
989
Dependencies: mbed Servo X_NUCLEO_IKS01A2 X_NUCLEO_IDW01M1v2 NetworkSocketAPI NDefLib MQTT
main.cpp
00001 #include "mbed.h" 00002 #include "SpwfInterface.h" 00003 #include "TCPSocket.h" 00004 #include "MQTTClient.h" 00005 #include "MQTTWiFi.h" 00006 #include <ctype.h> 00007 #include "NDefLib/NDefNfcTag.h" 00008 #include "NDefLib/RecordType/RecordURI.h" 00009 #include "Servo.h" 00010 #include"XNucleoIKS01A2.h" 00011 #include "stm32f4xx_hal.h" 00012 Servo servo(D10); 00013 00014 float range = 0.005; 00015 float position = 0.5; 00016 00017 Serial pc(SERIAL_TX, SERIAL_RX); 00018 bool quickstartMode = true; 00019 00020 #define ORG_QUICKSTART 101.132.47.170 // comment to connect to play.internetofthings.ibmcloud.com 00021 //#define SUBSCRIBE // uncomment to subscribe to broker msgs (not to be used with IBM broker) 00022 00023 #define MQTT_MAX_PACKET_SIZE 250 00024 #define MQTT_MAX_PAYLOAD_SIZE 300 00025 00026 // Configuration values needed to connect to IBM IoT Cloud 00027 #define BROKER_URL ".132.47.170"; 00028 #ifdef ORG_QUICKSTART 00029 #define ORG "101" // connect to quickstart.internetofthings.ibmcloud.com/ For a registered connection, replace with your org 00030 #define ID "168" 00031 #define AUTH_TOKEN "10" 00032 #define DEFAULT_TYPE_NAME "145" 00033 #else // not def ORG_QUICKSTART 00034 #define ORG "192" // connect to play.internetofthings.ibmcloud.com/ For a registered connection, replace with your org 00035 #define ID "168" // For a registered connection, replace with your id 00036 #define AUTH_TOKEN "10"// For a registered connection, replace with your auth-token 00037 #define DEFAULT_TYPE_NAME "145" 00038 #endif 00039 #define TOPIC "TempA" 00040 #define TOPIC2 "commond" 00041 #define TOPICT_hum "hum" 00042 #define TOPICT_x "x" 00043 #define TOPICT_y "y" 00044 #define TOPICT_z "z" 00045 #define TOPICT_ax "a_x" 00046 #define TOPICT_ay "a_y" 00047 #define TOPICT_az "a_z" 00048 #define TOPICT_bx "b_x" 00049 #define TOPICT_by "b_y" 00050 #define TOPICT_bz "b_z" 00051 00052 00053 #define TYPE DEFAULT_TYPE_NAME // For a registered connection, replace with your type 00054 #define MQTT_PORT 1883 00055 #define MQTT_TLS_PORT 8883 00056 #define IBM_IOT_PORT MQTT_PORT 00057 // WiFi network credential 00058 #define SSID "LongFreeMoble" // Network must be visible otherwise it can't connect 00059 #define PASSW "iots8230" 00060 #warning "Wifi SSID & password empty" 00061 char* topic = "TempA"; 00062 00063 char id[30] = ID; // mac without colons 00064 char org[12] = ORG; 00065 int connack_rc = 0; // MQTT connack return code 00066 const char* ip_addr = ""; 00067 char* host_addr = ""; 00068 char type[30] = TYPE; 00069 char auth_token[30] = AUTH_TOKEN; // Auth_token is only used in non-quickstart mode 00070 bool netConnecting = false; 00071 int connectTimeout = 1000; 00072 bool mqttConnecting = false; 00073 bool netConnected = false; 00074 bool connected = false; 00075 int retryAttempt = 0; 00076 char subscription_url[MQTT_MAX_PAYLOAD_SIZE]; 00077 00078 00079 static XNucleoIKS01A2 *mems_expansion_board = XNucleoIKS01A2::instance(D14, D15, D4, D5); 00080 00081 /* Retrieve the composing elements of the expansion board */ 00082 static LSM303AGRMagSensor *magnetometer = mems_expansion_board->magnetometer; 00083 static HTS221Sensor *hum_temp = mems_expansion_board->ht_sensor; 00084 static LPS22HBSensor *press_temp = mems_expansion_board->pt_sensor; 00085 static LSM6DSLSensor *acc_gyro = mems_expansion_board->acc_gyro; 00086 static LSM303AGRAccSensor *accelerometer = mems_expansion_board->accelerometer; 00087 00088 class WatchDog { 00089 00090 private: 00091 00092 IWDG_HandleTypeDef hiwdg; 