s
Fork of keypad by
Embed:
(wiki syntax)
Show/hide line numbers
Keypad.cpp
00001 #include "Keypad.h" 00002 #include "mbed.h" 00003 00004 extern Serial PC; 00005 00006 void Keypad::_cbRow0Rise() 00007 { 00008 _checkIndex(0, _rows[0]); 00009 } 00010 00011 void Keypad::_cbRow1Rise() 00012 { 00013 _checkIndex(1, _rows[1]); 00014 } 00015 00016 void Keypad::_cbRow2Rise() 00017 { 00018 _checkIndex(2, _rows[2]); 00019 } 00020 00021 void Keypad::_cbRow3Rise() 00022 { 00023 _checkIndex(3, _rows[3]); 00024 } 00025 00026 void 00027 Keypad::_setupRiseTrigger 00028 ( 00029 ) 00030 { 00031 if (_rows[0]) { 00032 _rows[0]->rise(this, &Keypad::_cbRow0Rise); 00033 } 00034 00035 if (_rows[1]) { 00036 _rows[1]->rise(this, &Keypad::_cbRow1Rise); 00037 } 00038 00039 if (_rows[2]) { 00040 _rows[2]->rise(this, &Keypad::_cbRow2Rise); 00041 } 00042 00043 if (_rows[3]) { 00044 _rows[3]->rise(this, &Keypad::_cbRow3Rise); 00045 } 00046 } 00047 00048 00049 Keypad::Keypad 00050 (PinName r0 00051 ,PinName r1 00052 ,PinName r2 00053 ,PinName r3 00054 ,PinName c0 00055 ,PinName c1 00056 ,PinName c2 00057 ,PinName c3 00058 ,int debounce_ms 00059 ) 00060 { 00061 PinName rPins[4] = {r0, r1, r2, r3}; 00062 PinName cPins[4] = {c0, c1, c2, c3}; 00063 00064 for (int i = 0; i < 4; i++) { 00065 _rows[i] = NULL; 00066 _cols[i] = NULL; 00067 } 00068 00069 _nRow = 0; 00070 for (int i = 0; i < 4; i++) { 00071 if (rPins[i] != NC) { 00072 _rows[i] = new InterruptIn(rPins[i]); 00073 _nRow++; 00074 } else 00075 break; 00076 } 00077 _setupRiseTrigger(); 00078 00079 _nCol = 0; 00080 for (int i = 0; i < 4; i++) { 00081 if (cPins[i] != NC) { 00082 _cols[i] = new DigitalOut(cPins[i]); 00083 _nCol++; 00084 } else 00085 break; 00086 } 00087 00088 _debounce = debounce_ms; 00089 } 00090 00091 Keypad::~Keypad 00092 () 00093 { 00094 for (int i = 0; i < 4; i++) { 00095 if (_rows[i] != 0) 00096 delete _rows[i]; 00097 } 00098 00099 for (int i = 0; i < 4; i++) { 00100 if (_cols[i] != 0) 00101 delete _cols[i]; 00102 } 00103 } 00104 00105 void 00106 Keypad::start 00107 ( 00108 ) 00109 { 00110 for (int i = 0; i < _nCol; i++) 00111 _cols[i]->write(1); 00112 } 00113 00114 void 00115 Keypad::stop 00116 ( 00117 ) 00118 { 00119 for (int i = 0; i < _nCol; i++) 00120 _cols[i++]->write(0); 00121 } 00122 00123 void 00124 Keypad::attach 00125 (uint32_t (*fptr)(uint32_t index) 00126 ) 00127 { 00128 _callback.attach(fptr); 00129 } 00130 00131 void 00132 Keypad::_checkIndex 00133 (int row 00134 ,InterruptIn *therow 00135 ) 00136 { 00137 #ifdef THREAD_H 00138 Thread::wait(_debounce); 00139 #else 00140 wait_ms(_debounce); 00141 #endif 00142 00143 if (therow->read() == 0) 00144 return; 00145 00146 int c; 00147 for (c = 0; c < _nCol; c++) { 00148 _cols[c]->write(0); // de-energize the column 00149 if (therow->read() == 0) { 00150 break; 00151 } 00152 } 00153 00154 int index = row * _nCol + c; 00155 _callback.call(index); 00156 start(); // Re-energize all columns 00157 } 00158
Generated on Tue Jul 12 2022 22:37:51 by
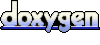