This library implements some hash and cryptographic algorithms.
Dependents: mBuinoBlinky PB_Emma_Ethernet SLOTrashHTTP Garagem ... more
BlockCipher.cpp
00001 #include "BlockCipher.h" 00002 #include <string.h> 00003 00004 BlockCipher::BlockCipher(uint32_t bs, BLOCK_CIPHER_MODE m, uint8_t *iv): 00005 Cipher(), 00006 blockSize(bs), 00007 mode(m), 00008 IV(0) 00009 { 00010 if(mode == CBC_MODE) 00011 { 00012 IV = new uint8_t[blockSize]; 00013 memcpy(IV, iv, blockSize); 00014 } 00015 } 00016 00017 BlockCipher::~BlockCipher() 00018 { 00019 if(IV != 0) 00020 delete[] IV; 00021 } 00022 00023 CIPHER_TYPE BlockCipher::getType() const 00024 { 00025 return BLOCK_CIPHER; 00026 } 00027 00028 uint32_t BlockCipher::getBlockSize() const 00029 { 00030 return blockSize; 00031 } 00032 00033 void BlockCipher::encrypt(uint8_t *out, uint8_t *in, uint32_t length) 00034 { 00035 uint8_t *tmp = 0; 00036 if(mode == CBC_MODE) 00037 tmp = new uint8_t[getBlockSize()]; 00038 for(uint32_t i = 0; i < length; i += getBlockSize()) 00039 { 00040 if(mode == CBC_MODE) 00041 { 00042 memcpy(tmp, &in[i], getBlockSize()); 00043 for(int j = 0; j < (int)getBlockSize(); ++j) 00044 tmp[j] ^= IV[j]; 00045 00046 encryptBlock(&out[i], tmp); 00047 00048 memcpy(IV, &out[i], getBlockSize()); 00049 } 00050 else 00051 encryptBlock(&out[i], &in[i]); 00052 } 00053 if(mode == CBC_MODE) 00054 delete[] tmp; 00055 } 00056 00057 void BlockCipher::decrypt(uint8_t *out, uint8_t *in, uint32_t length) 00058 { 00059 for(uint32_t i = 0; i < length; i += getBlockSize()) 00060 { 00061 if(mode == CBC_MODE) 00062 { 00063 decryptBlock(&out[i], &in[i]); 00064 for(int j = 0; j < (int)getBlockSize(); ++j) 00065 out[i+j] ^= IV[j]; 00066 00067 memcpy(IV, &in[i], getBlockSize()); 00068 } 00069 else 00070 decryptBlock(&out[i], &in[i]); 00071 } 00072 }
Generated on Tue Jul 12 2022 18:54:16 by
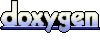