
test MAX31850
Embed:
(wiki syntax)
Show/hide line numbers
sht7X.cpp
00001 // 00002 // Ian Molesworth October 2010 00003 // SHT 75 class 00004 // 00005 // 00006 // To do: 00007 // modification PULL-UP 00008 //2010_12_22 F.BLANC 00009 00010 #include "sht7X.h" 00011 00012 void SHT75::reset() { 00013 _data.output(); 00014 _data = 1; // data bus high 00015 for (int i=0;i<12;i++) { 00016 _clock = 1; // clock high 00017 wait_us(1); 00018 _clock = 0; // clock lo 00019 wait_us(1); 00020 } 00021 _clock = 1; // clock high 00022 wait_us(1); 00023 _data = 0; 00024 wait_us(1); 00025 _clock = 0; // clock lo 00026 wait_us(1); 00027 _clock = 1; 00028 wait_us(1); 00029 _data = 1; 00030 wait_us(1); 00031 _clock = 0; 00032 wait_us(1); 00033 } 00034 00035 void SHT75::softReset(void) { 00036 _data.output(); 00037 start(); 00038 write(0x1E); 00039 wait_ms(12); 00040 } 00041 00042 void SHT75::start(void) { 00043 _data.output(); 00044 _clock = 1; 00045 wait_us(1); 00046 _data = 0; 00047 wait_us(1); 00048 _clock = 0; 00049 wait_us(1); 00050 _clock = 1; 00051 wait_us(1); 00052 _data = 1; 00053 wait_us(1); 00054 _clock = 0; 00055 } 00056 00057 int SHT75::readStatus(void) { 00058 int status; 00059 status = -1; 00060 start(); 00061 if (write(0x06) == 0) 00062 status = read(1); // read with a wait for ack 00063 read(0); // read without the wait 00064 return status; 00065 } 00066 00067 bool SHT75::write(char d) { 00068 auto int i; 00069 _data.output(); // bus output 00070 // Writes char and returns -1 if no ACK was sent from remote 00071 for (i=0;i<8;i++) { 00072 if (d & 0x80) 00073 _data = 1; // data high 00074 else 00075 _data = 0; // data lo 00076 // shift the data 00077 d <<= 1; 00078 wait_us(1); 00079 _clock = 1; // clock high 00080 wait_us(1); 00081 _clock = 0; // clock lo 00082 } 00083 _data.input(); // float the bus 00084 _data.mode(PullUp); //PULL-UP 00085 wait_us(1); 00086 _clock = 1; // clock high 00087 wait_us(1); 00088 i = _data; 00089 _clock = 0; // clock lo 00090 return i; // leave the bus in input mode and return the status of the ack bit read. 00091 } 00092 00093 int SHT75::read(char ack) { 00094 auto int i,s; 00095 auto char c; 00096 s = 0; 00097 _data.input(); // bus to input 00098 _data.mode(PullUp); //PULL-UP 00099 for (i=0;i<8;i++) { 00100 s <<= 1; 00101 wait_us(1); 00102 _clock = 1; // clock high 00103 wait_us(1); 00104 c = _data; // get the data bit 00105 _clock = 0; // clock lo 00106 if ( c ) 00107 s |= 1; 00108 } 00109 00110 if (ack == 1) 00111 _data = 0; // data lo 00112 else 00113 _data = 1; // data hi 00114 _data.output(); 00115 _clock = 1; // clock lo 00116 wait_us(1); 00117 _clock = 0; // clock lo 00118 _data = 1; // data hi 00119 _data.input(); 00120 _data.mode(PullUp); //PULL-UP 00121 return s; 00122 } 00123 00124 00125 // Put the current temperature into passed variable 00126 bool SHT75::readTempTicks(int* temp) { 00127 int v, value; 00128 start(); // Start a tx ( leaves data as input ) 00129 if (write(0x03) == 0) { // send the read command and get an ack 00130 for (v=0; v<50; v ++) { // wait for ready up to 500 ms 00131 wait_ms(10); // 10 ms pause 00132 if ( _data == 0 ) { // 00133 value = read(1); // read a byte 00134 value <<= 8; // shift it in 00135 value |= read(1); // read another byte 00136 read(0); 00137 // transfer the value 00138 *temp = value; 00139 reset(); 00140 return true; 00141 } 00142 } 00143 } 00144 return false; 00145 } 00146 00147 bool SHT75::readHumidityTicks(int* humi) { 00148 start(); 00149 if (write(0x05) == 0) { 00150 for (int value=0; value<50; value ++) { // wait for ready up to 500 ms 00151 wait_ms(10); // 00152 if ( _data == 0 ) { // 00153 value = read(1); 00154 value <<= 8; 00155 value |= read(1); 00156 read(0); 00157 *humi = value; // transfer the value 00158 reset(); 00159 return true; 00160 } 00161 } 00162 } 00163 00164 return false; 00165 }
Generated on Tue Jul 12 2022 16:41:44 by
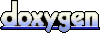