
I needed a device to time my sprinklers, because I had been forgetting to turn them off. So I made a very simple program to turn them off after 45 minutes. Future versions will have an array of outputs, so you can run sections with one button push. Hardware: Either board should work, I used the LPC1768. You will also need a solenoid to control the water, I am testing a 12V rainbird valve with a relay and 12V power supply.
main.cpp
00001 #include "mbed.h" 00002 #include "PinDetect.h" 00003 00004 // Objects 00005 DigitalOut myLed(LED1); 00006 DigitalOut solenoid(p6); 00007 PinDetect button(p5); 00008 Timer timer; 00009 00010 // Primitives 00011 int timeInMinutes = 25; // Timer is only good to about 30 mins! 00012 float halfSecond = 0.5; // LED blink rate 00013 00014 // Interrupt function for start/stop button 00015 ////////////////////////////////////////////////// 00016 void StartStop() { 00017 // Enabled = !Enabled 00018 if(solenoid.read()) { 00019 // Turn off 00020 timer.stop(); 00021 solenoid = false; 00022 } else { 00023 // Turn on 00024 timer.reset(); 00025 timer.start(); 00026 solenoid = true; 00027 } 00028 } 00029 00030 // Main loop 00031 ////////////////////////////////////////////////// 00032 int main() { 00033 // Set button interrupt w/PullDown and debounce 00034 button.mode(PullDown); 00035 button.attach_asserted_held(&StartStop); 00036 button.setSampleFrequency(9); 00037 00038 // Infinite loop 00039 while(true) { 00040 // Stop if time(s) > n Mins(m) * 60(s) 00041 if(timer.read() > timeInMinutes * 60) { 00042 solenoid = false; 00043 timer.stop(); 00044 } 00045 00046 // Slow pulse status LED when enabled 00047 if(solenoid.read()) 00048 myLed = !myLed.read(); 00049 else 00050 myLed = false; 00051 00052 // Wait so LED pulses 00053 wait(halfSecond); 00054 } 00055 }
Generated on Sat Jul 30 2022 00:11:34 by
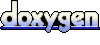