
This is the Mexican Standoff prototype made by Francisco Martin and Andrew Smith. Please refer to the following link for instructions on hardware hookup: https://developer.mbed.org/users/fomartin/notebook/mexican-standoff-reaction-game/
Dependencies: SDFileSystem mbed-rtos mbed wave_player 4DGL-uLCD-SE PinDetect
main.cpp
00001 #include "States.h" 00002 #include "mbed.h" 00003 00004 //button setup using PinDetect 00005 // button is pushed -> false 00006 // button is not pushed -> true 00007 PinDetect P1_LeftButton(p15, PullUp); 00008 PinDetect P1_RightButton(p16, PullUp); 00009 PinDetect P2_LeftButton(p26, PullUp); 00010 PinDetect P2_RightButton(p29, PullUp); 00011 00012 //LCD setup 00013 uLCD_4DGL uLCD(p28, p27, p21); // serial tx, serial rx, reset pin; 00014 00015 //speaker setup 00016 AnalogOut speaker(p18); 00017 wave_player waver(&speaker); 00018 00019 //states enum 00020 enum StateType{MainMenu, HowTo, SinglePlayerGame, TwoPlayerGame, EndScreen}; 00021 StateType state = MainMenu; 00022 00023 00024 SDFileSystem sd(p5, p6, p7, p8, "sd"); //SD card 00025 00026 //member variables 00027 int option; 00028 int points; 00029 00030 DigitalOut led1(LED1); 00031 00032 main() 00033 { 00034 Music music(waver); 00035 00036 //LCD setup 00037 uLCD.display_control(PORTRAIT_R); 00038 uLCD.baudrate(BAUD_3000000); //jack up baud rate to max for fast display 00039 uLCD.background_color(BLACK); 00040 uLCD.cls(); 00041 00042 music.playMainMusic(); 00043 00044 while(true) 00045 { 00046 switch(state) 00047 { 00048 case(MainMenu): 00049 { 00050 // Set-Up Main Menu 00051 Startup startup(uLCD, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton); 00052 //startup.scores(uLCD, sd); 00053 option = startup.select(uLCD, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton, music); 00054 if(option == 0) 00055 state = SinglePlayerGame; 00056 if(option == 1) 00057 state = TwoPlayerGame; 00058 if(option == 2) 00059 state = HowTo; 00060 break; 00061 } 00062 case(HowTo): 00063 { 00064 Rules rules(uLCD, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton); 00065 state = MainMenu; 00066 break; 00067 } 00068 case(SinglePlayerGame): 00069 { 00070 //after gameplay returns, we can use gameplay.getWinningPlayer() to find out who won. 00071 //this gets passed to the GameOver to display the correct winner on the screen 00072 Gameplay gameplay(uLCD, 1, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton); 00073 GameOver gameover(uLCD, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton, gameplay.getWinningPlayer()); 00074 state = MainMenu; 00075 break; 00076 } 00077 case(TwoPlayerGame): 00078 { 00079 Gameplay gameplay(uLCD, 2, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton); 00080 GameOver gameover(uLCD, P1_LeftButton, P1_RightButton, P2_LeftButton, P2_RightButton, gameplay.getWinningPlayer()); 00081 state = MainMenu; 00082 break; 00083 } 00084 }//end switch 00085 }//end while 00086 }//end main
Generated on Fri Jul 22 2022 05:53:26 by
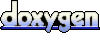