
VGA Game
Dependencies: 4DGL LSM9DS0 mbed
main.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "LSM9DS0.h" 00021 #include <stdio.h> 00022 #include <stdlib.h> 00023 #include <time.h> 00024 #include <string> 00025 #include "TFT_4DGL.h" 00026 #include <iostream> 00027 00028 // overwrite 4DGL library screen size settings in TFT_4DGL.h 00029 #define SIZE_X 479 00030 #define SIZE_Y 639 00031 // 00032 00033 // SDO_XM and SDO_G are pulled up, so our addresses are: 00034 #define LSM9DS0_XM_ADDR 0x1D // Would be 0x1E if SDO_XM is LOW 00035 #define LSM9DS0_G_ADDR 0x6B // Would be 0x6A if SDO_G is LOW 00036 00037 #define REFRESH_TIME_MS 200 00038 00039 TFT_4DGL lcd(p13,p14,p11); // serial tx, serial rx, reset pin; 00040 LSM9DS0 imu(p9, p10, LSM9DS0_G_ADDR, LSM9DS0_XM_ADDR); 00041 00042 class Brick { 00043 public: 00044 int x; 00045 int y; 00046 int color; 00047 int width; 00048 int height; 00049 bool exist; 00050 00051 Brick(){ 00052 width = 10 * 2; 00053 height = 5 * 2; 00054 exist = true; 00055 } 00056 00057 void set(int x, int y){ 00058 this->x = x; 00059 this->y = y; 00060 } 00061 }; 00062 00063 int main() { 00064 00065 //Initial setup 00066 uint16_t status = imu.begin(); 00067 lcd.baudrate(128000); 00068 // added - Set Display to 640 by 480 mode 00069 lcd.display_control(0x0c, 0x01); 00070 lcd.background_color(0); 00071 lcd.cls(); 00072 00073 int score = 0; 00074 Brick* wall[32]; 00075 srand(time(0)); 00076 for(int i = 0; i < 8; i ++){ 00077 for( int j = 0; j < 4; j++){ 00078 Brick * brick = new Brick(); 00079 brick->set(5 + i * brick->width, 20 + j * brick->height); 00080 int c = 0; 00081 int num = rand() % 8 + 1; 00082 switch (num) { 00083 case 1: 00084 c = 0xff0000; 00085 break; 00086 case 2: 00087 c = 0xffff00; 00088 break; 00089 case 3: 00090 c = 0x00FF00; 00091 break; 00092 case 4: 00093 c = 0x0000FF; 00094 break; 00095 case 5: 00096 c = 0xFFC0CB; 00097 break; 00098 case 6: 00099 c = 0xA52A2A; 00100 break; 00101 case 7: 00102 c = 0xd3d3d3; 00103 break; 00104 case 8: 00105 c = 0x800080; 00106 break; 00107 default: 00108 c = 0x445566; 00109 } 00110 brick->color = c; 00111 wall[i*4 + j] = brick; 00112 } 00113 } 00114 00115 float player_x = 60.0 * 2; 00116 float player_y = 120.0 * 2; 00117 float p_width = 30.0; 00118 00119 float ball_x = 23.0 * 2; 00120 float ball_y = 49.0 * 2; 00121 float ball_vx = 15.0; 00122 float ball_vy = 15.0; 00123 float ball_r = 2.0; 00124 float width = 110.0 * 2; 00125 float height = 120.0 * 2; 00126 bool end = false; 00127 bool win = false; 00128 00129 while (!end && !win) 00130 { 00131 lcd.cls(); 00132 imu.readMag(); 00133 imu.readAccel(); 00134 00135 for(int i = 0; i < 32; i++ ){ 00136 if(wall[i]->exist){ 00137 lcd.rectangle(wall[i]->x, wall[i]->y, wall[i]->x + wall[i]->width, wall[i]->y + wall[i]->height, wall[i]->color); 00138 } 00139 } 00140 00141 lcd.circle(ball_x, ball_y, ball_r, RED); 00142 lcd.line(player_x, player_y, player_x + p_width, player_y, BLUE); 00143 00144 ball_x += ball_vx * 0.7; 00145 ball_y += ball_vy * 0.7; 00146 player_x = player_x - imu.ax_raw * 0.003; 00147 00148 if(player_x + p_width > width){ 00149 player_x = width - p_width; 00150 } 00151 00152 if(player_x < 0){ 00153 player_x = 0; 00154 } 00155 00156 if(ball_x + ball_r > width){ 00157 ball_x = width - ball_r; 00158 ball_vx *= -1; 00159 } 00160 00161 if(ball_x - ball_r < 0){ 00162 ball_x = ball_r; 00163 ball_vx *= -1; 00164 } 00165 00166 if(ball_y + ball_r > height){ 00167 if(ball_x < player_x || ball_x > player_x + p_width){ 00168 end = true; 00169 } else { 00170 ball_y = height - ball_r; 00171 ball_vy *= -1; 00172 } 00173 } 00174 00175 if(ball_y - ball_r < 0){ 00176 ball_y = ball_r; 00177 ball_vy *= -1; 00178 } 00179 00180 for(int i = 0; i < 32; i++ ){ 00181 if(wall[i]->exist){ 00182 if (!(ball_x > wall[i]->x + wall[i]->width || 00183 ball_x + ball_r < wall[i]->x || 00184 ball_y > wall[i]->y + wall[i]->height || 00185 ball_y + ball_r < wall[i]->y)){ 00186 wall[i]->exist = false; 00187 score++; 00188 } 00189 } 00190 } 00191 if(score == 32){ 00192 win = true; 00193 } 00194 wait_ms(REFRESH_TIME_MS); 00195 } 00196 lcd.cls(); 00197 00198 if(win){ 00199 lcd.text_string("You Win!!!", 20, 60, FONT_8X8, WHITE); 00200 } else { 00201 lcd.text_string("Game Over...", 20, 60, FONT_8X8, WHITE); 00202 } 00203 00204 }
Generated on Sun Jul 17 2022 00:10:11 by
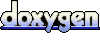