
Renamed
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /******************************************** 00002 Send File to Sharp PC-1403(H) 00003 ========================================== 00004 00005 Author: Fabio Fumi 00006 Date: 04.03.2022 00007 00008 This software comes with the GNU public licence (GPL). 00009 00010 It is an adaptation to the Mbed OS of the 00011 img2wav from the "Pocket Tools" suite 00012 https://www.peil-partner.de/ifhe.de/sharp/ 00013 *********************************************/ 00014 #include "mbed.h" 00015 #include "send_pc1403.h" 00016 //#ifdef FILE_BUF_SIZE 00017 //volatile char debugOut[1024]; 00018 //#endif 00019 RawSerial pc(USBTX,USBRX); // better than Serial, when using interrupts 00020 DigitalOut my_led(LED1); 00021 DigitalIn my_button(USER_BUTTON); 00022 InterruptIn btn(USER_BUTTON); 00023 Timer total_time; 00024 Ticker blink; 00025 00026 // bin-to-wav conversion information (from Pocket Tools code) 00027 int send_err; 00028 00029 void ledBlink () { 00030 my_led = !my_led; 00031 } 00032 00033 #ifdef FILE_BUF_SIZE 00034 void printInfo() 00035 { 00036 int i ; 00037 // printout info 00038 pc.printf("totBytesReceived %d\n\r", totBytesReceived); 00039 pc.printf("fileBufReceivePtr %d\n\r", fileBufReceivePtr); 00040 for ( i=0; i<10; i++ ) 00041 pc.printf("0x%.2X ", fileOverSerialBuffer[i] ); 00042 pc.printf("...\n\r"); 00043 pc.printf("fileBufSendPtr %d\n\r", fileBufSendPtr); 00044 //pc.printf("<%s>\n\r", debugOut); 00045 pc.printf("fileReceiveComplete %d\n\r", fileReceiveComplete); 00046 pc.printf("fileError %d\n\r", fileError); 00047 pc.printf("fileInfo.total %d\n\r", fileInfo.total); 00048 pc.printf("time (ms): %d [send_err %d]\n\r", total_time.read_us()/1000, send_err); 00049 00050 } 00051 00052 // invoked at each character received over serial 00053 void serial_rx() { 00054 00055 // getting data from serial 00056 if ( totBytesReceived == 0 ) { 00057 timeout.start(); // new file: enable a watchdog 00058 timeout.reset(); 00059 blink.attach( &ledBlink, 0.05 ); // fast blink: receiving 00060 } 00061 // push character on the circular buffer 00062 filePushData ( pc.getc() ); 00063 totBytesReceived++; 00064 timeout.reset(); 00065 00066 } 00067 #endif 00068 00069 00070 int main() 00071 { 00072 00073 my_led = 0; 00074 pc.baud(600); // file-over-serial can't go faster (sending to Sharp is about 500baud) 00075 fflush(stdout); 00076 #ifdef FILE_BUF_SIZE 00077 btn.rise(&printInfo); 00078 pc.attach(&serial_rx, Serial::RxIrq); 00079 fileReceiveInit( ); 00080 #endif 00081 // main loop 00082 while(true) { 00083 00084 blink.attach( &ledBlink, 1.0 ); // slow blink - waiting for file 00085 00086 #ifdef FILE_BUF_SIZE 00087 // File-over-serial 00088 // serial interrupt - pushing to buffer 00089 // main thread - pulling from buffer 00090 fileInfo.debug = 0x1000; // disable verbose printf while using serial 00091 fileReceiveInit( ); 00092 // wait for data available, before starting to send 00093 while ( !totBytesReceived ) 00094 wait (.1); 00095 #else 00096 // hardcoded BIN file 00097 pc.printf("Push user button to start sending \n\r"); 00098 // wait for button pressed, only for the hardcoded BIN file 00099 while ( my_button == 1 ) 00100 wait ( .1 ); 00101 #endif 00102 00103 // start sending new file to Sharp 00104 fileInfo.ident = IDENT_NEW_BAS; 00105 fileInfo.debug = 0x0040; // 0x0000 disable printf; 0x0040 DEBUG 00106 bitHandlerInit(); // new bit stream being sent 00107 total_time.reset(); 00108 total_time.start(); 00109 send_err = FileSend ( ) ; // removed for debug ! 00110 bitHandlerStop(); // stop bit consumer, after last bit 00111 while( bitHandlerRunning() ) // time for bit handler to complete 00112 wait (.1); 00113 total_time.stop(); 00114 #ifdef FILE_BUF_SIZE 00115 printInfo() ; 00116 #endif 00117 pc.printf("DONE - time (ms): %d [send_err %d]\n\r", total_time.read_us()/1000, send_err); 00118 00119 // doing nothing... 00120 wait(.1); 00121 00122 } 00123 }
Generated on Sat Jul 16 2022 05:01:30 by
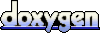