These are the examples provided for [[/users/frank26080115/libraries/LPC1700CMSIS_Lib/]] Note, the entire "program" is not compilable!
dac_dma.c
00001 /***********************************************************************//** 00002 * @file dac_dma.c 00003 * @purpose This example describes how to use DAC conversion and 00004 * using DMA to transfer data 00005 * @version 2.0 00006 * @date 21. May. 2010 00007 * @author NXP MCU SW Application Team 00008 *--------------------------------------------------------------------- 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **********************************************************************/ 00020 #include "lpc17xx_dac.h" 00021 #include "lpc17xx_libcfg.h" 00022 #include "lpc17xx_pinsel.h" 00023 #include "lpc17xx_gpdma.h" 00024 00025 /* Example group ----------------------------------------------------------- */ 00026 /** @defgroup DAC_DMA DMA 00027 * @ingroup DAC_Examples 00028 * @{ 00029 */ 00030 00031 /************************** PRIVATE MACROS *************************/ 00032 /** DMA size of transfer */ 00033 #define DMA_SIZE 1 00034 00035 /************************** PRIVATE VARIABLES *************************/ 00036 // Terminal Counter flag for Channel 0 00037 __IO uint32_t Channel0_TC; 00038 00039 // Error Counter flag for Channel 0 00040 __IO uint32_t Channel0_Err; 00041 00042 GPDMA_Channel_CFG_Type GPDMACfg; 00043 00044 /************************** PRIVATE FUNCTION *************************/ 00045 void DMA_IRQHandler (void); 00046 00047 00048 /*----------------- INTERRUPT SERVICE ROUTINES --------------------------*/ 00049 /*********************************************************************//** 00050 * @brief GPDMA interrupt handler sub-routine 00051 * @param[in] None 00052 * @return None 00053 **********************************************************************/ 00054 void DMA_IRQHandler (void) 00055 { 00056 // check GPDMA interrupt on channel 0 00057 if (GPDMA_IntGetStatus(GPDMA_STAT_INT, 0)){ 00058 // Check counter terminal status 00059 if(GPDMA_IntGetStatus(GPDMA_STAT_INTTC, 0)){ 00060 GPDMA_ClearIntPending (GPDMA_STATCLR_INTTC, 0); 00061 Channel0_TC++; 00062 } 00063 // Check error terminal status 00064 if (GPDMA_IntGetStatus(GPDMA_STAT_INTERR, 0)){ 00065 GPDMA_ClearIntPending (GPDMA_STATCLR_INTERR, 0); 00066 Channel0_Err++; 00067 } 00068 } 00069 } 00070 00071 00072 /*-------------------------MAIN FUNCTION------------------------------*/ 00073 /*********************************************************************//** 00074 * @brief c_entry: Main DAC program body 00075 * @param[in] None 00076 * @return int 00077 **********************************************************************/ 00078 int c_entry(void) 00079 { 00080 PINSEL_CFG_Type PinCfg; 00081 DAC_CONVERTER_CFG_Type DAC_ConverterConfigStruct; 00082 uint32_t dac_value =0; 00083 uint32_t i; 00084 /* 00085 * Init DAC pin connect 00086 * AOUT on P0.26 00087 */ 00088 PinCfg.Funcnum = 2; 00089 PinCfg.OpenDrain = 0; 00090 PinCfg.Pinmode = 0; 00091 PinCfg.Pinnum = 26; 00092 PinCfg.Portnum = 0; 00093 PINSEL_ConfigPin(&PinCfg); 00094 00095 /* GPDMA block section -------------------------------------------- */ 00096 00097 /* Disable GPDMA interrupt */ 00098 NVIC_DisableIRQ(DMA_IRQn); 00099 00100 /* preemption = 1, sub-priority = 1 */ 00101 NVIC_SetPriority(DMA_IRQn, ((0x01<<3)|0x01)); 00102 00103 DAC_ConverterConfigStruct.CNT_ENA =SET; 00104 DAC_ConverterConfigStruct.DMA_ENA = SET; 00105 DAC_Init(LPC_DAC); 00106 /* set time out for DAC*/ 00107 DAC_SetDMATimeOut(LPC_DAC,0xFFFF); 00108 DAC_ConfigDAConverterControl(LPC_DAC, &DAC_ConverterConfigStruct); 00109 00110 /* Initialize GPDMA controller */ 00111 GPDMA_Init(); 00112 00113 // Setup GPDMA channel -------------------------------- 00114 // channel 0 00115 GPDMACfg.ChannelNum = 0; 00116 // Source memory 00117 GPDMACfg.SrcMemAddr = (uint32_t)(&dac_value); 00118 // Destination memory - unused 00119 GPDMACfg.DstMemAddr = 0; 00120 // Transfer size 00121 GPDMACfg.TransferSize = DMA_SIZE; 00122 // Transfer width - unused 00123 GPDMACfg.TransferWidth = 0; 00124 // Transfer type 00125 GPDMACfg.TransferType = GPDMA_TRANSFERTYPE_M2P; 00126 // Source connection - unused 00127 GPDMACfg.SrcConn = 0; 00128 // Destination connection 00129 GPDMACfg.DstConn = GPDMA_CONN_DAC; 00130 // Linker List Item - unused 00131 GPDMACfg.DMALLI = 0; 00132 // Setup channel with given parameter 00133 GPDMA_Setup(&GPDMACfg); 00134 00135 /* Reset terminal counter */ 00136 Channel0_TC = 0; 00137 /* Reset Error counter */ 00138 Channel0_Err = 0; 00139 00140 /* Enable GPDMA interrupt */ 00141 NVIC_EnableIRQ(DMA_IRQn); 00142 00143 /* Wait for GPDMA processing complete */ 00144 while (1) { 00145 00146 // Enable GPDMA channel 0 00147 GPDMA_ChannelCmd(0, ENABLE); 00148 00149 while ((Channel0_TC == 0) ); 00150 00151 // Disable GPDMA channel 0 00152 GPDMA_ChannelCmd(0, DISABLE); 00153 00154 dac_value ++; 00155 if (dac_value == 0x3FF) dac_value =0; 00156 //delay 00157 for(i=0;i<100000;i++); 00158 00159 /* Reset terminal counter */ 00160 Channel0_TC = 0; 00161 00162 // Re-setup channel 00163 GPDMA_Setup(&GPDMACfg); 00164 } 00165 00166 return 1; 00167 } 00168 /* With ARM and GHS toolsets, the entry point is main() - this will 00169 allow the linker to generate wrapper code to setup stacks, allocate 00170 heap area, and initialize and copy code and data segments. For GNU 00171 toolsets, the entry point is through __start() in the crt0_gnu.asm 00172 file, and that startup code will setup stacks and data */ 00173 int main(void) 00174 { 00175 return c_entry(); 00176 } 00177 00178 #ifdef DEBUG 00179 /******************************************************************************* 00180 * @brief Reports the name of the source file and the source line number 00181 * where the CHECK_PARAM error has occurred. 00182 * @param[in] file Pointer to the source file name 00183 * @param[in] line assert_param error line source number 00184 * @return None 00185 *******************************************************************************/ 00186 void check_failed(uint8_t *file, uint32_t line) 00187 { 00188 /* User can add his own implementation to report the file name and line number, 00189 ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */ 00190 00191 /* Infinite loop */ 00192 while(1); 00193 } 00194 #endif 00195 00196 /* 00197 * @} 00198 */
Generated on Tue Jul 12 2022 17:28:08 by
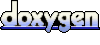