Libraries to support working with GMLAN - General Motors CAN BUS network in most of their vehicles between 2007-present day. Please note this is a work in progress and not guaranteed to be correct, use at your own risk! Read commit logs / subscribe to see what has been added, it's a work in progress after all ;)
GMLAN_11bit.h
00001 /* 00002 GMLAN_11bit.h - 11-Bit specific header file for GMLAN Library 00003 00004 GMLAN is a Controller Area Network Bus used in General Motors vehicles from 00005 roughly 2007-onwards. Its purpose is to allow various Electronic Control Units 00006 (aka ECUs) within a modern vehicle to share information and enact procedures. 00007 00008 An example of this would be communication between the HU (Head unit) and the 00009 DIC (Dashboard Information Cluster), when you adjust the volume up / down, this 00010 is reported to the cluster to be displayed. 00011 00012 It is the function of this library to "crack open" this world to allow anyone 00013 with only as little as a few hours of C++ programming under their belt to get 00014 started in what can sometimes seem a daunting world. 00015 00016 Jason Gaunt, 18th Feb 2013 00017 */ 00018 00019 #ifndef GMLAN_11BIT_H 00020 #define GMLAN_11BIT_H 00021 00022 // CAN IDs 00023 #define GMLAN_INITIAL_WAKE_UP_REQUEST 0x100 00024 #define GMLAN_REQUEST_TO_ALL_NODES 0x101 00025 #define GMLAN_DIAGNOSTIC_REQUEST 0x102 00026 #define GMLAN_TO_RESERVED_REQUEST 0x240 00027 #define GMLAN_TO_BCM 0x241 00028 #define GMLAN_TO_TDM 0x242 00029 #define GMLAN_TO_EBCM 0x243 00030 #define GMLAN_TO_EHU 0x244 00031 #define GMLAN_TO_SIC 0x246 00032 #define GMLAN_TO_SDC 0x247 00033 #define GMLAN_TO_IPC 0x24C 00034 #define GMLAN_TO_HVAC 0x251 00035 #define GMLAN_TO_RFA 0x258 00036 #define GMLAN_SF_FROM_RESERVED_RESPONSE 0x540 00037 #define GMLAN_MF_FROM_RESERVED_RESPONSE 0x640 00038 #define GMLAN_MF_FROM_BCM 0x641 00039 #define GMLAN_MF_FROM_TDM 0x642 00040 #define GMLAN_MF_FROM_EBCM 0x643 00041 #define GMLAN_MF_FROM_EHU 0x644 00042 #define GMLAN_MF_FROM_SIC 0x646 00043 #define GMLAN_MF_FROM_SDC 0x647 00044 #define GMLAN_MF_FROM_IPC 0x64C 00045 #define GMLAN_MF_FROM_HVAC 0x651 00046 #define GMLAN_MF_FROM_RFA 0x658 00047 #define GMLAN_EXTERNAL_OBD_TEST_EQUIPMENT_TO_NON_SPECIFIC_OBD_COMPLIANT_ECUS 0x7DF 00048 #define GMLAN_EXTERNAL_OBD_TEST_EQUIPMENT_TO_ECM 0x7E0 00049 #define GMLAN_EXTERNAL_OBD_TEST_EQUIPMENT_TO_SPECIFIC_OBD_COMPLIANT_ECU 0x7E1 00050 #define GMLAN_ECM_TO_EXTERNAL_OBD_TEST_EQUIPMENT 0x7E8 00051 #define GMLAN_SPECIFIC_OBD_COMPLIANT_ECU_TO_EXTERNAL_OBD_TEST_EQUIPMENT 0x7E9 00052 00053 // PCI byte 00054 #define GMLAN_PCI_UNSEGMENTED 0x0 00055 #define GMLAN_PCI_SEGMENTED 0x1 00056 #define GMLAN_PCI_ADDITIONAL 0x2 00057 #define GMLAN_PCI_FLOW_CONTROL 0x3 00058 00059 // Service ID byte 00060 #define GMLAN_SID_CLEAR_DTC 0x4 00061 #define GMLAN_SID_START_DIAG 0x10 00062 #define GMLAN_SID_REQ_FAIL_RECS 0x12 00063 #define GMLAN_SID_REQ_DID 0x1A 00064 #define GMLAN_SID_RES_NORM_OP 0x20 00065 #define GMLAN_SID_REQ_PID 0x22 00066 #define GMLAN_SID_READ_ADDR 0x23 00067 #define GMLAN_SID_REQ_SEC_ACCESS 0x27 00068 #define GMLAN_SID_DSBL_NORM_OP 0x28 00069 #define GMLAN_SID_DEF_DPID_MSG 0x2C 00070 #define GMLAN_SID_DEF_PID_BY_ADDR 0x2D 00071 #define GMLAN_SID_DL_REQ 0x34 00072 #define GMLAN_SID_DATA_TRANS 0x36 00073 #define GMLAN_SID_WRITE_DID 0x3B 00074 #define GMLAN_SID_TESTER_PRESENT 0x3E 00075 #define GMLAN_SID_ERROR 0x7F 00076 #define GMLAN_SID_REQ_PROG_STATE 0xA2 00077 #define GMLAN_SID_PROG_MODE 0xA5 00078 #define GMLAN_SID_READ_DTC 0xA9 00079 #define GMLAN_SID_REQ_DPID 0xAA 00080 #define GMLAN_SID_REQ_CONTROL 0xAE 00081 00082 // States of request 00083 #define GMLAN_STATE_READY_TO_SEND 0x0 00084 #define GMLAN_STATE_SEND_DATA 0x1 00085 #define GMLAN_STATE_AWAITING_FC 0x2 00086 #define GMLAN_STATE_AWAITING_REPLY 0x3 00087 #define GMLAN_STATE_SEND_FC 0x4 00088 #define GMLAN_STATE_COMPLETED 0x5 00089 #define GMLAN_STATE_ERROR 0x6 00090 00091 #endif
Generated on Tue Jul 12 2022 23:00:10 by
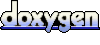