This library implements some hash and cryptographic algorithms.
Dependents: mBuinoBlinky PB_Emma_Ethernet SLOTrashHTTP Garagem ... more
SHA2_32.h
00001 #ifndef SHA2_32_H 00002 #define SHA2_32_H 00003 00004 #include <stdint.h> 00005 00006 enum SHA_32_TYPE 00007 { 00008 SHA_224, 00009 SHA_256 00010 }; 00011 00012 class SHA2_32 00013 { 00014 public : 00015 00016 SHA2_32(SHA_32_TYPE type); 00017 void update(uint8_t *data, uint32_t length); 00018 void finalize(uint8_t *digest); 00019 00020 static void computeHash(SHA_32_TYPE type, uint8_t *digest, uint8_t *data, uint32_t length); 00021 00022 private : 00023 00024 static void computeBlock(uint32_t *h02, 00025 uint32_t *h12, 00026 uint32_t *h22, 00027 uint32_t *h32, 00028 uint32_t *h42, 00029 uint32_t *h52, 00030 uint32_t *h62, 00031 uint32_t *h72, 00032 uint8_t *buffer); 00033 00034 SHA_32_TYPE type; 00035 uint32_t h0, h1, h2, h3, h4, h5, h6, h7; 00036 uint32_t totalBufferLength; 00037 uint8_t buffer[64]; 00038 uint8_t bufferLength; 00039 }; 00040 00041 #endif
Generated on Tue Jul 12 2022 18:54:16 by
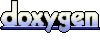