These are the examples provided for [[/users/frank26080115/libraries/LPC1700CMSIS_Lib/]] Note, the entire "program" is not compilable!
shell.c
00001 /* 00002 * Copyright (c) 2003, Adam Dunkels. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions 00007 * are met: 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote 00014 * products derived from this software without specific prior 00015 * written permission. 00016 * 00017 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS 00018 * OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00019 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00020 * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY 00021 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00022 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00023 * GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00025 * WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00026 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00027 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00028 * 00029 * This file is part of the uIP TCP/IP stack. 00030 * 00031 * $Id: shell.c,v 1.1 2006/06/07 09:43:54 adam Exp $ 00032 * 00033 */ 00034 00035 #include "shell.h" 00036 00037 #include <string.h> 00038 00039 struct ptentry { 00040 char *commandstr; 00041 void (* pfunc)(char *str); 00042 }; 00043 00044 #define SHELL_PROMPT "uIP 1.0> " 00045 00046 /*---------------------------------------------------------------------------*/ 00047 static void 00048 parse(register char *str, struct ptentry *t) 00049 { 00050 struct ptentry *p; 00051 for(p = t; p->commandstr != NULL; ++p) { 00052 if(strncmp(p->commandstr, str, strlen(p->commandstr)) == 0) { 00053 break; 00054 } 00055 } 00056 00057 p->pfunc(str); 00058 } 00059 /*---------------------------------------------------------------------------*/ 00060 static void 00061 inttostr(register char *str, unsigned int i) 00062 { 00063 str[0] = '0' + i / 100; 00064 if(str[0] == '0') { 00065 str[0] = ' '; 00066 } 00067 str[1] = '0' + (i / 10) % 10; 00068 if(str[0] == ' ' && str[1] == '0') { 00069 str[1] = ' '; 00070 } 00071 str[2] = '0' + i % 10; 00072 str[3] = ' '; 00073 str[4] = 0; 00074 } 00075 /*---------------------------------------------------------------------------*/ 00076 static void 00077 help(char *str) 00078 { 00079 shell_output("Available commands:", ""); 00080 shell_output("stats - show network statistics", ""); 00081 shell_output("conn - show TCP connections", ""); 00082 shell_output("help, ? - show help", ""); 00083 shell_output("exit - exit shell", ""); 00084 } 00085 /*---------------------------------------------------------------------------*/ 00086 static void 00087 unknown(char *str) 00088 { 00089 if(strlen(str) > 0) { 00090 shell_output("Unknown command: ", str); 00091 } 00092 } 00093 /*---------------------------------------------------------------------------*/ 00094 static struct ptentry parsetab[] = 00095 {{"stats", help}, 00096 {"conn", help}, 00097 {"help", help}, 00098 {"exit", shell_quit}, 00099 {"?", help}, 00100 00101 /* Default action */ 00102 {NULL, unknown}}; 00103 /*---------------------------------------------------------------------------*/ 00104 void 00105 shell_init(void) 00106 { 00107 } 00108 /*---------------------------------------------------------------------------*/ 00109 void 00110 shell_start(void) 00111 { 00112 shell_output("uIP command shell", ""); 00113 shell_output("Type '?' and return for help", ""); 00114 shell_prompt(SHELL_PROMPT); 00115 } 00116 /*---------------------------------------------------------------------------*/ 00117 void 00118 shell_input(char *cmd) 00119 { 00120 parse(cmd, parsetab); 00121 shell_prompt(SHELL_PROMPT); 00122 } 00123 /*---------------------------------------------------------------------------*/
Generated on Tue Jul 12 2022 17:28:09 by
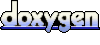