00093 00094 public: 00095 00096 WatchDog(uint32_t prescaler = IWDG_PRESCALER_256, uint32_t reload = 0xfff) { 00097 00098 hiwdg.Instance = IWDG; 00099 00100 hiwdg.Init.Prescaler = prescaler; 00101 00102 hiwdg.Init.Reload = reload; 00103 00104 HAL_IWDG_Init(&hiwdg); 00105 00106 } 00107 00108 void feed() { 00109 00110 HAL_IWDG_Refresh(&hiwdg); 00111 00112 } 00113 00114 }; 00115 00116 00117 /* Retrieve the composing elements of the expansion board */ 00118 //PressureSensor *pressure_sensor; 00119 //HumiditySensor *humidity_sensor; 00120 //TempSensor *temp_sensor1; 00121 00122 MQTT::Message message; 00123 MQTTString TopicName={TOPIC}; 00124 MQTT::MessageData MsgData(TopicName, message); 00125 00126 void subscribe_cb(MQTT::MessageData & msgMQTT) { 00127 char msg[MQTT_MAX_PAYLOAD_SIZE]; 00128 msg[0]='\0'; 00129 strncat (msg, (char*)msgMQTT.message.payload, msgMQTT.message.payloadlen); 00130 printf ("--->>> subscribe_cb msg: %s\n\r", msg); 00131 printf("%d\r\n",strlen(msg)); 00132 if(strlen(msg)==2) 00133 { 00134 printf ("I AM WORKING\n\r"); 00135 int i=0; 00136 while(i<500) { 00137 i++; 00138 servo = position; 00139 position += range; 00140 if(position >= 1) { 00141 position = 0; 00142 wait(0.5); 00143 } 00144 wait(0.002); 00145 } 00146 } 00147 } 00148 00149 int subscribe(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00150 { 00151 char* pubTopic = TOPIC2; 00152 printf("subscribe int finished\r\n"); 00153 return client->subscribe(pubTopic, MQTT::QOS1, subscribe_cb); 00154 } 00155 00156 int connect(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00157 { 00158 const char* iot_ibm = BROKER_URL; 00159 00160 00161 char hostname[strlen(org) + strlen(iot_ibm) + 1]; 00162 sprintf(hostname, "%s%s", org, iot_ibm); 00163 SpwfSAInterface& WiFi = ipstack->getWiFi(); 00164 char clientId[strlen(org) + strlen(type) + strlen(id) + 5]; 00165 sprintf(clientId, "d:%s:%s:%s", org, type, id); 00166 sprintf(subscription_url, "%s.%s/#/device/%s/sensor/", org, "iots",id); 00167 00168 // Network debug statements 00169 LOG("\n\r=====================================\n\r"); 00170 LOG("Connecting WiFi.\n\r"); 00171 LOG("Nucleo IP ADDRESS: %s\n\r", WiFi.get_ip_address()); 00172 LOG("Nucleo MAC ADDRESS: %s\n\r", WiFi.get_mac_address()); 00173 LOG("Server Hostname: %s port: %d\n\r", hostname, IBM_IOT_PORT); 00174 LOG("Client ID: %s\n\r", clientId); 00175 LOG("Topic: %s\n\r",TOPIC); 00176 LOG("Subscription URL: %s\n\r", subscription_url); 00177 LOG("=====================================\n\r"); 00178 00179 netConnecting = true; 00180 ipstack->open(&ipstack->getWiFi()); 00181 int rc = ipstack->connect(hostname, IBM_IOT_PORT, connectTimeout); 00182 if (rc != 0) 00183 { 00184 WARN("IP Stack connect returned: %d\n", rc); 00185 return rc; 00186 } 00187 printf ("--->TCP Connected\n\r"); 00188 netConnected = true; 00189 netConnecting = false; 00190 00191 // MQTT Connect 00192 mqttConnecting = true; 00193 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00194 data.MQTTVersion = 4; 00195 data.struct_version=0; 00196 data.clientID.cstring = clientId; 00197 00198 if (!quickstartMode) 00199 { 00200 data.username.cstring = "use-token-auth"; 00201 data.password.cstring = auth_token; 00202 } 00203 if ((rc = client->connect(data)) == 0) 00204 { 00205 connected = true; 00206 printf ("--->MQTT Connected\n\r"); 00207 #ifdef SUBSCRIBE 00208 if (!subscribe(client, ipstack)) printf ("--->>>MQTT subscribed to: %s\n\r",TOPIC); 00209 #endif 00210 } 00211 else { 00212 WARN("MQTT connect returned %d\n", rc); 00213 } 00214 if (rc >= 0) 00215 connack_rc = rc; 00216 mqttConnecting = false; 00217 return rc; 00218 } 00219 00220 int getConnTimeout(int attemptNumber) 00221 { // First 10 attempts try within 3 seconds, next 10 attempts retry after every 1 minute 00222 // after 20 attempts, retry every 10 minutes 00223 return (attemptNumber < 10) ? 