These are the examples provided for [[/users/frank26080115/libraries/LPC1700CMSIS_Lib/]] Note, the entire "program" is not compilable!
usbuser.c
00001 /*---------------------------------------------------------------------------- 00002 * U S B - K e r n e l 00003 *---------------------------------------------------------------------------- 00004 * Name: usbuser.c 00005 * Purpose: USB Custom User Module 00006 * Version: V1.20 00007 *---------------------------------------------------------------------------- 00008 * This software is supplied "AS IS" without any warranties, express, 00009 * implied or statutory, including but not limited to the implied 00010 * warranties of fitness for purpose, satisfactory quality and 00011 * noninfringement. Keil extends you a royalty-free right to reproduce 00012 * and distribute executable files created using this software for use 00013 * on NXP Semiconductors LPC family microcontroller devices only. Nothing 00014 * else gives you the right to use this software. 00015 * 00016 * Copyright (c) 2009 Keil - An ARM Company. All rights reserved. 00017 *---------------------------------------------------------------------------*/ 00018 #include "lpc_types.h" 00019 00020 #include "usb.h" 00021 #include "usbcfg.h" 00022 #include "usbhw.h" 00023 #include "usbcore.h" 00024 #include "usbuser.h" 00025 #include "cdcuser.h" 00026 00027 00028 /* 00029 * USB Power Event Callback 00030 * Called automatically on USB Power Event 00031 * Parameter: power: On(TRUE)/Off(FALSE) 00032 */ 00033 00034 #if USB_POWER_EVENT 00035 void USB_Power_Event (uint32_t power) { 00036 } 00037 #endif 00038 00039 00040 /* 00041 * USB Reset Event Callback 00042 * Called automatically on USB Reset Event 00043 */ 00044 00045 #if USB_RESET_EVENT 00046 void USB_Reset_Event (void) { 00047 USB_ResetCore(); 00048 } 00049 #endif 00050 00051 00052 /* 00053 * USB Suspend Event Callback 00054 * Called automatically on USB Suspend Event 00055 */ 00056 00057 #if USB_SUSPEND_EVENT 00058 void USB_Suspend_Event (void) { 00059 } 00060 #endif 00061 00062 00063 /* 00064 * USB Resume Event Callback 00065 * Called automatically on USB Resume Event 00066 */ 00067 00068 #if USB_RESUME_EVENT 00069 void USB_Resume_Event (void) { 00070 } 00071 #endif 00072 00073 00074 /* 00075 * USB Remote Wakeup Event Callback 00076 * Called automatically on USB Remote Wakeup Event 00077 */ 00078 00079 #if USB_WAKEUP_EVENT 00080 void USB_WakeUp_Event (void) { 00081 } 00082 #endif 00083 00084 00085 /* 00086 * USB Start of Frame Event Callback 00087 * Called automatically on USB Start of Frame Event 00088 */ 00089 00090 #if USB_SOF_EVENT 00091 void USB_SOF_Event (void) { 00092 } 00093 #endif 00094 00095 00096 /* 00097 * USB Error Event Callback 00098 * Called automatically on USB Error Event 00099 * Parameter: error: Error Code 00100 */ 00101 00102 #if USB_ERROR_EVENT 00103 void USB_Error_Event (uint32_t error) { 00104 } 00105 #endif 00106 00107 00108 /* 00109 * USB Set Configuration Event Callback 00110 * Called automatically on USB Set Configuration Request 00111 */ 00112 00113 #if USB_CONFIGURE_EVENT 00114 void USB_Configure_Event (void) { 00115 00116 if (USB_Configuration) { /* Check if USB is configured */ 00117 /* add your code here */ 00118 } 00119 } 00120 #endif 00121 00122 00123 /* 00124 * USB Set Interface Event Callback 00125 * Called automatically on USB Set Interface Request 00126 */ 00127 00128 #if USB_INTERFACE_EVENT 00129 void USB_Interface_Event (void) { 00130 } 00131 #endif 00132 00133 00134 /* 00135 * USB Set/Clear Feature Event Callback 00136 * Called automatically on USB Set/Clear Feature Request 00137 */ 00138 00139 #if USB_FEATURE_EVENT 00140 void USB_Feature_Event (void) { 00141 } 00142 #endif 00143 00144 00145 #define P_EP(n) ((USB_EP_EVENT & (1 << (n))) ? USB_EndPoint##n : NULL) 00146 00147 /* USB Endpoint Events Callback Pointers */ 00148 void (* const USB_P_EP[16]) (uint32_t