * Variables: 00030 * mosi - SPI mosi pin connected to SD Card 00031 * miso - SPI miso pin conencted to SD Card 00032 * sclk - SPI sclk pin connected to SD Card 00033 * cs - DigitalOut pin used as SD Card chip select 00034 * name - The name used to access the filesystem 00035 */ 00036 SDFileSystem(PinName mosi, PinName miso, PinName sclk, PinName cs, const char* name); 00037 virtual int disk_initialize(); 00038 virtual int disk_write(const char *buffer, int block_number); 00039 virtual int disk_read(char *buffer, int block_number); 00040 virtual int disk_status(); 00041 virtual int disk_sync(); 00042 virtual int disk_sectors(); 00043 00044 protected: 00045 00046 int _cmd(int cmd, int arg); 00047 int _read(char *buffer, int length); 00048 int _write(const char *buffer, int length); 00049 int _sd_sectors(); 00050 int _sectors; 00051 00052 SPI _spi; 00053 DigitalOut _cs; 00054 }; 00055 00056 #endif
Generated on Thu Jul 21 2022 14:33:32 by
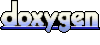