
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
stepper.h
00001 /* 00002 stepper.h - stepper motor driver: executes motion plans of planner.c using the stepper motors 00003 Part of Grbl 00004 00005 Copyright (c) 2009-2011 Simen Svale Skogsrud 00006 00007 Grbl is free software: you can redistribute it and/or modify 00008 it under the terms of the GNU General Public License as published by 00009 the Free Software Foundation, either version 3 of the License, or 00010 (at your option) any later version. 00011 00012 Grbl is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 GNU General Public License for more details. 00016 00017 You should have received a copy of the GNU General Public License 00018 along with Grbl. If not, see <http://www.gnu.org/licenses/>. 00019 */ 00020 00021 #ifndef stepper_h 00022 #define stepper_h 00023 00024 // from nuts_bolts.h: 00025 #define square(x) ((x)*(x)) 00026 #define sleep_mode(x) do {} while (0) 00027 // #define sei(x) 00028 00029 #define NUM_AXES 4 00030 00031 #define X_AXIS 0 00032 #define Y_AXIS 1 00033 #define Z_AXIS 2 00034 #define E_AXIS 3 00035 00036 #define clear_vector(a) memset(a, 0, sizeof(a)) 00037 #define clear_vector_double(a) memset(a, 0.0, sizeof(a)) 00038 #define max(a,b) (((a) > (b)) ? (a) : (b)) 00039 #define min(a,b) (((a) < (b)) ? (a) : (b)) 00040 // end 00041 00042 /* From grbl/config.h */ 00043 #define X_STEP_BIT 20 00044 #define Y_STEP_BIT 25 00045 #define Z_STEP_BIT 29 00046 #define E_STEP_BIT 10 00047 00048 #define X_DIRECTION_BIT 23 00049 #define Y_DIRECTION_BIT 26 00050 #define Z_DIRECTION_BIT 0 00051 #define E_DIRECTION_BIT 11 00052 00053 00054 // This parameter sets the delay time before disabling the steppers after the final block of movement. 00055 // A short delay ensures the steppers come to a complete stop and the residual inertial force in the 00056 // CNC axes don't cause the axes to drift off position. This is particularly important when manually 00057 // entering g-code into grbl, i.e. locating part zero or simple manual machining. If the axes drift, 00058 // grbl has no way to know this has happened, since stepper motors are open-loop control. Depending 00059 // on the machine, this parameter may need to be larger or smaller than the default time. 00060 #define STEPPER_IDLE_LOCK_TIME 25 // (milliseconds) 00061 00062 // The temporal resolution of the acceleration management subsystem. Higher number give smoother 00063 // acceleration but may impact performance. 00064 // NOTE: Increasing this parameter will help any resolution related issues, especially with machines 00065 // requiring very high accelerations and/or very fast feedrates. In general, this will reduce the 00066 // error between how the planner plans the motions and how the stepper program actually performs them. 00067 // However, at some point, the resolution can be high enough, where the errors related to numerical 00068 // round-off can be great enough to cause problems and/or it's too fast for the Arduino. The correct 00069 // value for this parameter is machine dependent, so it's advised to set this only as high as needed. 00070 // Approximate successful values can range from 30L to 100L or more. 00071 #define ACCELERATION_TICKS_PER_SECOND 1000L 00072 00073 // Minimum planner junction speed. Sets the default minimum speed the planner plans for at the end 00074 // of the buffer and all stops. This should not be much greater than zero and should only be changed 00075 // if unwanted behavior is observed on a user's machine when running at very slow speeds. 00076 #define MINIMUM_PLANNER_SPEED 0.0 // (mm/min) 00077 00078 // Minimum stepper rate. Sets the absolute minimum stepper rate in the stepper program and never run 00079 // slower than this value, except when sleeping. This parameter overrides the minimum planner speed. 00080 // This is primarily used to guarantee that the end of a movement is always reached and not stop to 00081 // never reach its target. This parameter should always be greater than zero. 00082 #define MINIMUM_STEPS_PER_MINUTE 1200 // (steps/min) - Integer value only 00083 00084 // Number of arc generation iterations by small angle approximation before exact arc 00085 // trajectory correction. Value must be 1-255. This parameter maybe decreased if there are issues 00086 // with the accuracy of the arc generations. In general, the default value is more than enough for 00087 // the intended CNC applications of grbl, and should be on the order or greater than the size of 00088 // the buffer to help with the computational efficiency of generating arcs. 00089 #define N_ARC_CORRECTION 25 00090 00091 // end 00092 00093 #define LIMIT_MASK ((1<<X_LIMIT_BIT)|(1<<Y_LIMIT_BIT)|(1<<Z_LIMIT_BIT)) // All limit bits 00094 #define STEP_MASK ((1<<X_STEP_BIT)|(1<<Y_STEP_BIT)|(1<<Z_STEP_BIT)) // All step bits 00095 #define DIRECTION_MASK ((1<<X_DIRECTION_BIT)|(1<<Y_DIRECTION_BIT)|(1<<Z_DIRECTION_BIT)) // All direction bits 00096 #define STEPPING_MASK (STEP_MASK | DIRECTION_MASK) // All stepping-related bits (step/direction) 00097 00098 // Initialize and start the stepper motor subsystem 00099 void st_init(); 00100 00101 // Block until all buffered steps are executed 00102 void st_synchronize(); 00103 00104 // Execute the homing cycle 00105 void st_go_home(); 00106 00107 // The stepper subsystem goes to sleep when it runs out of things to execute. Call this 00108 // to notify the subsystem that it is time to go to work. 00109 void st_wake_up(); 00110 00111 #endif
Generated on Tue Jul 12 2022 21:03:04 by
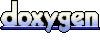