event) = { 00149 P_EP(0), 00150 P_EP(1), 00151 P_EP(2), 00152 P_EP(3), 00153 P_EP(4), 00154 P_EP(5), 00155 P_EP(6), 00156 P_EP(7), 00157 P_EP(8), 00158 P_EP(9), 00159 P_EP(10), 00160 P_EP(11), 00161 P_EP(12), 00162 P_EP(13), 00163 P_EP(14), 00164 P_EP(15), 00165 }; 00166 00167 00168 /* 00169 * USB Endpoint 1 Event Callback 00170 * Called automatically on USB Endpoint 1 Event 00171 * Parameter: event 00172 */ 00173 00174 void USB_EndPoint1 (uint32_t event) { 00175 uint16_t temp; 00176 static uint16_t serialState; 00177 00178 switch (event) { 00179 case USB_EVT_IN: 00180 temp = CDC_GetSerialState(); 00181 if (serialState != temp) { 00182 serialState = temp; 00183 CDC_NotificationIn(); /* send SERIAL_STATE notification */ 00184 } 00185 break; 00186 } 00187 } 00188 00189 00190 /* 00191 * USB Endpoint 2 Event Callback 00192 * Called automatically on USB Endpoint 2 Event 00193 * Parameter: event 00194 */ 00195 00196 void USB_EndPoint2 (uint32_t event) { 00197 00198 switch (event) { 00199 case USB_EVT_OUT: 00200 CDC_BulkOut (); /* data received from Host */ 00201 break; 00202 case USB_EVT_IN: 00203 CDC_BulkIn (); /* data expected from Host */ 00204 break; 00205 } 00206 } 00207 00208 00209 /* 00210 * USB Endpoint 3 Event Callback 00211 * Called automatically on USB Endpoint 3 Event 00212 * Parameter: event 00213 */ 00214 00215 void USB_EndPoint3 (uint32_t event) { 00216 } 00217 00218 00219 /* 00220 * USB Endpoint 4 Event Callback 00221 * Called automatically on USB Endpoint 4 Event 00222 * Parameter: event 00223 */ 00224 00225 void USB_EndPoint4 (uint32_t event) { 00226 } 00227 00228 00229 /* 00230 * USB Endpoint 5 Event Callback 00231 * Called automatically on USB Endpoint 5 Event 00232 * Parameter: event 00233 */ 00234 00235 void USB_EndPoint5 (uint32_t event) { 00236 } 00237 00238 00239 /* 00240 * USB Endpoint 6 Event Callback 00241 * Called automatically on USB Endpoint 6 Event 00242 * Parameter: event 00243 */ 00244 00245 void USB_EndPoint6 (uint32_t event) { 00246 } 00247 00248 00249 /* 00250 * USB Endpoint 7 Event Callback 00251 * Called automatically on USB Endpoint 7 Event 00252 * Parameter: event 00253 */ 00254 00255 void USB_EndPoint7 (uint32_t event) { 00256 } 00257 00258 00259 /* 00260 * USB Endpoint 8 Event Callback 00261 * Called automatically on USB Endpoint 8 Event 00262 * Parameter: event 00263 */ 00264 00265 void USB_EndPoint8 (uint32_t event) { 00266 } 00267 00268 00269 /* 00270 * USB Endpoint 9 Event Callback 00271 * Called automatically on USB Endpoint 9 Event 00272 * Parameter: event 00273 */ 00274 00275 void USB_EndPoint9 (uint32_t event) { 00276 } 00277 00278 00279 /* 00280 * USB Endpoint 10 Event Callback 00281 * Called automatically on USB Endpoint 10 Event 00282 * Parameter: event 00283 */ 00284 00285 void USB_EndPoint10 (uint32_t event) { 00286 } 00287 00288 00289 /* 00290 * USB Endpoint 11 Event Callback 00291 * Called automatically on USB Endpoint 11 Event 00292 * Parameter: event 00293 */ 00294 00295 void USB_EndPoint11 (uint32_t event) { 00296 } 00297 00298 00299 /* 00300 * USB Endpoint 12 Event Callback 00301 * Called automatically on USB Endpoint 12 Event 00302 * Parameter: event 00303 */ 00304 00305 void USB_EndPoint12 (uint32_t event) { 00306 } 00307 00308 00309 /* 00310 * USB Endpoint 13 Event Callback 00311 * Called automatically on USB Endpoint 13 Event 00312 * Parameter: event 00313 */ 00314 00315 void USB_EndPoint13 (uint32_t event) { 00316 } 00317 00318 00319 /* 00320 * USB Endpoint 14 Event Callback 00321 * Called automatically on USB Endpoint 14 Event 00322 * Parameter: event 00323 */ 00324 00325 void USB_EndPoint14 (uint32_t event) { 00326 } 00327 00328 00329 /* 00330 * USB Endpoint 15 Event Callback 00331 * Called automatically on USB Endpoint 15 Event 00332 * Parameter: event 00333 */ 00334 00335 void USB_EndPoint15 (uint32_t event) { 00336 }
Generated on Tue Jul 12 2022 17:28:10 by
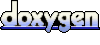