Libraries to support working with GMLAN - General Motors CAN BUS network in most of their vehicles between 2007-present day. Please note this is a work in progress and not guaranteed to be correct, use at your own risk! Read commit logs / subscribe to see what has been added, it's a work in progress after all ;)
GMLAN.h
00001 /* 00002 GMLAN.h - Header file for GMLAN Library 00003 00004 GMLAN is a Controller Area Network Bus used in General Motors vehicles from 00005 roughly 2007-onwards. Its purpose is to allow various Electronic Control Units 00006 (aka ECUs) within a modern vehicle to share information and enact procedures. 00007 00008 An example of this would be communication between the HU (Head unit) and the 00009 DIC (Dashboard Information Cluster), when you adjust the volume up / down, this 00010 is reported to the cluster to be displayed. 00011 00012 It is the function of this library to "crack open" this world to allow anyone 00013 with only as little as a few hours of C++ programming under their belt to get 00014 started in what can sometimes seem a daunting world. 00015 00016 Jason Gaunt, 18th Feb 2013 00017 */ 00018 00019 #include "mbed.h" 00020 #include "GMLAN_29bit.h" 00021 #include "GMLAN_11bit.h" 00022 #include <vector> 00023 00024 #ifndef GMLAN_H 00025 #define GMLAN_H 00026 00027 /* Baud rates of various services */ 00028 #define GMLAN_BAUD_LS_NORMAL 33333 00029 #define GMLAN_BAUD_LS_FAST 83333 00030 #define GMLAN_BAUD_MS 95200 00031 #define GMLAN_BAUD_HS 500000 00032 00033 class CANHeader { 00034 /* 00035 CANHeader was designed solely for 29-bit frames but supports 11-bit too by just setting the ArbID 00036 00037 Example 29-bit header packet from Steering Wheel Switches: 00038 00039 Hexadecimal: 0x10 0x0D 0x00 0x60 00040 Binary: 00010000 00001101 00000000 01100000 00041 Priority: --- 00042 Arbitration: -- -------- --- 00043 Sending ECU: ----- -------- 00044 00045 Example 11-bit header packet from Head Unit: 00046 00047 Hexadecimal: 0x02 0x44 00048 Binary: 00000010 01000100 00049 Identifier: --- -------- 00050 00051 */ 00052 00053 private: 00054 int priorityID, arbitrationID, senderID; 00055 00056 public: 00057 // Main function 00058 CANHeader() { } 00059 00060 // Methods for getting / setting priority, both integers 00061 int priority(void) { return priorityID; } 00062 void priority(int _priority) { priorityID = _priority; } 00063 00064 // Method for getting / setting arbitration id aka arbid, both integers 00065 int arbitration(void) { return arbitrationID; } 00066 void arbitration(int _arbitration) { arbitrationID = _arbitration; } 00067 00068 // Method for getting / setting sender id, both integers 00069 int sender(void) { return senderID; } 00070 void sender(int _sender) { senderID = _sender; } 00071 00072 // Function to decode either an 11-bit or 29-bit header packet and store values in respective variables 00073 void decode(int _header); 00074 00075 // Function to encode stored values as 29-bit header and return header packet as int 00076 int encode29bit(void); 00077 00078 // Function to encode stored values as 11-bit header and return header packet as int 00079 int encode11bit(void); 00080 }; 00081 00082 class GMLAN_Message { 00083 /* 00084 Wrapper for CANMessage that automatically parses settings 00085 */ 00086 private: 00087 vector<char> data; 00088 int priority, arbitration, sender; 00089 00090 public: 00091 // Main function 00092 GMLAN_Message(int _priority = -1, int _arbitration = -1, int _sender = -1, 00093 int _b0 = -1, int _b1 = -1, int _b2 = -1, int _b3 = -1, int _b4 = -1, int _b5 = -1, int _b6 = -1, int _b7 = -1); 00094 00095 // Return result 00096 CANMessage generate(void); 00097 }; 00098 00099 class GMLAN_11Bit_Request { 00100 /* 00101 Class to allow easier handling of sending and receiving 11-bit messages 00102 */ 00103 private: 00104 vector<char> request_data, response_data; 00105 int id, request_state; 00106 int tx_frame_counter, tx_bytes; 00107 int rx_frame_counter, rx_bytes; 00108 bool await_response, handle_flowcontrol; 00109 char frame_padding [8]; 00110 00111 public: 00112 // (Main function) Create message and send it 00113 GMLAN_11Bit_Request(int _id, vector<char> _request, bool _await_response = true, bool _handle_flowcontrol = true); 00114 00115 // Process each frame to transmit and flow control frame if needed 00116 CANMessage getNextFrame(void); 00117 CANMessage getFlowControl(void); 00118 // Process each received frame 00119 void processFrame(CANMessage msg); 00120 00121 // Handle starting and flow control 00122 void start(void) { request_state = GMLAN_STATE_SEND_DATA; } 00123 void continueFlow(void) { request_state = GMLAN_STATE_SEND_DATA; } 00124 00125 // Return request_state to confirm status 00126 int getState(void) { return request_state; } 00127 // Return ID 00128 int getID(void) { return id; } 00129 // Return rx_bytes 00130 int getRXcount(void) { return rx_bytes; } 00131 // Return response 00132 vector<char> getResponse(void) { return response_data; } 00133 }; 00134 00135 #endif
Generated on Tue Jul 12 2022 23:00:10 by
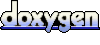