Baseline for testing
Embed:
(wiki syntax)
Show/hide line numbers
ButtonController.h
00001 #ifndef _BUTTON_CONTROLLER_ 00002 #define _BUTTON_CONTROLLER_ 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #include "mcp23s08.h" 00007 #include "Menu.h" 00008 #include "Navigator.h" 00009 00010 /** 00011 * service to manage the external GPIO expander board. 00012 */ 00013 typedef enum 00014 { 00015 NO_BUTTON = 27, 00016 BUTTON_UP = 26, 00017 BUTTON_DOWN = 25, 00018 BUTTON_MODE = 19, 00019 BUTTON_SET = 11, 00020 BUTTON_CLEAR = 0 00021 } tButtonValue ; 00022 00023 typedef void (*t_ButtonPressCallback)(void); 00024 00025 00026 /*** 00027 * 00028 * This class wrapps the communication with the GPIO expander over SPI bus. 00029 * PIN 0 : Up 00030 * PIN 1 : Down 00031 * PIN 2 : Clear 00032 * PIN 3 : Mode 00033 * PIN 4 : Set 00034 * PIN 5 : Enable Audio 00035 * PIN 6 : na 00036 * PIN 7 : na 00037 * 00038 * MASK = 0x1F; 00039 * 00040 */ 00041 class ButtonController 00042 { 00043 public : 00044 00045 Navigator * navigator; 00046 mcp23s08 * spi_io_exp; 00047 Mutex _mutex; 00048 unsigned char currentValue; 00049 unsigned char prevValue; 00050 unsigned char confirmedValue; 00051 unsigned int countsSinceChange; 00052 unsigned char isServiced; 00053 unsigned char isHeld; 00054 00055 00056 CircularBuffer<uint8_t, 64> cmd_queue; 00057 00058 ButtonController(Navigator * navigator); 00059 ~ButtonController(); 00060 00061 void pressButtonUp(void); 00062 void pressButtonDown(void); 00063 void pressButtonClear(void); 00064 void pressButtonMode(void); 00065 void pressButtonSet(void); 00066 void releaseButton(void); 00067 00068 void init(void); 00069 void update(void); 00070 void update(Navigator * navigator); 00071 void update(Menu * menu); 00072 void update(int currentValue, Menu * menu); 00073 00074 uint8_t getCurrentState (); 00075 }; 00076 00077 00078 #endif
Generated on Tue Jul 12 2022 17:43:27 by
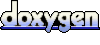