These are the examples provided for [[/users/frank26080115/libraries/LPC1700CMSIS_Lib/]] Note, the entire "program" is not compilable!
demo.c
00001 /*---------------------------------------------------------------------------- 00002 * Name: DEMO.C 00003 * Purpose: USB HID Demo 00004 * Version: V1.10 00005 *---------------------------------------------------------------------------- 00006 * This software is supplied "AS IS" without any warranties, express, 00007 * implied or statutory, including but not limited to the implied 00008 * warranties of fitness for purpose, satisfactory quality and 00009 * noninfringement. Keil extends you a royalty-free right to reproduce 00010 * and distribute executable files created using this software for use 00011 * on NXP Semiconductors LPC family microcontroller devices only. Nothing 00012 * else gives you the right to use this software. 00013 * 00014 * Copyright (c) 2005-2009 Keil Software. 00015 *---------------------------------------------------------------------------*/ 00016 00017 #include "LPC17xx.h" /* LPC17xx definitions */ 00018 00019 #include "lpc_types.h" 00020 00021 #include "usb.h" 00022 #include "usbcfg.h" 00023 #include "usbhw.h" 00024 00025 #include "demo.h" 00026 #include "hid.h" 00027 00028 #include "lpc17xx_libcfg.h" 00029 #include "lpc17xx_nvic.h" 00030 00031 /* Example group ----------------------------------------------------------- */ 00032 /** @defgroup USBDEV_USBHID USBHID 00033 * @ingroup USBDEV_Examples 00034 * @{ 00035 */ 00036 00037 00038 uint8_t InReport; /* HID Input Report */ 00039 /* Bit0 : Buttons */ 00040 /* Bit1..7: Reserved */ 00041 00042 uint8_t OutReport; /* HID Out Report */ 00043 /* Bit0..7: LEDs */ 00044 00045 00046 /* 00047 * Get HID Input Report -> InReport 00048 */ 00049 00050 void GetInReport (void) { 00051 00052 if ((LPC_GPIO2 -> FIOPIN & PBINT) == 0) { /* Check if PBINT is pressed */ 00053 InReport = 0x01; 00054 } else { 00055 InReport = 0x00; 00056 } 00057 } 00058 00059 00060 /* 00061 * Set HID Output Report <- OutReport 00062 */ 00063 00064 void SetOutReport (void) { 00065 //Because 8 LEDs are not ordered, so we have check each bit 00066 //of OurReport to turn on/off LED correctly 00067 uint8_t led_num; 00068 LPC_GPIO2 -> FIOCLR = LEDMSK; 00069 LPC_GPIO1 -> FIOCLR = 0xF0000000; 00070 //LED0 (P2.6) 00071 led_num = OutReport & (1<<0); 00072 if(led_num == 0) 00073 LPC_GPIO2 -> FIOCLR |= (1<<6); 00074 else 00075 LPC_GPIO2 -> FIOSET |= (1<<6); 00076 //LED1 (P2.5) 00077 led_num = OutReport & (1<<1); 00078 if(led_num == 0) 00079 LPC_GPIO2 -> FIOCLR |= (1<<5); 00080 else 00081 LPC_GPIO2 -> FIOSET |= (1<<5); 00082 //LED2 (P2.4) 00083 led_num = OutReport & (1<<2); 00084 if(led_num == 0) 00085 LPC_GPIO2 -> FIOCLR |= (1<<4); 00086 else 00087 LPC_GPIO2 -> FIOSET |= (1<<4); 00088 //LED3 (P2.3) 00089 led_num = OutReport & (1<<3); 00090 if(led_num == 0) 00091 LPC_GPIO2 -> FIOCLR |= (1<<3); 00092 else 00093 LPC_GPIO2 -> FIOSET |= (1<<3); 00094 //LED4 (P2.2) 00095 led_num = OutReport & (1<<4); 00096 if(led_num == 0) 00097 LPC_GPIO2 -> FIOCLR |= (1<<2); 00098 else 00099 LPC_GPIO2 -> FIOSET |= (1<<2); 00100 //LED5 (P1.31) 00101 led_num = OutReport & (1<<5); 00102 if(led_num == 0) 00103 LPC_GPIO1 -> FIOCLR |= (1<<31); 00104 else 00105 LPC_GPIO1 -> FIOSET |= (1<<31); 00106 //LED6 (P1.29) 00107 led_num = OutReport & (1<<6); 00108 if(led_num == 0) 00109 LPC_GPIO1 -> FIOCLR |= (1<<29); 00110 else 00111 LPC_GPIO1 -> FIOSET |= (1<<29); 00112 //LED7 (P1.28) 00113 led_num = OutReport & (1<<7); 00114 if(led_num == 0) 00115 LPC_GPIO1 -> FIOCLR |= (1<<28); 00116 else 00117 LPC_GPIO1 -> FIOSET |= (1<<28); 00118 } 00119 00120 00121 /* Main Program */ 00122 00123 int main (void) { 00124 LPC_GPIO2 -> FIODIR = LEDMSK; /* LEDs, port 2, bit 0~7 output only */ 00125 LPC_GPIO1 -> FIODIR = 0xF0000000; /* LEDs, port 1, bit 28-31 output */ 00126 00127 USB_Init(); /* USB Initialization */ 00128 USB_Connect(TRUE); /* USB Connect */ 00129 00130 while (1); /* Loop forever */ 00131 } 00132 00133 #ifdef DEBUG 00134 /******************************************************************************* 00135 * @brief Reports the name of the source file and the source line number 00136 * where the CHECK_PARAM error has occurred. 00137 * @param[in] file Pointer to the source file name 00138 * @param[in] line assert_param error line source number 00139 * @return None 00140 *******************************************************************************/ 00141 void check_failed(uint8_t *file, uint32_t line) 00142 { 00143 /* User can add his own implementation to report the file name and line number, 00144 ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */ 00145 00146 /* Infinite loop */ 00147 while(1); 00148 } 00149 #endif 00150 00151 /* 00152 * @} 00153 */ 00154
Generated on Tue Jul 12 2022 17:28:08 by
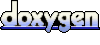