This library implements some hash and cryptographic algorithms.
Dependents: mBuinoBlinky PB_Emma_Ethernet SLOTrashHTTP Garagem ... more
BlockCipher.h
00001 #ifndef BLOCK_CIPHER_H 00002 #define BLOCK_CIPHER_H 00003 00004 #include "Cipher.h" 00005 00006 enum BLOCK_CIPHER_MODE 00007 { 00008 ECB_MODE, 00009 CBC_MODE 00010 }; 00011 00012 class BlockCipher : public Cipher 00013 { 00014 public : 00015 00016 BlockCipher(uint32_t bs, BLOCK_CIPHER_MODE m, uint8_t *iv = 0); 00017 virtual ~BlockCipher(); 00018 00019 virtual CIPHER_TYPE getType() const; 00020 uint32_t getBlockSize() const; 00021 00022 virtual void encrypt(uint8_t *out, uint8_t *in, uint32_t length); 00023 virtual void decrypt(uint8_t *out, uint8_t *in, uint32_t length); 00024 00025 protected : 00026 00027 virtual void encryptBlock(uint8_t *out, uint8_t *in) = 0; 00028 virtual void decryptBlock(uint8_t *out, uint8_t *in) = 0; 00029 00030 private : 00031 00032 uint32_t blockSize; 00033 BLOCK_CIPHER_MODE mode; 00034 uint8_t *IV; 00035 }; 00036 00037 #endif
Generated on Tue Jul 12 2022 18:54:16 by
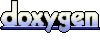