
Server that executes RPC commands through HTTP.
Dependencies: EthernetInterface mbed-rpc mbed-rtos mbed
Formatter.cpp
00001 #include "Formatter.h" 00002 #include "mbed.h" 00003 #include "RPCObjectManager.h" 00004 #include "EthernetInterface.h" 00005 00006 const char *SIMPLE_HTML_CODE = "\ 00007 <!DOCTYPE html>\ 00008 <html>\ 00009 <head>\ 00010 <meta http-equiv=\"Content-Type\" content=\"text/html; charset=utf-8\">\ 00011 <title>TCP Server</title>\ 00012 </head>\ 00013 <body>"; 00014 00015 00016 const char* INTERACTIVE_HTML_CODE_1 = "\ 00017 <!DOCTYPE html> \ 00018 <html>\ 00019 <head>\ 00020 <meta http-equiv=\"Content-Type\" content=\"text/html; charset=utf-8\">\ 00021 <title>TCP Server</title>\ 00022 <script type=\"text/javascript\">\ 00023 var ip = \"%s\";\ 00024 function submitCreateForm()\ 00025 {\ 00026 var list = document.getElementById(\"type\");\ 00027 var type = list.options[list.selectedIndex].value;\ 00028 var name = document.getElementById(\"name\").value;\ 00029 if(name === \"\") \ 00030 return;\ 00031 var arg = document.getElementById(\"arg\").value;\ 00032 var url;\ 00033 if(arg === \"\") url = \"http://\" + ip + type + \"new?name=\" + name;\ 00034 else url = \"http://\" + ip + type + \"new?arg=\" + arg + \"&name=\" + name;\ 00035 location.href= url;\ 00036 }\ 00037 function submitCallFuncForm()\ 00038 {\ 00039 var command = document.getElementById(\"command\").value;\ 00040 if(command === \"\") \ 00041 return; \ 00042 var tmp = command.split(\' \');\ 00043 var url = tmp[0];\ 00044 if(tmp.length > 1)\ 00045 url += \"?\";\ 00046 for(var i = 1; i < tmp.length; ++i)\ 00047 {\ 00048 url += \"arg\" + i + \"=\" + tmp[i];\ 00049 if(i+1 < tmp.length)\ 00050 url += \"&\";\ 00051 }\ 00052 location.href = url;\ 00053 }\ 00054 </script>\ 00055 </head> \ 00056 <body>"; 00057 00058 const char* INTERACTIVE_HTML_CODE_2 = "<h3>Create Object :</h3>\ 00059 <form>\ 00060 Type: <select id=\"type\">\ 00061 <option value=\"/DigitalOut/\">DigitalOut</option>\ 00062 <option value=\"/DigitalIn/\">DigitalIn</option>\ 00063 <option value=\"/DigitalInOut/\">DigitalInOut</option>\ 00064 <option value=\"/PwmOut/\">PwmOut</option>\ 00065 <option value=\"/Timer/\">Timer</option>\ 00066 </select><br>\ 00067 name: <input type=\"text\" id=\"name\"><br>\ 00068 arg(optional): <input type=\"text\" id=\"arg\">\ 00069 <p><input type=\"button\" value=\"Create\" onclick=\"javascript:submitCreateForm();\"></p>\ 00070 </form> \ 00071 \ 00072 <h3>Call a function :</h3>\ 00073 <p>Enter an RPC command.</p>\ 00074 <form>\ 00075 Command: <input type= \"text\" id=\"command\" maxlength=127><br>\ 00076 <p><input type=\"button\" value=\"Send\" onclick=\"javascript:submitCallFuncForm();\"></p><br>\ 00077 </form>\ 00078 </body> \ 00079 </html>"; 00080 00081 static char chunk[1024]; 00082 00083 Formatter::Formatter(int nb): 00084 currentChunk(0), 00085 nbChunk(nb) 00086 { 00087 } 00088 00089 char* Formatter::get_page(char *reply) 00090 { 00091 chunk[0] = '\0'; 00092 00093 if(currentChunk < nbChunk) 00094 { 00095 get_chunk(currentChunk, reply); 00096 currentChunk++; 00097 } 00098 else 00099 currentChunk = 0; 00100 00101 return chunk; 00102 } 00103 00104 void Formatter::get_chunk(const int c, char *reply) 00105 { 00106 strcat(chunk, reply); 00107 } 00108 00109 SimpleHTMLFormatter::SimpleHTMLFormatter(): 00110 Formatter() 00111 { 00112 } 00113 00114 void SimpleHTMLFormatter::get_chunk(const int c, char* reply) 00115 { 00116 strcat(chunk, SIMPLE_HTML_CODE); 00117 00118 if(reply != NULL && strlen(reply) != 0) 00119 { 00120 strcat(chunk, "RPC reply : "); 00121 strcat(chunk, reply); 00122 } 00123 00124 if(!RPCObjectManager::instance().is_empty()) 00125 { 00126 strcat(chunk, "<ul>"); 00127 for(std::list<char*>::iterator itor = RPCObjectManager::instance().begin(); 00128 itor != RPCObjectManager::instance().end(); 00129 ++itor) 00130 { 00131 strcat(chunk, "<li>"); 00132 strcat(chunk, *itor); 00133 strcat(chunk, "</li>"); 00134 } 00135 strcat(chunk, "</ul>"); 00136 } 00137 00138 strcat(chunk, "</body></html>"); 00139 } 00140 00141 InteractiveHTMLFormatter::InteractiveHTMLFormatter(): 00142 Formatter(3) 00143 { 00144 } 00145 00146 void InteractiveHTMLFormatter::get_chunk(const int c, char *reply) 00147 { 00148 if(c == 0) 00149 sprintf(chunk, INTERACTIVE_HTML_CODE_1, EthernetInterface::getIPAddress()); 00150 00151 else if(c == 1) 00152 { 00153 if(reply != NULL && strlen(reply) != 0) 00154 { 00155 strcat(chunk, "RPC reply : "); 00156 strcat(chunk, reply); 00157 } 00158 if(!RPCObjectManager::instance().is_empty()) 00159 { 00160 strcat(chunk, "<p>Objects created :</p>"); 00161 00162 strcat(chunk, "<ul>"); 00163 for(std::list<char*>::iterator itor = RPCObjectManager::instance().begin(); 00164 itor != RPCObjectManager::instance().end(); 00165 ++itor) 00166 { 00167 strcat(chunk, "<li>"); 00168 strcat(chunk, *itor); 00169 strcat(chunk, " (<a href=\"http://"); 00170 strcat(chunk, EthernetInterface::getIPAddress()); 00171 strcat(chunk, "/"); 00172 strcat(chunk, *itor); 00173 strcat(chunk, "/delete\">delete</a>)"); 00174 strcat(chunk, "</li>"); 00175 } 00176 strcat(chunk, "</ul>"); 00177 } 00178 strcat(chunk, " "); 00179 } 00180 else if(c == 2) 00181 strcat(chunk, INTERACTIVE_HTML_CODE_2); 00182 } 00183 00184
Generated on Tue Jul 12 2022 18:58:44 by
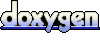