Baseline for testing
Embed:
(wiki syntax)
Show/hide line numbers
mcp23s08.cpp
00001 #include "mbed.h" 00002 #include "mcp23s08.h" 00003 00004 #define INPUT 0 00005 #define OUTPUT 1 00006 00007 #define LOW 0 00008 #define HIGH 1 00009 00010 mcp23s08::mcp23s08(PinName mosi, PinName miso, PinName clk, PinName cs_pin,const uint8_t haenAdrs) : SPI(mosi, miso, clk), cs(cs_pin) { 00011 format(8, 3); 00012 frequency(2000000); 00013 00014 postSetup(haenAdrs); 00015 00016 } 00017 00018 00019 void mcp23s08::postSetup(const uint8_t haenAdrs){ 00020 if (haenAdrs >= 0x20 && haenAdrs <= 0x23){//HAEN works between 0x20...0x23 00021 _adrs = haenAdrs; 00022 _useHaen = 1; 00023 } else { 00024 _adrs = 0; 00025 _useHaen = 0; 00026 } 00027 _readCmd = (_adrs << 1) | 1; 00028 _writeCmd = _adrs << 1; 00029 //setup register values for this chip 00030 IOCON = 0x05; 00031 IODIR = 0x00; 00032 GPPU = 0x06; 00033 GPIO = 0x09; 00034 GPINTEN = 0x02; 00035 IPOL = 0x01; 00036 DEFVAL = 0x03; 00037 INTF = 0x07; 00038 INTCAP = 0x08; 00039 OLAT = 0x0A; 00040 INTCON = 0x04; 00041 } 00042 00043 void mcp23s08::begin(bool protocolInitOverride) { 00044 00045 cs=1; 00046 wait(0.1); 00047 _useHaen == 1 ? writeByte(IOCON,0b00101000) : writeByte(IOCON,0b00100000); 00048 /* 00049 if (_useHaen){ 00050 writeByte(IOCON,0b00101000);//read datasheet for details! 00051 } else { 00052 writeByte(IOCON,0b00100000); 00053 } 00054 */ 00055 _gpioDirection = 0xFF;//all in 00056 _gpioState = 0x00;//all low 00057 } 00058 00059 00060 uint8_t mcp23s08::readAddress(uint8_t addr){ 00061 uint8_t low_byte = 0x00; 00062 startSend(1); 00063 SPI::write(addr); 00064 low_byte = (uint8_t)SPI::write(0x00); 00065 endSend(); 00066 return low_byte; 00067 } 00068 00069 00070 00071 void mcp23s08::gpioPinMode(uint8_t mode){ 00072 if (mode == INPUT){ 00073 _gpioDirection = 0xFF; 00074 } else if (mode == OUTPUT){ 00075 _gpioDirection = 0x00; 00076 _gpioState = 0x00; 00077 } else { 00078 _gpioDirection = mode; 00079 } 00080 writeByte(IODIR,_gpioDirection); 00081 } 00082 00083 void mcp23s08::gpioPinMode(uint8_t pin, bool mode){ 00084 if (pin < 8){//0...7 00085 mode == INPUT ? _gpioDirection |= (1 << pin) :_gpioDirection &= ~(1 << pin); 00086 writeByte(IODIR,_gpioDirection); 00087 } 00088 } 00089 00090 void mcp23s08::gpioPort(uint8_t value){ 00091 if (value == HIGH){ 00092 _gpioState = 0xFF; 00093 } else if (value == LOW){ 00094 _gpioState = 0x00; 00095 } else { 00096 _gpioState = value; 00097 } 00098 writeByte(GPIO,_gpioState); 00099 } 00100 00101 00102 uint8_t mcp23s08::readGpioPort(){ 00103 return readAddress(GPIO); 00104 } 00105 00106 uint8_t mcp23s08::readGpioPortFast(){ 00107 return _gpioState; 00108 } 00109 00110 int mcp23s08::gpioDigitalReadFast(uint8_t pin){ 00111 if (pin < 8){//0...7 00112 int temp = _gpioState & (1 << pin); 00113 return temp; 00114 } else { 00115 return 0; 00116 } 00117 } 00118 00119 void mcp23s08::portPullup(uint8_t data) { 00120 if (data == HIGH){ 00121 _gpioState = 0xFF; 00122 } else if (data == LOW){ 00123 _gpioState = 0x00; 00124 } else { 00125 _gpioState = data; 00126 } 00127 writeByte(GPPU, _gpioState); 00128 } 00129 00130 00131 00132 00133 void mcp23s08::gpioDigitalWrite(uint8_t pin, bool value){ 00134 if (pin < 8){//0...7 00135 value == HIGH ? _gpioState |= (1 << pin) : _gpioState &= ~(1 << pin); 00136 writeByte(GPIO,_gpioState); 00137 } 00138 } 00139 00140 void mcp23s08::gpioDigitalWriteFast(uint8_t pin, bool value){ 00141 if (pin < 8){//0...8 00142 value == HIGH ? _gpioState |= (1 << pin) : _gpioState &= ~(1 << pin); 00143 } 00144 } 00145 00146 void mcp23s08::gpioPortUpdate(){ 00147 writeByte(GPIO,_gpioState); 00148 } 00149 00150 int mcp23s08::gpioDigitalRead(uint8_t pin){ 00151 if (pin < 8) return (int)(readAddress(GPIO) & 1 << pin); 00152 return 0; 00153 } 00154 00155 uint8_t mcp23s08::gpioRegisterReadByte(uint8_t reg){ 00156 uint8_t data = 0; 00157 startSend(1); 00158 SPI::write(reg); 00159 data = (uint8_t)SPI::write(0x00); 00160 endSend(); 00161 return data; 00162 } 00163 00164 00165 void mcp23s08::gpioRegisterWriteByte(uint8_t reg,uint8_t data){ 00166 writeByte(reg,(uint8_t)data); 00167 } 00168 00169 /* ------------------------------ Low Level ----------------*/ 00170 void mcp23s08::startSend(bool mode){ 00171 cs=0; 00172 mode == 1 ? SPI::write(_readCmd) : SPI::write(_writeCmd); 00173 } 00174 00175 void mcp23s08::endSend(){ 00176 cs=1; 00177 } 00178 00179 00180 void mcp23s08::writeByte(uint8_t addr, uint8_t data){ 00181 startSend(0); 00182 SPI::write(addr); 00183 SPI::write(data); 00184 endSend(); 00185 }
Generated on Tue Jul 12 2022 17:43:27 by
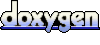