
runinig version
Dependencies: C12832_lcd NetServices freezer_guard_running mbed
Fork of Freezer_Guard_prog by
main.cpp
00001 /****************************************************** 00002 *File Name: Freezer_Guard main.cpp 00003 *Purpose: Freezer Guard System protects your freezer from unintentionaly defrost 00004 * 00005 *Author: denis schnier and fabian bitz @ University of applied sciences bingen 00006 *Changes: 00007 * 00008 * 18.05.2015 initial version 00009 * ... 00010 *******************************************************/ 00011 00012 #include "guard.h" 00013 #include "string.h" 00014 #define LIMIT 25 // Temperature Alarm Threshold 00015 byte MAIN_bState; 00016 byte bError; 00017 char cString[25]; 00018 char data_array[2]; 00019 DigitalOut led4(LED4); //DEBUG 00020 00021 int main() 00022 { 00023 int8 i8Value=0; //value has 8 bit + sign 00024 MAIN_bState=0; //statemachine: 0=no Alarm, 1=Alarm and no Mail sent, 2=Alarm and Mail sent, 3= Pending Alarm Confirmed 00025 bError=0; 00026 vGuardInit(); 00027 led4=0; //DEBUG 00028 while(1){ 00029 i8Value=readValue(MCP9808_ADDR); 00030 wait(1); 00031 if(i8Value>LIMIT && MAIN_bState==0) MAIN_bState=1; 00032 if(i8Value<LIMIT) MAIN_bState=0; 00033 switch(MAIN_bState) { 00034 case 0: { //normal case 00035 vLcdIntPrint(i8Value); 00036 vLcdAlarmPrint("System Running"); 00037 vLcdStringPrint("Temperature Ok \n "); 00038 break; 00039 } 00040 00041 case 1:{ 00042 vLcdIntPrint(i8Value); 00043 vLcdAlarmPrint("Alarm! "); 00044 vAudioAlarm(); 00045 // bError=SendMail(i8Value); 00046 if(SendMail(i8Value))vLcdStringPrint("Mail Sent! \n "); 00047 else{ 00048 vLcdStringPrint("Mail Not Sent! \n "); 00049 } 00050 led4=1; //DEBUG 00051 00052 MAIN_bState=2; 00053 break; 00054 } 00055 case 2:{ // case: mail sent, alarm pending 00056 vLcdIntPrint(i8Value); 00057 vAudioAlarm(); 00058 break; 00059 } 00060 case 3:{ //MAIN_bState=3 could only be set by ISR 00061 vLcdIntPrint(i8Value); 00062 break; 00063 } 00064 default:{ 00065 vLcdStringPrint("State Machine Error!"); 00066 break; 00067 } 00068 }//state machine 00069 }//while(1) 00070 00071 }//main
Generated on Fri Jul 15 2022 15:32:44 by
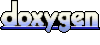