3 : (attemptNumber < 20) ? 60 : 600; 00224 } 00225 00226 void attemptConnect(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00227 { 00228 connected = false; 00229 00230 while (connect(client, ipstack) != MQTT_CONNECTION_ACCEPTED) 00231 { 00232 if (connack_rc == MQTT_NOT_AUTHORIZED || connack_rc == MQTT_BAD_USERNAME_OR_PASSWORD) { 00233 printf ("File: %s, Line: %d Error: %d\n\r",__FILE__,__LINE__, connack_rc); 00234 return; // don't reattempt to connect if credentials are wrong 00235 } 00236 int timeout = getConnTimeout(++retryAttempt); 00237 WARN("Retry attempt number %d waiting %d\n", retryAttempt, timeout); 00238 00239 // if ipstack and client were on the heap we could deconstruct and goto a label where they are constructed 00240 // or maybe just add the proper members to do this disconnect and call attemptConnect(...) 00241 // this works - reset the system when the retry count gets to a threshold 00242 if (retryAttempt == 5) 00243 NVIC_SystemReset(); 00244 else 00245 wait(timeout); 00246 } 00247 } 00248 00249 int publish(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00250 { 00251 MQTT::Message message; 00252 char* pubTopic = TOPIC; 00253 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00254 float temp, press, hum; 00255 printf("%f\r\n",press); 00256 hum_temp->get_temperature(&temp); 00257 hum_temp->get_humidity(&hum); 00258 // temp_sensor1->GetTemperature(&temp); 00259 // humidity_sensor->GetHumidity(&hum); 00260 // pressure_sensor->GetPressure(&press); 00261 00262 00263 sprintf(buf,"%0.4f",temp); 00264 message.qos = MQTT::QOS1; 00265 message.retained = false; 00266 message.dup = false; 00267 message.payload = (void*)buf; 00268 message.payloadlen = strlen(buf); 00269 //message.payloadlen = sizeof(temp); 00270 00271 // LOG("Publishing %s\n\r", buf); 00272 printf("Publishing hum %s\n\r", buf); 00273 return client->publish(pubTopic, message); 00274 } 00275 int publish2(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00276 { 00277 MQTT::Message message; 00278 char* pubTopic = TOPICT_hum; 00279 uint8_t id; 00280 00281 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00282 float temp, press, hum; 00283 printf("%f\r\n",press); 00284 hum_temp->get_temperature(&temp); 00285 hum_temp->get_humidity(&hum); 00286 // temp_sensor1->GetTemperature(&temp); 00287 // humidity_sensor->GetHumidity(&hum); 00288 // pressure_sensor->GetPressure(&press); 00289 00290 00291 sprintf(buf,"%0.4f",hum); 00292 message.qos = MQTT::QOS1; 00293 message.retained = false; 00294 message.dup = false; 00295 message.payload = (void*)buf; 00296 message.payloadlen = strlen(buf); 00297 //message.payloadlen = sizeof(temp); 00298 00299 // LOG("Publishing %s\n\r", buf); 00300 printf("Publishing temp %s\n\r", buf); 00301 return client->publish(pubTopic, message); 00302 } 00303 int publish3(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00304 { 00305 int32_t axes[3]; 00306 MQTT::Message message; 00307 char* pubTopic = TOPICT_x; 00308 uint8_t id; 00309 00310 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00311 float temp, press, hum; 00312 printf("%f\r\n",press); 00313 hum_temp->get_temperature(&temp); 00314 hum_temp->get_humidity(&hum); 00315 magnetometer->get_m_axes(axes); 00316 00317 00318 sprintf(buf,"%0.4d",axes[0]); 00319 message.qos = MQTT::QOS1; 00320 message.retained = false; 00321 message.dup = false; 00322 message.payload = (void*)buf; 00323 message.payloadlen = strlen(buf); 00324 printf("Publishing x %s\n\r", buf); 00325 return client->publish(pubTopic, message); 00326 } 00327 int publish4(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00328 { 00329 int32_t axes[3]; 00330 MQTT::Message message; 00331 char* pubTopic = TOPICT_y; 00332 uint8_t id; 00333 00334 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00335 magnetometer->get_m_axes(axes); 00336 00337 printf("axes's number%d\r\n",axes[1]); 00338 sprintf(buf,"%0.4d",axes[1]); 00339 message.qos = MQTT::QOS1; 00340 message.retained = false; 00341 message.dup = false; 00342 message.payload = (void*)buf; 00343 message.payloadlen = strlen(buf); 00344 printf("Publishing y %s\n\r", buf); 00345 return client->publish(pubTopic, message); 00346 } 00347 int publish5(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00348 { 00349 int32_t axes[3]; 00350 MQTT::Message message; 00351 char* pubTopic = TOPICT_z; 00352 uint8_t id; 00353 00354 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00355 float temp, press, hum; 00356 hum_temp->get_temperature(&temp); 00357 hum_temp->get_humidity(&hum); 00358 magnetometer->get_m_axes(axes); 00359 00360 00361 sprintf(buf,"%0.4d",axes[2]); 00362 message.qos = MQTT::QOS1; 00363 message.retained = false; 00364 message.dup = false; 00365 message.payload = (void*)buf; 00366 message.payloadlen = strlen(buf); 00367 //message.payloadlen = sizeof(temp); 00368 00369 // LOG("Publishing %s\n\r", buf); 00370 printf("Publishing z %s\n\r", buf); 00371 return client->publish(pubTopic, message); 00372 } 00373 int publish7(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00374 { 00375 int32_t axes[3]; 00376 MQTT::Message message; 00377 char* pubTopic = TOPICT_ax; 00378 00379 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00380 accelerometer->get_x_axes(axes); 00381 sprintf(buf,"%0.4d",axes[0]); 00382 message.qos = MQTT::QOS0; 00383 message.retained = false; 00384 message.dup = false; 00385 message.payload = (void*)buf; 00386 message.payloadlen = strlen(buf); 00387 printf("Publishing %s\n\r", buf); 00388 return client->publish(pubTopic, message); 00389 } 00390 int publish8(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00391 { 00392 int32_t axes[3]; 00393 MQTT::Message message; 00394 char* pubTopic = TOPICT_ay; 00395 00396 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00397 accelerometer->get_x_axes(axes); 00398 sprintf(buf,"%0.4d",axes[1]); 00399 message.qos = MQTT::QOS0; 00400 message.retained = false; 00401 message.dup = false; 00402 message.payload = (void*)buf; 00403 message.payloadlen = strlen(buf); 00404 printf("Publishing %s\n\r", buf); 00405 return client->publish(pubTopic, message); 00406 } 00407 int publish9(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00408 { 00409 int32_t axes[3]; 00410 MQTT::Message message; 00411 char* pubTopic = TOPICT_az; 00412 00413 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00414 accelerometer->get_x_axes(axes); 00415 sprintf(buf,"%0.4d",axes[2]); 00416 message.qos = MQTT::QOS0; 00417 message.retained = false; 00418 message.dup = false; 00419 message.payload = (void*)buf; 00420 message.payloadlen = strlen(buf); 00421 printf("Publishing %s\n\r", buf); 00422 return client->publish(pubTopic, message); 00423 } 00424 int publish10(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00425 { 00426 int32_t axes[3]; 00427 MQTT::Message message; 00428 char* pubTopic = TOPICT_bx; 00429 00430 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00431 00432 acc_gyro->get_x_axes(axes); 00433 sprintf(buf,"%0.4d",axes[0]); 00434 message.qos = MQTT::QOS0; 00435 message.retained = false; 00436 message.dup = false; 00437 message.payload = (void*)buf; 00438 message.payloadlen = strlen(buf); 00439 printf("Publishing %s\n\r", buf); 00440 return client->publish(pubTopic, message); 00441 } 00442 int publish11(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00443 { 00444 int32_t axes[3]; 00445 MQTT::Message message; 00446 char* pubTopic = TOPICT_by; 00447 00448 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00449 00450 acc_gyro->get_x_axes(axes); 00451 sprintf(buf,"%0.4d",axes[1]); 00452 message.qos = MQTT::QOS0; 00453 message.retained = false; 00454 message.dup = false; 00455 message.payload = (void*)buf; 00456 message.payloadlen = strlen(buf); 00457 printf("Publishing %s\n\r", buf); 00458 return client->publish(pubTopic, message); 00459 } 00460 int publish12(MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTWiFi* ipstack) 00461 { 00462 int32_t axes[3]; 00463 MQTT::Message message; 00464 char* pubTopic = TOPICT_bz; 00465 00466 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00467 00468 acc_gyro->get_x_axes(axes); 00469 sprintf(buf,"%0.4d",axes[2]); 00470 message.qos = MQTT::QOS0; 00471 message.retained = false; 00472 message.dup = false; 00473 message.payload = (void*)buf; 00474 message.payloadlen = strlen(buf); 00475 printf("Publishing %s\n\r", buf); 00476 return client->publish(pubTopic, message); 00477 } 00478 00479 00480 int main() 00481 { 00482 00483 const char * ssid = SSID; // Network must be visible otherwise it can't connect 00484 const char * seckey = PASSW; 00485 SpwfSAInterface spwf(D8, D2, false); 00486 DevI2C *i2c = new DevI2C(I2C_SDA, I2C_SCL); 00487 i2c->frequency(400000); 00488 00489 00490 00491 00492 pc.printf("\r\nX-NUCLEO-IDW01M1 mbed Application\r\n"); 00493 pc.printf("\r\nconnecting to AP\r\n"); 00494 00495 hum_temp->enable(); 00496 press_temp->enable(); 00497 magnetometer->enable(); 00498 accelerometer->enable(); 00499 acc_gyro->enable_x(); 00500 acc_gyro->enable_g(); 00501 00502 quickstartMode=false; 00503 if (strcmp(org, "quickstart") == 0){quickstartMode = true;} 00504 MQTTWiFi ipstack(spwf, ssid, seckey, NSAPI_SECURITY_WPA2); 00505 MQTT::Client<MQTTWiFi, Countdown, MQTT_MAX_PACKET_SIZE> client(ipstack); 00506 if (quickstartMode){ 00507 char mac[50]; // remove all : from mac 00508 char *digit=NULL; 00509 sprintf (id,"%s", ""); 00510 sprintf (mac,"%s",ipstack.getWiFi().get_mac_address()); 00511 digit = strtok (mac,":"); 00512 while (digit != NULL) 00513 { 00514 strcat (id, digit); 00515 digit = strtok (NULL, ":"); 00516 } 00517 } 00518 attemptConnect(&client, &ipstack); 00519 if (connack_rc == MQTT_NOT_AUTHORIZED || connack_rc == MQTT_BAD_USERNAME_OR_PASSWORD) 00520 { 00521 while (true) 00522 wait(1.0); // Permanent failures - don't retry 00523 } 00524 WatchDog wdg(IWDG_PRESCALER_64, 62500); 00525 int count = 0; 00526 // tyeld.start(); 00527 while (true) 00528 { 00529 wdg.feed(); 00530 if (++count == 100) 00531 { 00532 if(subscribe(&client, &ipstack)>30){ 00533 printf("ok \n\r"); 00534 } 00535 publish2(&client, &ipstack); 00536 publish3(&client, &ipstack); 00537 publish4(&client, &ipstack); 00538 publish5(&client, &ipstack); 00539 publish5(&client, &ipstack); 00540 publish7(&client, &ipstack); 00541 publish8(&client, &ipstack); 00542 publish9(&client, &ipstack); 00543 publish10(&client, &ipstack); 00544 publish11(&client, &ipstack); 00545 publish12(&client, &ipstack); 00546 if (publish(&client, &ipstack) != 0) { 00547 attemptConnect(&client, &ipstack); // if we have lost the connection 00548 } 00549 count = 0; 00550 } 00551 client.yield(10); 00552 } 00553 }
Generated on Sat Aug 13 2022 13:02:27 by
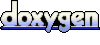