dualshock3
Embed:
(wiki syntax)
Show/hide line numbers
ds3_si.cpp
00001 #include "mbed.h" 00002 #include "ds3_si.h" 00003 00004 ds3_si::ds3_si(PinName tx, PinName rx, int baudrate):serial(tx, rx) 00005 { 00006 in[0] = 128; 00007 in[1] = 0; 00008 in[2] = 0; 00009 in[3] = 64; 00010 in[4] = 64; 00011 in[5] = 64; 00012 in[6] = 64; 00013 in[7] = 0; 00014 00015 serial.baud(baudrate); 00016 serial.attach(callback(this, &ds3_si::serialin), Serial::RxIrq); 00017 } 00018 00019 void ds3_si::serialin() 00020 { 00021 if(serial.readable()) 00022 { 00023 while(serial.getc() != 128) {} 00024 for(short i = 1; i < 8; i++) 00025 { 00026 *(in + i) = serial.getc(); 00027 } 00028 } 00029 } 00030 00031 bool ds3_si::buttonstate(short order) 00032 { 00033 return in[(order >> 7) + 1] & order; 00034 } 00035 00036 int ds3_si::analogstate(short order) 00037 { 00038 return (order % 2) ? in[order] - 64 : 64 - in[order]; 00039 } 00040 00041 int ds3_si::getinputdata(short order) 00042 { 00043 return in[order]; 00044 } 00045 00046 double ds3_si::getangle(short order) 00047 { 00048 int x = analogstate(order * 2 + 1); 00049 int y = analogstate(order * 2 + 2); 00050 if(x || y) return atan2(double(y), double(x)); 00051 else return NONE_angle; 00052 }
Generated on Wed Jul 13 2022 18:58:34 by
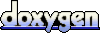