
acsip s76g
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Copyright (c) 2017, Arm Limited and affiliates. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include <stdio.h> 00018 #include "mbed.h" 00019 #include "lorawan/LoRaWANInterface.h" 00020 #include "lorawan/system/lorawan_data_structures.h" 00021 #include "events/EventQueue.h" 00022 #include "TinyGPSPlus.h" 00023 // Application helpers 00024 #include "trace_helper.h" 00025 #include "lora_radio_helper.h" 00026 00027 using namespace events; 00028 00029 // Max payload size can be LORAMAC_PHY_MAXPAYLOAD. 00030 // This example only communicates with much shorter messages (<30 bytes). 00031 // If longer messages are used, these buffers must be changed accordingly. 00032 uint8_t tx_buffer[30]; 00033 uint8_t rx_buffer[30]; 00034 char buf[128]; 00035 00036 char c; 00037 /* 00038 * Sets up an application dependent transmission timer in ms. Used only when Duty Cycling is off for testing 00039 */ 00040 #define TX_TIMER 10000 00041 00042 00043 00044 /** 00045 * Maximum number of events for the event queue. 00046 * 10 is the safe number for the stack events, however, if application 00047 * also uses the queue for whatever purposes, this number should be increased. 00048 */ 00049 #define MAX_NUMBER_OF_EVENTS 10 00050 00051 /** 00052 * Maximum number of retries for CONFIRMED messages before giving up 00053 */ 00054 #define CONFIRMED_MSG_RETRY_COUNTER 3 00055 00056 #define DATABUFFER_SIZE 128 00057 00058 00059 00060 00061 Serial gpsPort(PC_10,PC_11,115200); 00062 Serial pc(PA_9,PA_10); 00063 TinyGPSPlus gps; 00064 DigitalOut GPS_LEVEL_SHIFTER_EN(PC_6); 00065 DigitalOut GPS_RESET(PB_2); 00066 /** 00067 * This event queue is the global event queue for both the 00068 * application and stack. To conserve memory, the stack is designed to run 00069 * in the same thread as the application and the application is responsible for 00070 * providing an event queue to the stack that will be used for ISR deferment as 00071 * well as application information event queuing. 00072 */ 00073 static EventQueue ev_queue(MAX_NUMBER_OF_EVENTS *EVENTS_EVENT_SIZE); 00074 00075 /** 00076 * Event handler. 00077 * 00078 * This will be passed to the LoRaWAN stack to queue events for the 00079 * application which in turn drive the application. 00080 */ 00081 static void lora_event_handler(lorawan_event_t event); 00082 00083 /** 00084 * Constructing Mbed LoRaWANInterface and passing it the radio object from lora_radio_helper. 00085 */ 00086 static LoRaWANInterface lorawan(radio); 00087 00088 /** 00089 * Application specific callbacks 00090 */ 00091 static lorawan_app_callbacks_t callbacks; 00092 00093 void gpsSetup() 00094 { 00095 00096 GPS_LEVEL_SHIFTER_EN=1; 00097 wait(0.2); 00098 GPS_RESET=0; 00099 00100 wait(1); 00101 00102 GPS_RESET=1; 00103 00104 // wait(1); 00105 //gpsPort.printf("@GSR\r\n");//"@GNS 0x3\r\n" 00106 //wait(.25); 00107 //gpsPort.printf("@GNS 0x3\r\n"); 00108 //wait(.25); 00109 //gpsPort.printf("@BSSL 0x25\r\n"); 00110 //wait(.25); 00111 //gpsPort.printf("@GCD\r\n"); 00112 //wait(.25); 00113 } 00114 00115 void getGpsData() 00116 { 00117 00118 c= gpsPort.getc(); 00119 00120 //pc.putc(c); 00121 switch (c) { 00122 case '\n': 00123 if (gps.satellites.isValid() && gps.satellites.value() > 3 && gps.hdop.hdop() > 0) { 00124 snprintf(buf, 128, "{\"lat\":%lf,\"lng\":%lf}", gps.location.lat(), gps.location.lng()); 00125 } else { 00126 snprintf(buf, 128, "Satellites: %lu, time: %04d-%02d-%02dT%02d:%02d:%02d.%02d", 00127 gps.satellites.value(), gps.date.year(), gps.date.month(), gps.date.day(), 00128 gps.time.hour(), gps.time.minute(), gps.time.second(), gps.time.centisecond()); 00129 } 00130 pc.printf("%s\r\n", buf); 00131 break; 00132 default: 00133 gps.encode(c); 00134 break; 00135 } 00136 00137 ev_queue.call(getGpsData); 00138 } 00139 00140 00141 /** 00142 * Entry point for application 00143 */ 00144 int main(void) 00145 00146 { 00147 //getGpsData(); 00148 gpsSetup(); 00149 00150 00151 pc.printf("hello"); 00152 // setup tracing 00153 setup_trace(); 00154 00155 // stores the status of a call to LoRaWAN protocol 00156 lorawan_status_t retcode; 00157 00158 // Initialize LoRaWAN stack 00159 if (lorawan.initialize(&ev_queue) != LORAWAN_STATUS_OK) { 00160 pc.printf("\r\n LoRa initialization failed! \r\n"); 00161 return -1; 00162 } 00163 00164 pc.printf("\r\n Mbed LoRaWANStack initialized \r\n"); 00165 00166 // prepare application callbacks 00167 callbacks.events = mbed::callback(lora_event_handler); 00168 lorawan.add_app_callbacks(&callbacks); 00169 00170 // Set number of retries in case of CONFIRMED messages 00171 if (lorawan.set_confirmed_msg_retries(CONFIRMED_MSG_RETRY_COUNTER) 00172 != LORAWAN_STATUS_OK) { 00173 pc.printf("\r\n set_confirmed_msg_retries failed! \r\n\r\n"); 00174 return -1; 00175 } 00176 00177 pc.printf("\r\n CONFIRMED message retries : %d \r\n", 00178 CONFIRMED_MSG_RETRY_COUNTER); 00179 00180 // Enable adaptive data rate 00181 if (lorawan.enable_adaptive_datarate() != LORAWAN_STATUS_OK) { 00182 pc.printf("\r\n enable_adaptive_datarate failed! \r\n"); 00183 return -1; 00184 } 00185 00186 pc.printf("\r\n Adaptive data rate (ADR) - Enabled \r\n"); 00187 00188 retcode = lorawan.connect(); 00189 00190 if (retcode == LORAWAN_STATUS_OK || 00191 retcode == LORAWAN_STATUS_CONNECT_IN_PROGRESS) { 00192 } else { 00193 pc.printf("\r\n Connection error, code = %d \r\n", retcode); 00194 return -1; 00195 } 00196 00197 pc.printf("\r\n Connection - In Progress ...\r\n"); 00198 00199 00200 ev_queue.call(getGpsData); 00201 // make your event queue dispatching events forever 00202 ev_queue.dispatch_forever(); 00203 00204 return 0; 00205 } 00206 00207 /** 00208 * Sends a message to the Network Server 00209 */ 00210 static void send_message() 00211 { 00212 uint16_t packet_len; 00213 int16_t retcode; 00214 pc.printf("\n\rHello world"); 00215 00216 packet_len = sprintf((char *) tx_buffer, "Hello world"); 00217 00218 retcode = lorawan.send(MBED_CONF_LORA_APP_PORT, tx_buffer, packet_len, 00219 MSG_UNCONFIRMED_FLAG); 00220 00221 if (retcode < 0) { 00222 retcode == LORAWAN_STATUS_WOULD_BLOCK ? pc.printf("send - WOULD BLOCK\r\n") 00223 : pc.printf("\r\n send() - Error code %d \r\n", retcode); 00224 00225 if (retcode == LORAWAN_STATUS_WOULD_BLOCK) { 00226 //retry in 3 seconds 00227 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00228 ev_queue.call_in(3000, send_message); 00229 } 00230 } 00231 return; 00232 } 00233 00234 pc.printf("\r\n %d bytes scheduled for transmission \r\n", retcode); 00235 memset(tx_buffer, 0, sizeof(tx_buffer)); 00236 00237 } 00238 00239 /** 00240 * Event handler 00241 */ 00242 static void lora_event_handler(lorawan_event_t event) 00243 { 00244 switch (event) { 00245 case CONNECTED: 00246 pc.printf("\r\n Connection - Successful \r\n"); 00247 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00248 send_message(); 00249 } else { 00250 ev_queue.call_every(TX_TIMER, send_message); 00251 } 00252 00253 break; 00254 case DISCONNECTED: 00255 ev_queue.break_dispatch(); 00256 pc.printf("\r\n Disconnected Successfully \r\n"); 00257 break; 00258 case TX_DONE: 00259 pc.printf("\r\n Message Sent to Network Server \r\n"); 00260 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00261 send_message(); 00262 00263 } 00264 break; 00265 case TX_TIMEOUT: 00266 case TX_ERROR: 00267 case TX_CRYPTO_ERROR: 00268 case TX_SCHEDULING_ERROR: 00269 pc.printf("\r\n Transmission Error - EventCode = %d \r\n", event); 00270 // try again 00271 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00272 send_message(); 00273 } 00274 break; 00275 case RX_DONE: 00276 pc.printf("\r\n Received message from Network Server \r\n"); 00277 break; 00278 case RX_TIMEOUT: 00279 case RX_ERROR: 00280 pc.printf("\r\n Error in reception - Code = %d \r\n", event); 00281 break; 00282 case JOIN_FAILURE: 00283 pc.printf("\r\n OTAA Failed - Check Keys \r\n"); 00284 break; 00285 case UPLINK_REQUIRED: 00286 pc.printf("\r\n Uplink required by NS \r\n"); 00287 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00288 send_message(); 00289 } 00290 break; 00291 default: 00292 MBED_ASSERT("Unknown Event"); 00293 } 00294 } 00295 00296 // EOF
Generated on Thu Jul 21 2022 08:13:41 by
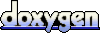